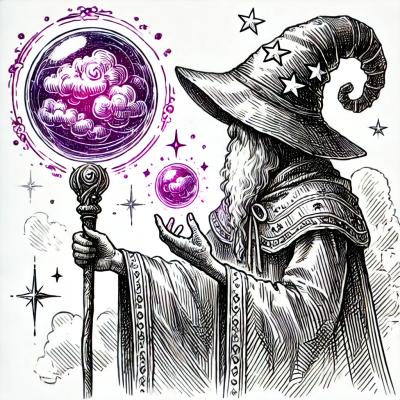
Security News
Cloudflare Adds Security.txt Setup Wizard
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
What is Yub? It's a simple Yubico Yubikey API client that
Yub is published as an NPM module for your convenience:
npm install yub
You'll also need a Yubico API Key from here: https://upgrade.yubico.com/getapikey/. This gives you the client_id and secret_key that must be passed to "yub.init()", see below.
var yub = require('yub');
// initialise the yub library
yub.init("<client id here>", "<secret here>");
// attempt to verify the key
yub.verify("<otp here>", function(err,data) {
console.log(err, data)
});
A typical 'data' return from yub.verify looks like this:
{
t: '2013-08-31T07: 13: 27Z0111',
otp: 'cccaccbtbvkwjjirhcctvdgbahdbijduldcjdurgjgfi',
nonce: '50fb8a88a327b4af16e6e7bd9ec4e4e6c692f2e5',
sl: '25',
status: 'OK',
signatureVerified: true,
nonceVerified: true,
identity: 'cccaccbtbvkw'
}
In the 'example' directory is an example command-line utility (test.js) which exits with a different return code, depending on whether the supplied OTP was valid or not. This could easily be plumbed into a command-line script to only allow execution to proceed with a valid OTP.
It takes the OTP as a command-line parameter i.e. type "node test.js ", insert your Yubikey and press the gold button:
> node test.js cccaccbtbvkwjjirhcctvdgbahdbijduldcjdurgjgfi
{ t: '2013-08-31T07: 13: 27Z0111',
otp: 'cccaccbtbvkwjjirhcctvdgbahdbijduldcjdurgjgfi',
nonce: '50fb8a88a327b4af16e6e7bd9ec4e4e6c692f2e5',
sl: '25',
status: 'OK',
signatureVerified: true,
nonceVerified: true,
identity: 'cccaccbtbvkw'}
This software is open-source and is a personal project, not officially endorsed by Yubico in any way.
FAQs
Yubico Yubikey API Client for Node.js
The npm package yub receives a total of 5,584 weekly downloads. As such, yub popularity was classified as popular.
We found that yub demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.
Security News
ENISA’s 2024 report highlights the EU’s top cybersecurity threats, including rising DDoS attacks, ransomware, supply chain vulnerabilities, and weaponized AI.