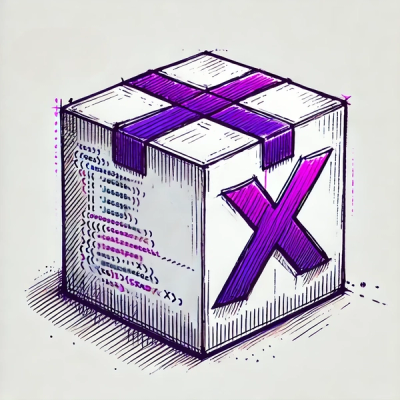
Security News
pnpm 10.0.0 Blocks Lifecycle Scripts by Default
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
youzanyun-data-security
Advanced tools
npm install youzanyun-data-security --save
const DataSecurity = require('youzanyun-data-security');
(async () => {
const clientId = '68ffaf8440a4babcde';
const clientSecret = 'b0030740948e368c4ca21ed5d0b123456';
const kdtId = 789456;
const ds = new DataSecurity(clientId, clientSecret);
// 加密
const cipherText = await ds.encrypt(kdtId, '13012341234');
const cipherText2 = await ds.encrypt(kdtId, '18812341234');
// 解密
const source = await ds.decrypt(kdtId, cipherText); // 13012341234
// 解密并脱敏
const decryptMaskResult = await ds.decryptMask(kdtId, cipherText, DataSecurity.MASK_TYPE.MOBILE); // 130****1234
// 批量加密
const encryptResult = await ds.batchEncrypt(kdtId, ['13012341234', '浙江省杭州市']); // {"13012341234": 'xxxxx', "浙江省杭州市": xxxxx}
// 批量解密
const mobiles = batchDecrypt(kdtId, [cipherText, cipherText2]);
// 批量解密并脱敏
const result = await ds.batchDecryptMask(
kdtId,
[cipherText, cipherText2],
SecretClient.MASK_TYPE.BANK_CARD,
);
// 生成模糊搜索字符串
const searchStr = await ds.generateEncryptSearchDigest(kdtId, cipherText);
// 是否是密文
const isCipher = DataSecurity.isEncrypt(cipherText); // true
// 批量判断是否是密文
const isCiphers = DataSecurity.isEncrypt([cipherText, cipherText2]); // true
})();
构造器函数
字段 | 类型 | 说明 |
---|---|---|
clientId | string | clientId |
clientSecret | string | clientSecret |
const ds = new DataSecurity(clientId, clientSecret);
加密方法,返回加密后的字符串
字段 | 类型 | 说明 |
---|---|---|
kdtId | number | 店铺 id |
source | string | 明文 |
const cipherText = await ds.encrypt(kdtId, '13012341234');
const cipherText2 = await ds.encrypt(kdtId, '18812341234');
批量加密方法,返回一个 Object,key 为明文,value 为密文。
字段 | 类型 | 说明 |
---|---|---|
kdtId | number | 店铺 id |
sources | string[] | 明文数组 |
const encryptResult = await ds.batchEncrypt(kdtId, ['13012341234', '浙江省杭州市']); // {"13012341234": 'xxxxx', "浙江省杭州市": xxxxx}
解密方法,返回解密后的明文,传入明文时返回明文。tip: 解密失败会抛异常
字段 | 类型 | 说明 |
---|---|---|
kdtId | number | 店铺 id |
cipherText | string | 密文 |
// 加密
const cipherText = await ds.encrypt(kdtId, '13012341234');
// 解密
const source = await ds.decrypt(kdtId, cipherText); // 13012341234
批量解密,返回一个 Object,key 为密文,value 为明文。
字段 | 类型 | 说明 |
---|---|---|
kdtId | number | 店铺 id |
cipherTexts | string[] | 密文数组 |
// 加密
const cipherText = await ds.encrypt(kdtId, '13012341234');
const cipherText2 = await ds.encrypt(kdtId, '18812341234');
// 批量解密
const mobiles = batchDecrypt(kdtId, [cipherText, cipherText2]);
解密并脱敏,返回解密并脱敏后的明文。
字段 | 类型 | 说明 |
---|---|---|
kdtId | number | 店铺 id |
cipherText | string | 密文 |
type | number | 脱敏类型 (参考文档最后的脱敏类型枚举) |
// 加密
const cipherText = await ds.encrypt(kdtId, '13012341234');
// 解密并脱敏
const decryptMaskResult = await ds.decryptMask(kdtId, cipherText, DataSecurity.MASK_TYPE.MOBILE); // 130****1234
批量解密并脱敏,返回一个 Object,key 为密文,value 为解密并脱敏后的明文。
字段 | 类型 | 说明 |
---|---|---|
kdtId | number | 店铺 id |
cipherTexts | string[] | 密文数组 |
type | number | 脱敏类型 (参考文档最后的脱敏类型枚举) |
// 加密
const cipherText = await ds.encrypt(kdtId, '13012341234');
const cipherText2 = await ds.encrypt(kdtId, '18812341234');
// 批量解密并脱敏
const result = await ds.batchDecryptMask(
kdtId,
[cipherText, cipherText2],
SecretClient.MASK_TYPE.BANK_CARD,
);
生成模糊加密字符串, 支持部分搜索,返回一个结果字符串。
字段 | 类型 | 说明 |
---|---|---|
kdtId | number | 店铺 id |
source | string | 明文 |
// 加密
const cipherText = await ds.encrypt(kdtId, '13012341234');
// 生成模糊搜索字符串
const searchStr = await ds.generateEncryptSearchDigest(kdtId, cipherText);
静态属性,脱敏类型枚举定义
类型 | 类型说明 | 值 |
---|---|---|
ADDRESS | 地址 | 0 |
BANK_CARD | 银行卡号 | 1 |
NAME | 用户名 | 2 |
邮箱地址 | 3 | |
COMPANY_NAME | 企业名称 | 4 |
ID_CARD | 身份证号 | 5 |
MOBILE | 手机号码 | 6 |
// 加密
const cipherText = await ds.encrypt(kdtId, '13012341234');
// 解密并脱敏
const decryptMaskResult = await ds.decryptMask(kdtId, cipherText, DataSecurity.MASK_TYPE.MOBILE); // 130****1234
判断输入是不是密文,返回 true/false。
const cipherText = await ds.encrypt(kdtId, '13012341234');
const isCipher = DataSecurity.isEncrypt(cipherText); // true
批量是否密文判断,返回 true/false。
// 加密
const cipherText = await ds.encrypt(kdtId, '13012341234');
const cipherText2 = await ds.encrypt(kdtId, '18812341234');
const isCipher = DataSecurity.isEncrypt([cipherText, cipherText2]);
FAQs
有赞云数据加密 NodeJS 版
We found that youzanyun-data-security demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.