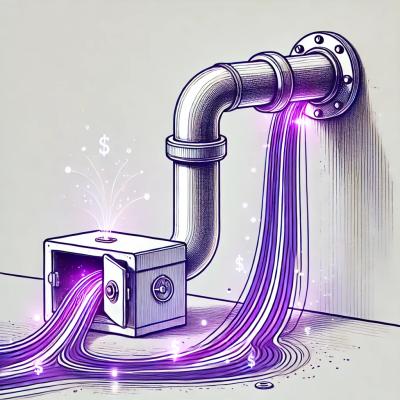
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
ytdl-browser
Advanced tools
This is based on a [fork](https://www.npmjs.com/package/ytdl-core-browser). By March 12, 2022, it is working.
This is based on a fork. By March 12, 2022, it is working.
This library is a tiny wrapper around the ytdl-core library to make it work in the browser. Also add other stuff - such as ytpl and ytsr. We don't care about bugs and issues, do what you want. This library is just a proof of concept. free js static CDN
Have fun!
In order to make ytdl-core work in the browser, we use two steps:
Here is an example usage. You can replace /dist/ytdl.js
with the path to the library's
entry file.
...
<body>
...
<script src="/dist/ytdl.js"></script>
<script>
const ytdl = window.require('ytdl-core-browser')({
proxyUrl: 'https://cors-anywhere.herokuapp.com/',
// proxyquireStubs: {}, arguments mapped directly to proxyquireify
// For more info, see https://www.npmjs.com/package/proxyquireify
});
ytdl
.getInfo('https://www.youtube.com/watch?v=WPdbEbwNTcU')
.then(info=>console.log(info))
.catch(err=>{throw err;});
</script>
</body>
This is the source code of the library:
const proxyquire = require('proxyquireify')(require);
const realMiniget = require('miniget');
const m3u8stream = require('m3u8stream');
// We import the library so it cached before using proxyquire
const ytdlCore = require('ytdl-core');
interface YtdlBrowserOptions {
proxyUrl: string; // Ex: 'https://cors-anywhere.herokuapp.com/'
proxyquireStubs?: any;
}
module.exports = (options: YtdlBrowserOptions) => {
return proxyquire('ytdl-core', {
miniget(url, opts){
return realMiniget(options.proxyUrl + url, opts);
},
m3u8stream(url, opts){
return m3u8stream(options.proxyUrl + url, opts);
},
...(options.proxyquireStubs || {})
});
};
You are very welcomed to hack the settings (especially with option proxyquireStubs
).
For instance, if you want to use a custom implementation of the miniget library:
const ytdl = window.require('ytdl-core-browser')({
proxyUrl: ...
proxyquireStubs: {
miniget(url,options){
// Your custom mock of miniget(...)
},
m3u8stream(url, options){
// Your custom mock of m3u8stream(...)
}
}
})
FAQs
This is based on a [fork](https://www.npmjs.com/package/ytdl-core-browser). By March 12, 2022, it is working.
We found that ytdl-browser demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.