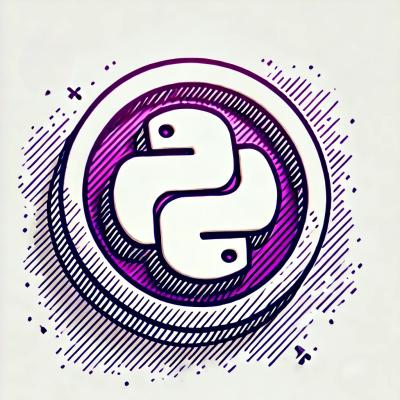
Security News
PyPI Introduces Digital Attestations to Strengthen Python Package Security
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
The z-schema npm package is a JSON Schema validator that is compliant with the latest JSON Schema standards. It allows users to validate JSON data against a schema to ensure it meets certain criteria before processing it. This can be particularly useful for validating configuration files, API request payloads, and any other JSON data that requires validation against a predefined structure.
Validation of JSON data
This feature allows you to validate JSON data against a JSON Schema. The code sample demonstrates how to create a new ZSchema validator instance, define a schema, and validate data against that schema.
{"var ZSchema = require('z-schema');
var validator = new ZSchema();
var schema = {"type": "object","properties": {"name": {"type": "string"}}};
var data = {"name": "John Doe"};
validator.validate(data, schema, function(err, valid) {console.log(valid);});}
Custom format validators
Z-schema allows you to define custom formats for data validation. In this code sample, a custom format called 'custom-format' is defined and used in a schema to validate a string.
{"var ZSchema = require('z-schema');
var validator = new ZSchema();
validator.setFormat('custom-format', function(str) {return str === 'custom';});
var schema = {"type": "string","format": "custom-format"};
var data = 'custom';
validator.validate(data, schema, function(err, valid) {console.log(valid);});}
Asynchronous validation
Z-schema supports asynchronous validation using promises. This code sample shows how to validate data against a schema asynchronously.
{"var ZSchema = require('z-schema');
var validator = new ZSchema();
var schema = {"type": "object","properties": {"name": {"type": "string"}}};
var data = {"name": "John Doe"};
validator.validate(data, schema).then(function(valid) {console.log(valid);}).catch(function(err) {console.error(err);});}
Remote references
Z-schema can handle remote references in schemas. This code sample demonstrates how to set up a validator with a remote reference and validate data against it.
{"var ZSchema = require('z-schema');
var validator = new ZSchema({remoteReferences: {"http://my.site/myschema.json": {"type": "object"}}});
var schema = {"$ref": "http://my.site/myschema.json"};
var data = {"name": "John Doe"};
validator.validate(data, schema, function(err, valid) {console.log(valid);});}
Ajv is a fast JSON Schema validator that supports draft-06/07/2019-09 JSON Schema standards. It is known for its performance and is often used in projects that require high-speed validation. Compared to z-schema, Ajv may offer better performance and more up-to-date standards support.
Tiny Validator (tv4) is a small and fast JSON Schema validator that supports draft-04 of the JSON Schema. It is simpler and has a smaller footprint than z-schema, making it suitable for environments with limited resources or where a simple validation solution is needed.
The jsonschema package is another validator for JSON Schema that supports various schema versions. It is designed to be easy to use and extend, with a straightforward API. While z-schema is focused on compliance with the standards, jsonschema emphasizes ease of use and extensibility.
JSON Schema validator for Node.js (draft4 version)
Coded according to:
json-schema documentation, json-schema-core, json-schema-validation, json-schema-hypermedia
Passing all tests here (even optional, except zeroTerminatedFloats):
json-schema/JSON-Schema-Test-Suite
Will try to maintain this as much as possible, all bug reports welcome.
var report = zSchema.validate(json, schema, function(report) {
if (report.valid === true) ...
});
If report.valid === false
, then errors can be found in report.errors
.
The report object will look something like:
{
"valid": false,
"errors": [
]
}
You can pre-compile schemas (for example on your server startup) so your application is not bothered by schema compilation and validation when validating ingoing / outgoing objects.
var validator = new zSchema();
validator.compileSchema(schema, function (err, compiledSchema) {
assert.isUndefined(err);
...
});
Then you can re-use compiled schemas easily with sync-async validation API.
var report = validator.validateWithCompiled(json, compiledSchema);
assert.isTrue(report.valid);
...
validator.validateWithCompiled(json, compiledSchema, function(err, success, report) {
assert.isTrue(success);
...
});
Note:
Most basic schemas don't have to be compiled for validation to work (although recommended). Async compilation was mostly created to work with schemas that contain references to other files.
You can add validation for your own custom string formats like this: (these are added to all validator instances, because it would never make sense to have multiple functions to validate format with the same name)
zSchema.registerFormat('xstring', function (str) {
return str === 'xxx';
});
zSchema.validate('xxx', {
'type': 'string',
'format': 'xstring'
}, function (report) {
// report.valid will be true
}
When creating new instance of validator, you can specify some options that will alter the validator behaviour like this:
var validator = new zSchema({
option: true
});
true/false
when true, do not allow unknown keywords in schema
true/false
when true, always adds minLength: 1 to schemas where type is string
true/false
when true, every schema must specify a type
true/false
when true, forces not to leave out some keys on schemas (additionalProperties, additionalItems)
true/false
when true, forces not to leave out properties or patternProperties on type-object schemas
true/false
when true, forces not to leave out items on array-type schemas
true/false
when true, forces not to leave out maxLength on string-type schemas, when format or enum is not specified
Alternatively, you can turn on all of the above options with:
var validator = new zSchema({
strict: true
});
FAQs
JSON schema validator
The npm package z-schema receives a total of 902,179 weekly downloads. As such, z-schema popularity was classified as popular.
We found that z-schema demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.