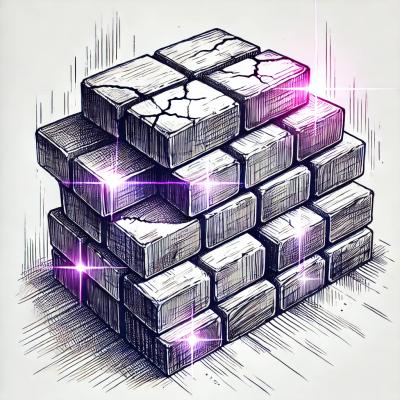
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
youzanyun-oss-sdk
Advanced tools
有赞 OSS 下的 Node 客户端,提供 put、putStream、get、getStream、head、delete 方法
yarn add @youzan/youzan-oss -S
在 3.x 版本中,导出方式已订正为 ESM 标准导出,使用 CommonJS 的同学注意
上传文件到 OSS
CJS
const OSS = require('@youzan/youzan-oss').default;
// 实例化
const client = new OSS({
namespace: 'xxxx', // 需要申请
env: 'prod', // 可选。环境
});
// 上传文件,xxx 可以是文件路径、Buffer 或者是可读流
// key 是用来查找改资源文件的钥匙
client.put('key', 'xxxxx')
.then(url => {
console.log('put done. The resource url is %s', url);
})
.catch((err) => {
console.log(err);
})
ESM
import OSS from '@youzan/youzan-oss'
const client = new OSS({
namespace: 'xxxx',
env: 'prod'
})
const url = await client.put('key', 'xxxxx')
从 OSS 上获取文件
const OSS = require('@youzan/youzan-oss').default;
// 实例化
const client = new OSS({
namespace: 'xxxx' // 需要申请
});
// 查找文件
// key 是上传时定义的
// xxxx 可传,文件地址,传入后把 OSS 上的文件写入到此
client.get('key', 'xxxxx')
.then(() => {
console.log('get done');
})
.catch((err) => {
console.log(err);
})
目前实例化时值需要传入 namespace
即可,namespace
需要找运维申请
const client = new OSS({
namespace: 'xxxx' // 需要申请
});
参数 | 类型 | 描述 | 是否必传 | 默认值 | 备注 |
---|---|---|---|---|---|
namespace | string | 命名空间 | 是 | 无 | 需要申请 |
env | string | 环境 | 否 | NODE_ENV | |
host | string | 请求的 host 域名 | 否 | src/constants/index | 不传时会根据环境获取 |
put 方法用来将本地文件上传到 OSS
client.put('object-key', file)
.then(url => {
console.log('put done. The resource url is %s', url);
})
.catch((err) => {
console.log(err);
})
参数 | 类型 | 描述 | 是否必传 | 默认值 | 备注 |
---|---|---|---|---|---|
key | string | 文件对应的 key | 是 | 无 | 每个上传的文件对应的 key |
file | Buffer、ReadStream、string | 需要上传的文件 | 是 | 无 | 可以是文件路径、Buffer、可读流 |
options | urllib.RequestOptions | 请求参数 | 否 | 无 | 详见:https://www.npmjs.com/package/urllib |
putStream 方法用来将本地大文件上传到 OSS,可监听进度
(适合大文件)
const stream = client.putStream('object-key', file);
let total = 0;
let count = 0;
stream.on('progress', (contentLength, totalLength) => {
total += contentLength;
count += 1;
console.log(total + '------' + count);
});
stream.on('finish', url => {
console.log('put done. The resource url is %s', url);
console.log('finish', total + '------' + count)
});
stream.on('error', (err) => console.log(err));
参数 | 类型 | 描述 | 是否必传 | 默认值 | 备注 |
---|---|---|---|---|---|
key | string | 文件对应的 key | 是 | 无 | 每个上传的文件对应的 key |
file | ReadStream、string | 需要上传的文件 | 是 | 无 | 可以是文件路径、可读流 |
options | urllib.RequestOptions | 请求参数 | 否 | 无 | 详见:https://www.npmjs.com/package/urllib |
get 方法用来获取 OSS 上的文件
client.get('object-key', file)
.then(() => {
console.log('done');
})
.catch((err) => {
console.log(err);
})
client.get('object-key')
.then((buf) => {
// 返回 Buffer
console.log(buf');
})
.catch((err) => {
console.log(err);
})
参数 | 类型 | 描述 | 是否必传 | 默认值 | 备注 |
---|---|---|---|---|---|
key | string | 文件对应的 key | 是 | 无 | 每个上传的文件对应的 key |
file | string | 需要写入的文件路径 | 否 | 无 | 如果传入,将 OSS 上的内容写入到该文件 |
options | urllib.RequestOptions | 请求参数 | 否 | 无 | 详见:https://www.npmjs.com/package/urllib |
getStream 通过流的形式获取 OSS 文件,可获取下载进度。常用来获取大文件
const stream = client.getStream('object-key', file);
let total = 0;
stream.on('progress', (contentLength, totalLength) => {
total += contentLength;
console.log('获取进度为:' + total / totalLength);
});
stream.on('finish', () => console.log('获取完毕'));
stream.on('error', (err) => console.log('获取出错' + err));
getStream
入参和上面 get
相同。返回的实例可监听 on、finish、error
。
参数 | 类型 | 描述 | 是否必传 | 默认值 | 备注 |
---|---|---|---|---|---|
key | string | 文件对应的 key | 是 | 无 | 每个上传的文件对应的 key |
file | string、WriteStream | 需要写入的文件 | 否 | 无 | 如果传入,将 OSS 上的内容写入到该文件或可写流 |
options | urllib.RequestOptions | 请求参数 | 否 | 无 | 详见:https://www.npmjs.com/package/urllib |
head 方法用来获取 OSS 上的文件信息
client.head('object-key')
.then((info) => {
console.log(info);
})
.catch((err) => {
console.log(err);
})
参数 | 类型 | 描述 | 是否必传 | 默认值 | 备注 |
---|---|---|---|---|---|
key | string | 文件对应的 key | 是 | 无 | 每个上传的文件对应的 key |
delete 会删除 OSS 上的文件
client.delete('object-key')
.then(() => {
console.log('delete done');
})
.catch((err) => {
console.log(err);
})
参数 | 类型 | 描述 | 是否必传 | 默认值 | 备注 |
---|---|---|---|---|---|
key | string | 文件对应的 key | 是 | 无 | 每个上传的文件对应的 key |
code | message | 备注 |
---|---|---|
FILE_OUT_OF_SIZE | 文件超过限制 | 默认限制 1 G |
UNKNOWN_HOST | 当前 host 不是标准的环境变量 | host 必须是 qa pre prod test officeProd 中的一个 |
FAQs
有赞 node 端的 oss
We found that youzanyun-oss-sdk demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.