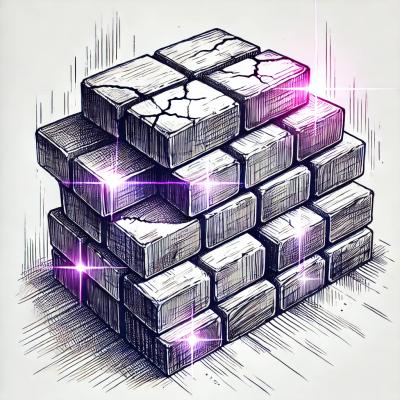
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Installing is as simple as using
npm i yt-api
To use the api you need a api key from google and you can get that here
Once you have created the api key you need to enable the Youtube Data API v3
by clicking on the libraries tab and clicking on the Youtube Data API v3
then click on enable and your done!
const api = require("yt-api");
const API = new api("PASTE_API_KEY_HERE");
API.getVideoInfo("YOUTUBE_VIDEO_ID" /*Needs to be a valid video id or it will throw a error!*/, info => {
console.log("This video's title is "+info.title+" with a id of "+info.videoID+".");
});
//Results for the getVideoInfo
videoID: //the video's id,
url: //the url to the video,
title: //The video title
channel: {
id: //The channel id
user: //The user,
url: //The video's channel url
},
livestream: //Is it a livestream?,
category: //The categoryId that the videos in,
thumbnail: //The videos thumbnail,
description: //The video's description,
publishDate: //The date it was published at,
duration: {
days: //The durration in days
hours: //The durration in hours,
minutes: //The durration in minutes,
seconds: //The durration in seconds
},
statistics: {
views: //The total views the video has,
likes: //The total likes the video has,
dislikes: //The total dislikes the video has,
comments: //The total commends the video has,
favorites: //The people who favorited the video
}
API.searchYouTube("Alan walker faded" /*If there are 0 results it will log to the console that it found no results*/, info => {
console.log(`The video's title is ${info.title} and its id is ${info.videoID}`);
});
/*Results that you can use for the callback function.
NOTE: You can use the getVideoInfo function to get more info about this search*/
videoID: //The video's unique id,
url: //the video's url,
title: //the Video's title,
channel: {
id: //The channel id,
user: //The user,
url: //The video's channel url
},
thumbnail: //The videos thumbnail,
API.searchVideos("Marshmello alone", 5 /*The amount of videos you want to be searched or the limit*/, videos => {
//Mapping the videos
let sidenum=0;
console.log(videos.map(v=>`the video's title is ${v.title} and the id is ${v.videoID}`).join("\n"));
//Getting a specific video
let video = videos[/*number in between 0 and the ammount you specified in this case its 5*/];
console.log(`This videos title is ${video.title} and the id is ${video.videoID}`);
});
//Returns
videoID: //The video's unique id,
url: //the video's url,
title: //the Video's title,
channel: {
id: //The channel id,
user: //The user,
url: //The video's channel url
},
thumbnail: //The videos thumbnail,
const { Client } = require("discord.js");
const bot = new Client();
const ytdl = require("ytdl-core");
//The api to get song info and user input.
let YouTube = require("./index.js");
let yt = new YouTube("API_KEY_HERE");
//The queue's
let guilds = {};
bot.login("BOT_TOKEN_HERE");
bot.on("ready", () => console.log("REEADY!"));
bot.on("message", msg => {
let prefix = ';';
let args = msg.content.slice(prefix.length).trim().split(" ");
let cmd = args.shift().toLowerCase();
let guild = guilds[msg.guild.id];
if(cmd === "play") {
if(!guild) guild = guilds[msg.guild.id] = {
queue: [],
isPlaying: false
};
if(!msg.member.voiceChannel) return msg.channel.send("You must be in a voice channel first!");
let song = args.join(" ");
if(!song) return msg.channel.send("You need to provide a searchterm!");
if(guild.isPlaying) {
yt.searchVideos(song, 5, async videos => {
try {
let num=0;
let m = await msg.channel.send(`
**Song selection:**
${videos.map(v=>`**${++num}:** ${v.title}`).join("\n")}
Please provide a value between 1 and 5.
`);
let collector = await msg.channel.awaitMessages(m=>m.content > 0 && m.content < 6 && m.author.id === msg.author.id && m.channel.id === msg.channel.id, {
maxMatches: 1,
time: 10000,
errors: ['time']
});
let selection = parseInt(collector.first().content);
let vid = videos[selection - 1];
m.delete(5000);
yt.getVideoInfo(vid.videoID, i => {
guild.queue.push({
title: i.title,
id: i.videoID,
url: i.url,
duration: {
days: i.duration.days,
hours: i.duration.hours,
minutes: i.duration.minutes,
seconds: i.duration.seconds
}
});
return msg.channel.send(`Added **${i.title}** to the queue!`);
});
} catch (error) {
return msg.channel.send("No value was entered. Canceling video selection!");
}
});
} else {
guild.isPlaying = true;
yt.searchVideos(song, 5, async videos => {
try {
let num=0;
let m = await msg.channel.send(`
**Song selection:**
${videos.map(v=>`**${++num}:** ${v.title}`).join("\n")}
Please provide a value between 1 and 5.
`);
let collector = await msg.channel.awaitMessages(m=>m.content > 0 && m.content < 6 && m.author.id === msg.author.id && m.channel.id === msg.channel.id, {
maxMatches: 1,
time: 10000,
errors: ['time']
});
let selection = parseInt(collector.first().content);
let vid = videos[selection - 1];
m.delete(5000);
yt.getVideoInfo(vid.videoID, i => {
guild.queue.push({
title: i.title,
id: i.videoID,
url: i.url,
duration: {
days: i.duration.days,
hours: i.duration.hours,
minutes: i.duration.minutes,
seconds: i.duration.seconds
}
});
return playSong(i.url, guild, msg);
});
} catch (error) {
return msg.channel.send("No value was entered. Canceling video selection!");
}
});
}
}
});
function playSong(url, guild, msg) {
let vc = msg.member.voiceChannel;
vc.join().then(con => {
let dispatcher = con.playStream(ytdl(url, { filter: "audioonly" }));
dispatcher.on("end", () => {
guild.queue.shift();
if(guild.queue.length === 0) {
vc.leave();
delete guilds[msg.guild.id];
} else {
playSong(guild.queue[0].url, guild, msg);
}
});
});
msg.channel.send(`Now playing: **${guild.queue[0].title}** (${guild.queue[0].duration.hours}${guild.queue[0].duration.minutes}${guild.queue[0].duration.seconds})`);
}
FAQs
A light weight, easy, and super fast way of interacting with the YouTube Data API v3.
The npm package yt-api receives a total of 3 weekly downloads. As such, yt-api popularity was classified as not popular.
We found that yt-api demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.