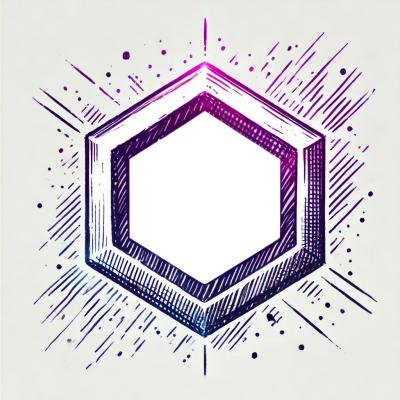
Security News
Maven Central Adds Sigstore Signature Validation
Maven Central now validates Sigstore signatures, making it easier for developers to verify the provenance of Java packages.
Amazon Pay Integration.
Please note this is a Non-Official Amazon Pay Python SDK and can only be used for API calls to the pay-api.amazon.com|eu|jp endpoint.
For more details about the api, please check the Official Documentation for developers.
Use PyPI to install the latest release of the SDK:
pip install AmazonPayClient
from AmazonPay import Client
client = Client(
public_key_id='YOUR_PUBLIC_KEY_ID',
private_key='keys/private.pem',
region='jp',
sandbox=True
)
If you have created environment specific keys (i.e. Public Key Starts with LIVE or SANDBOX) in Seller Central, then use those PublicKeyId & PrivateKey. In this case, there is no need to pass the sandbox parameter to the configuration.
from AmazonPay import Client
client = Client(
public_key_id='YOUR_PUBLIC_KEY_ID',
private_key='keys/private.pem',
region='jp',
)
The pay-api.amazon.com|eu|jp endpoint uses versioning to allow future updates. The major version of this SDK will stay aligned with the API version of the endpoint.
If you have downloaded version 2.x.y of this SDK, the API version would be "v2".
If you need to use a "v1" version of Amazon Pay API, seek Official Documentation for help.
Make use of the built-in convenience functions to easily make API calls. Scroll down further to see example code snippets.
When using the convenience functions, the request payload will be signed using the provided private key, and a HTTPS request is made to the correct regional endpoint.
from AmazonPay import Client
client = Client(
public_key_id='YOUR_PUBLIC_KEY_ID',
private_key='keys/private.pem',
region='us',
sandbox=False
)
body = {
'amazonOrderReferenceId': 'P00-0000000-0000000',
'deliveryDetails': [
{
'carrierCode': 'UPS',
'trackingNumber': '1Z999AA10123456784'
}
]
}
response = client.create_delivery_tracker(body)
if response.status_code == 200:
# success
result = response.json()
print(result)
else:
# check the error
print('Status Code: ' + str(response.status_code) + '\n' + 'Content: ' + response.content.decode(encoding='utf-8') + '\n')
from AmazonPay import Client
client = Client(
public_key_id='YOUR_PUBLIC_KEY_ID',
private_key='keys/private.pem',
region='us',
sandbox=True
)
body = {
'webCheckoutDetails': {
'checkoutReviewReturnUrl': 'https://localhost/store/checkout_review',
'checkoutResultReturnUrl': 'https://localhost/store/checkout_result'
},
'storeId': 'YOUR_STORE_ID'
}
response = client.create_checkout_session(body)
if response.status_code == 201:
# success
result = response.json()
print(result)
else:
# check the error
print('Status Code: ' + str(response.status_code) + '\n' + 'Content: ' + response.content.decode(encoding='utf-8') + '\n')
from AmazonPay import Client
client = Client(
public_key_id='YOUR_PUBLIC_KEY_ID',
private_key='keys/private.pem',
region='us',
sandbox=True
)
checkout_session_id = '00000000-0000-0000-0000-000000000000'
response = client.get_checkout_session(checkout_session_id)
if response.status_code == 200:
# success
result = response.json()
print(result)
else:
# check the error
print('Status Code: ' + str(response.status_code) + '\n' + 'Content: ' + response.content.decode(encoding='utf-8') + '\n')
from AmazonPay import Client
client = Client(
public_key_id='YOUR_PUBLIC_KEY_ID',
private_key='keys/private.pem',
region='us',
sandbox=True
)
checkout_session_id = '00000000-0000-0000-0000-000000000000'
body = {
'paymentDetails': {
'chargeAmount': {
'amount': '100',
'currencyCode': 'JPY'
}
}
}
response = client.update_checkout_session(checkout_session_id, body)
if response.status_code == 200:
