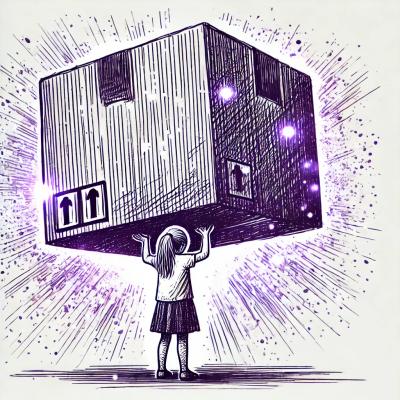
Security News
The Unpaid Backbone of Open Source: Solo Maintainers Face Increasing Security Demands
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.
Toolbox for Node. Contains stuff you only wished was there in the first place.
npm install yow --save
var sprintf = require('yow/sprintf');
var extend = require('yow/extend');
var mkdir = require('yow/fs').mkdir;
var mkpath = require('yow/fs').mkpath;
var fileExists = require('yow/fs').fileExists;
var readJSON = require('yow/fs').readJSON;
var writeJSON = require('yow/fs').writeJSON;
var isType = require('yow/is').isType;
var isArray = require('yow/is').isArray;
var isNumber = require('yow/is').isNumber;
var isString = require('yow/is').isString;
var isDate = require('yow/is').isDate;
var isFunction = require('yow/is').isFunction;
var isObject = require('yow/is').isObject;
var isInteger = require('yow/is').isInteger;
var isFloat = require('yow/is').isFloat;
var random = require('yow/random');
Also available as require('yow/random')
var range = require('yow/range');
var logs = require('yow/logs');
var Queue = require('yow/queue');
var queue = new Queue();
var Timer = require('yow/timer');
var timer = new Timer();
timer.setTimer(delay, fn) - Executes the specified function fn after a delay. Previously set timers are cancelled.
timer.cancel() - Cancels the timer.
FAQs
You Only Wish module
The npm package yow receives a total of 44 weekly downloads. As such, yow popularity was classified as not popular.
We found that yow demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.
Security News
License exceptions modify the terms of open source licenses, impacting how software can be used, modified, and distributed. Developers should be aware of the legal implications of these exceptions.
Security News
A developer is accusing Tencent of violating the GPL by modifying a Python utility and changing its license to BSD, highlighting the importance of copyleft compliance.