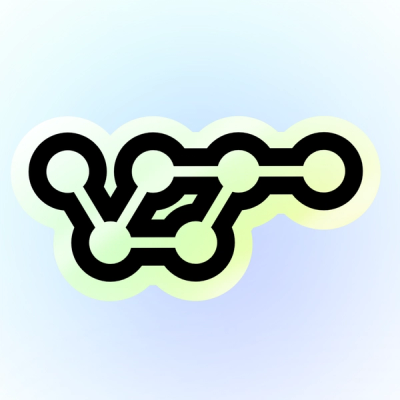
Security News
vlt Debuts New JavaScript Package Manager and Serverless Registry at NodeConf EU
vlt introduced its new package manager and a serverless registry this week, innovating in a space where npm has stagnated.
zod-to-json-schema
Advanced tools
The zod-to-json-schema npm package is a utility that converts Zod schemas to JSON Schema. This is useful for generating JSON Schema definitions from Zod validation schemas, which can then be used for various purposes such as API documentation, validation, and more.
Basic Schema Conversion
This feature allows you to convert a basic Zod schema to a JSON Schema. The example demonstrates converting a Zod object schema with string and integer properties to its JSON Schema equivalent.
const { z } = require('zod');
const { zodToJsonSchema } = require('zod-to-json-schema');
const schema = z.object({
name: z.string(),
age: z.number().int(),
});
const jsonSchema = zodToJsonSchema(schema);
console.log(JSON.stringify(jsonSchema, null, 2));
Nested Schema Conversion
This feature supports the conversion of nested Zod schemas to JSON Schema. The example shows a user schema that includes an address schema, demonstrating how nested objects are handled.
const { z } = require('zod');
const { zodToJsonSchema } = require('zod-to-json-schema');
const addressSchema = z.object({
street: z.string(),
city: z.string(),
});
const userSchema = z.object({
name: z.string(),
address: addressSchema,
});
const jsonSchema = zodToJsonSchema(userSchema);
console.log(JSON.stringify(jsonSchema, null, 2));
Array Schema Conversion
This feature allows for the conversion of Zod schemas that include arrays to JSON Schema. The example demonstrates converting a schema with an array of strings.
const { z } = require('zod');
const { zodToJsonSchema } = require('zod-to-json-schema');
const schema = z.object({
tags: z.array(z.string()),
});
const jsonSchema = zodToJsonSchema(schema);
console.log(JSON.stringify(jsonSchema, null, 2));
AJV (Another JSON Schema Validator) is a popular JSON Schema validator. While it focuses on validating JSON data against JSON Schema, it also provides utilities for generating JSON Schema from various sources. Compared to zod-to-json-schema, AJV is more focused on validation rather than schema conversion.
This package converts JSON Schema to TypeScript types. While it operates in the opposite direction of zod-to-json-schema, it serves a similar purpose of bridging the gap between JSON Schema and TypeScript. It is useful for generating TypeScript definitions from existing JSON Schemas.
This package generates JSON Schema from TypeScript types. It is similar to zod-to-json-schema in that it converts type definitions to JSON Schema, but it works directly with TypeScript types instead of Zod schemas.
Looking for the exact opposite? Check out json-schema-to-zod
Does what it says on the tin; converts Zod schemas into JSON schemas!
$ref
s.import { z } from "zod";
import { zodToJsonSchema } from "zod-to-json-schema";
const mySchema = z
.object({
myString: z.string().min(5),
myUnion: z.union([z.number(), z.boolean()]),
})
.describe("My neat object schema");
const jsonSchema = zodToJsonSchema(mySchema, "mySchema");
{
"$schema": "http://json-schema.org/draft-07/schema#",
"$ref": "#/definitions/mySchema",
"definitions": {
"mySchema": {
"description": "My neat object schema",
"type": "object",
"properties": {
"myString": {
"type": "string",
"minLength": 5
},
"myUnion": {
"type": ["number", "boolean"]
}
},
"additionalProperties": false,
"required": ["myString", "myUnion"]
}
}
}
You can pass a string as the second parameter of the main zodToJsonSchema function. If you do, your schema will end up inside a definitions object property on the root and referenced from there. Alternatively, you can pass the name as the name
property of the options object (see below).