# success
result = response.json()
print(result)
else:
# check the error
print('Status Code: ' + str(response.status_code) + '\n' + 'Content: ' + response.content.decode(encoding='utf-8') + '\n')
from AmazonPay import Client
client = Client(
public_key_id='YOUR_PUBLIC_KEY_ID',
private_key='keys/private.pem',
region='us',
sandbox=True
)
checkout_session_id = '00000000-0000-0000-0000-000000000000'
body = {
'chargeAmount': {
'amount': '100',
'currencyCode': 'JPY'
}
}
response = client.complete_checkout_session(checkout_session_id, body)
if response.status_code == 200:
# success
result = response.json()
print(result)
else:
# check the error
print('Status Code: ' + str(response.status_code) + '\n' + 'Content: ' + response.content.decode(encoding='utf-8') + '\n')
from AmazonPay import Client
client = Client(
public_key_id='YOUR_PUBLIC_KEY_ID',
private_key='keys/private.pem',
region='us',
sandbox=True
)
charge_permission_id = 'S00-0000000-0000000'
response = client.get_charge_permission(charge_permission_id)
if response.status_code == 200:
# success
result = response.json()
print(result)
else:
# check the error
print('Status Code: ' + str(response.status_code) + '\n' + 'Content: ' + response.content.decode(encoding='utf-8') + '\n')
from AmazonPay import Client
client = Client(
public_key_id='YOUR_PUBLIC_KEY_ID',
private_key='keys/private.pem',
region='us',
sandbox=True
)
charge_permission_id = 'S00-0000000-0000000'
body = {
'merchantMetadata': {
'merchantReferenceId': '00-00-000000-00',
'merchantStoreName': 'Test Store',
'noteToBuyer': 'Some Note to buyer',
'customInformation': 'Custom Information'
}
}
response = client.update_charge_permission(charge_permission_id, body)
if response.status_code == 200:
# success
result = response.json()
print(result)
else:
# check the error
print('Status Code: ' + str(response.status_code) + '\n' + 'Content: ' + response.content.decode(encoding='utf-8') + '\n')
from AmazonPay import Client
client = Client(
public_key_id='YOUR_PUBLIC_KEY_ID',
private_key='keys/private.pem',
region='us',
sandbox=True
)
charge_permission_id = 'S00-0000000-0000000'
body = {
'closureReason': 'No more charges required',
'cancelPendingCharges': False
}
response = client.close_charge_permission(charge_permission_id, body)
if response.status_code == 200:
# success
result = response.json()
print(result)
else:
# check the error
print('Status Code: ' + str(response.status_code) + '\n' + 'Content: ' + response.content.decode(encoding='utf-8') + '\n')
from AmazonPay import Client
client = Client(
public_key_id='YOUR_PUBLIC_KEY_ID',
private_key='keys/private.pem',
region='us',
sandbox=True
)
body = {
'chargePermissionId': 'S00-0000000-0000000',
'chargeAmount': {
'amount': '100',
'currencyCode': 'JPY'
},
'captureNow': True
}
response = client.create_charge(body)
if response.status_code == 201:
# success
result = response.json()
print(result)
else:
# check the error
print('Status Code: ' + str(response.status_code) + '\n' + 'Content: ' + response.content.decode(encoding='utf-8') + '\n')
from AmazonPay import Client
client = Client(
public_key_id='YOUR_PUBLIC_KEY_ID',
private_key='keys/private.pem',
region='us',
sandbox=True
)
charge_id = 'S00-0000000-0000000-C000000'
response = client.get_charge(charge_id)
if response.status_code == 200:
# success
result = response.json()
print(result)
else:
# check the error
print('Status Code: ' + str(response.status_code) + '\n' + 'Content: ' + response.content.decode(encoding='utf-8') + '\n')
from AmazonPay import Client
client = Client(
public_key_id='YOUR_PUBLIC_KEY_ID',
private_key='keys/private.pem',
region='us',
sandbox=True
)
charge_id = 'S00-0000000-0000000-C000000'
body = {
'captureAmount': {
'amount': '100',
'currencyCode': 'JPY'
}
}
