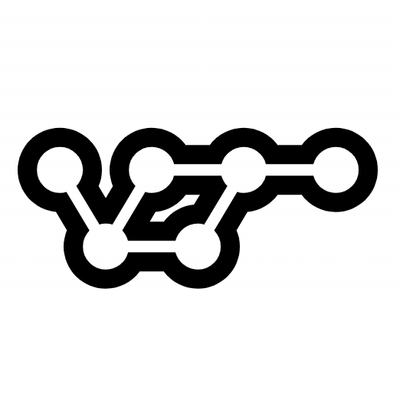
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Zoro API is an API that generates images with filters to enhance your photos !
npm i --save zoro-api
The first step is to import the module in your code.
const Zoro = require("zoro-api");
Then you have to request your image and send it as an attachement. Be careful, if you want to use animated filters (gifs), you will have to modify the extension of the gif type instead of png.
//Import the discord.js library
const Discord = require("discord.js");
//Create a new discord.js client
const bot = new Discord.Client()
//Import the module
const Zoro = require("zoro-api");
//Listen to the ready event
bot.on("ready", () => {
console.log("The robot is online !");
})
//Listen to the message event
bot.on("message", async (message) => {
//If the user types !blur
if (message.content === "!blur") {
//Get user
let user = message.mentions.users.first() || message.author;
//Get the avatarUrl of the user
let avatar = user.displayAvatarURL({ size: 512 }).replace(".webp", ".png")
//Loading message
const msg = await message.channel.send("Generating ...")
//Generating the image
let img = await Zoro.blur(avatar)
//Adding the image as an attachement
let attachment = new Discord.MessageAttachment(img, "blur.png");
//Sending the image and delete the loading message
message.channel.send(attachment) && msg.delete();
}
})
//Log in to the bot
bot.login("Token")
PNG :
GIFS :
RANDOM GIFS :
RANDOM PICTURES :
CREATE GIFS :
How to generate an image in an embed ? (Example with the blur filter).
bot.on("message", async (message) => {
//If the user types !blur
if (message.content === "!blur") {
//Get user
let user = message.mentions.users.first() || message.author;
//Get the avatarUrl of the user
let avatar = user.displayAvatarURL({ size: 512 }).replace(".webp", ".png")
//Loading message
const msg = await message.channel.send("Generating ...")
//Generating the image
let img = await Zoro.blur(avatar)
//Adding the image as an attachement
let attachment = new Discord.MessageAttachment(img, "blur.png");
//New embed
const embed = {
//The bot displays the name of the user
title: `Blur, user ${user.username}`,
//Attachment
image: {
url: 'attachment://blur.png',
},
};
//Sending the image (with embed) and delete the loading message
message.channel.send(({ files: [attachment], embed: embed })) && msg.delete();
}
})
How to generate an image with a link ? (Example with the blur filter).
Zoro.blur("Link")
It is also possible to get the link from a command.
Example !blur http://image.png
.
//Listen to the message event
client.on("message", async (message) => {
//If the user types !blur
if (message.content.startsWith("!img")) {
//Get args (link)
let messageArray = message.content.split(' ');
let args = messageArray.slice(1);
//If no link
if (!args[0]) return message.channel.send("Please indicate the link of an image !")
//Loading message
const msg = await message.channel.send("Generating ...")
try {
//Generating the image
let img = await Zoro.blur(args.join(" "))
//Adding the image as an attachement
let attachment = new Discord.MessageAttachment(img, "blur.png");
//Sending the image and delete the loading message
message.channel.send(attachment) && msg.delete();
} catch (e) {
//The bot sends the error to the console
console.log(e)
//Error message
return message.channel.send("An error occured, please check the link of your image !") && msg.delete();
}
}
})
How to design a custom gif ?
Example !cgif http://image.png
.
//Listen to the message event
client.on("message", async (message) => {
//If the user types !cgif
if (message.content.startsWith("!cgif")) {
//Get args (link)
let messageArray = message.content.split(' ');
let args = messageArray.slice(1);
//Get user
let user = message.mentions.users.first() || message.author;
//Get the avatarUrl of the user
let avatar = user.displayAvatarURL({ size: 512 }).replace(".webp", ".png")
//If no link
if (!args[0]) return message.channel.send("Please indicate the link of an image !")
//Loading message
const msg = await message.channel.send("Generating ...")
try {
//Generating the image
let img = await Zoro.cgif(avatar, args.join(" "))
//Adding the image as an attachement
let attachment = new Discord.MessageAttachment(img, "cgif.gif");
//Sending the image and delete the loading message
message.channel.send(attachment) && msg.delete();
} catch (e) {
//The bot sends the error to the console
console.log(e)
//Error message
return message.channel.send("An error occured, please check the link of your image !") && msg.delete();
}
}
})
Do you have a question? Problem? Join the server discord.
Thanks to Mr¤KayJayDee for contributing to the project.
FAQs
An api to generate images / gifs !
We found that zoro-api demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.