Instead of the schema name (or nothing), you can pass an options object as the second parameter. The following options are available:
Option | Effect |
---|---|
name?: string | As described above. |
basePath?: string[] | The base path of the root reference builder. Defaults to ["#"]. |
$refStrategy?: "root" | "relative" | "none" | The reference builder strategy;
|
effectStrategy?: "input" | "any" | The effects output strategy. Defaults to "input". See known issues! |
definitionPath?: "definitions" | "$defs" | The name of the definitions property when name is passed. Defaults to "definitions". |
target?: "jsonSchema7" | "openApi3" | Which spec to target. Defaults to "jsonSchema7" |
strictUnions?: boolean | Scrubs unions of any-like json schemas, like {} or true . Multiple zod types may result in these out of necessity, such as z.instanceof() |
definitions?: Record<string, ZodSchema> | See separate section below |
errorMessages?: boolean | Include custom error messages created via chained function checks for supported zod types. See section below |
The definitions option lets you manually add recurring schemas into definitions for cleaner outputs. It's fully compatible with named schemas, changed definitions path and base path. Here's a simple example:
const myRecurringSchema = z.string();
const myObjectSchema = z.object({ a: myRecurringSchema, b: myRecurringSchema });
const myJsonSchema = zodToJsonSchema(myObjectSchema, {
definitions: { myRecurringSchema },
});
{
"type": "object",
"properties": {
"a": {
"$ref": "#/definitions/myRecurringSchema"
},
"b": {
"$ref": "#/definitions/myRecurringSchema"
}
},
"definitions": {
"myRecurringSchema": {
"type": "string"
}
}
}
This feature allows optionally including error messages created via chained function calls for supported zod types:
// string schema with additional chained function call checks
const EmailSchema = z.string().email("Invalid email").min(5, "Too short");
const jsonSchema = zodToJsonSchema(EmailSchema, { errorMessages: true });
{
"$schema": "http://json-schema.org/draft-07/schema#",
"type": "string",
"format": "email",
"minLength": 5,
"errorMessage": {
"format": "Invalid email",
"minLength": "Too short"
}
}
This allows for field specific, validation step specific error messages which can be useful for building forms and such. This format is accepted by react-hook-form
's ajv resolver (and therefor ajv-errors
which it uses under the hood). Note that if using AJV with this format will require enabling ajv-errors
as vanilla AJV does not accept this format by default.
.transform
, the return type is inferred from the supplied function. In other words, there is no schema for the return type, and there is no way to convert it in runtime. Currently the JSON schema will therefore reflect the input side of the Zod schema and not necessarily the output (the latter aka. z.infer
). If this causes problems with your schema, consider using the effectStrategy "any", which will allow any type of output.z.record
with any other key type, this will be ignored. An exception to this rule is z.enum
as is supported since 3.11.3.isOptional()
to check if a property should be included in required
or not. This has the potentially dangerous behavior of calling .safeParse
with undefined
. To work around this, make sure your preprocess
and other effects callbacks are pure and not liable to throw errors. An issue has been logged in the Zod repo and can be tracked here.This package does not follow semantic versioning. The major and minor versions of this package instead reflects feature parity with the Zod package.
I will do my best to keep API-breaking changes to an absolute minimum, but new features may appear as "patches", such as introducing the options pattern in 3.9.1.
https://github.com/StefanTerdell/zod-to-json-schema/blob/master/changelog.md
FAQs
Converts Zod schemas to Json Schemas
The npm package zod-to-json-schema receives a total of 1,753,134 weekly downloads. As such, zod-to-json-schema popularity was classified as popular.
We found that zod-to-json-schema demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt introduced its new package manager and a serverless registry this week, innovating in a space where npm has stagnated.
Security News
Research
The Socket Research Team uncovered a malicious Python package typosquatting the popular 'fabric' SSH library, silently exfiltrating AWS credentials from unsuspecting developers.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.