response = client.capture_charge(charge_id, body)
if response.status_code == 200:
# success
result = response.json()
print(result)
else:
# check the error
print('Status Code: ' + str(response.status_code) + '\n' + 'Content: ' + response.content.decode(encoding='utf-8') + '\n')
from AmazonPay import Client
client = Client(
public_key_id='YOUR_PUBLIC_KEY_ID',
private_key='keys/private.pem',
region='us',
sandbox=True
)
charge_id = 'S00-0000000-0000000-C000000'
body = {
'cancellationReason': 'REASON DESCRIPTION'
}
response = client.cancel_charge(charge_id, body)
if response.status_code == 200:
# success
result = response.json()
print(result)
else:
# check the error
print('Status Code: ' + str(response.status_code) + '\n' + 'Content: ' + response.content.decode(encoding='utf-8') + '\n')
from AmazonPay import Client
client = Client(
public_key_id='YOUR_PUBLIC_KEY_ID',
private_key='keys/private.pem',
region='us',
sandbox=True
)
body = {
'chargeId': 'S00-0000000-0000000-C000000',
'refundAmount': {
'amount': '100',
'currencyCode': 'JPY'
},
}
response = client.create_refund(body)
if response.status_code == 201:
# success
result = response.json()
print(result)
else:
# check the error
print('Status Code: ' + str(response.status_code) + '\n' + 'Content: ' + response.content.decode(encoding='utf-8') + '\n')
from AmazonPay import Client
client = Client(
public_key_id='YOUR_PUBLIC_KEY_ID',
private_key='keys/private.pem',
region='us',
sandbox=True
)
refund_id = 'S00-0000000-0000000-R000000'
response = client.get_refund(refund_id)
if response.status_code == 200:
# success
result = response.json()
print(result)
else:
# check the error
print('Status Code: ' + str(response.status_code) + '\n' + 'Content: ' + response.content.decode(encoding='utf-8') + '\n')
The signatures generated by this helper function are only valid for the Checkout v2 front-end buttons. Unlike API signing, no timestamps are involved, so the result of this function can be considered a static signature that can safely be placed in your website JS source files and used repeatedly (as long as your payload does not change).
from AmazonPay import Client
client = Client(
public_key_id='YOUR_PUBLIC_KEY_ID',
private_key='keys/private.pem',
region='us',
sandbox=True
)
payload = '{"webCheckoutDetails": {"checkoutResultReturnUrl": "https://localhost/store/checkout_result", "checkoutMode": "ProcessOrder"}, "chargePermissionType": "OneTime", "paymentDetails": {"paymentIntent": "Confirm", "chargeAmount": {"amount": "100", "currencyCode": "JPY"}}, "storeId": "YOUR_STORE_ID"}'
signature = client.generate_button_signature(payload)
You can also use a dict as your payload. But make sure the json.dumps(payload)
result matches the one you are using
in your button, such as spaces etc.
from AmazonPay import Client
client = Client(
public_key_id='YOUR_PUBLIC_KEY_ID',
private_key='keys/private.pem',
region='us',
sandbox=True
)
payload = {
'webCheckoutDetails': {
'checkoutResultReturnUrl': 'https://localhost/store/checkout_result',
'checkoutMode': 'ProcessOrder'
},
'chargePermissionType': 'OneTime',
'paymentDetails': {
'paymentIntent': 'Confirm',
'chargeAmount': {
'amount': '100',
'currencyCode': 'JPY'
}
},
'storeId': 'YOUR_STORE_ID'
}
signature = client.generate_button_signature(payload)
FAQs
A non-official Amazon Pay Python SDK
We found that AmazonPayClient demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Maven Central now validates Sigstore signatures, making it easier for developers to verify the provenance of Java packages.
Security News
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
Research
Security News
Socket researchers uncovered a backdoored typosquat of BoltDB in the Go ecosystem, exploiting Go Module Proxy caching to persist undetected for years.