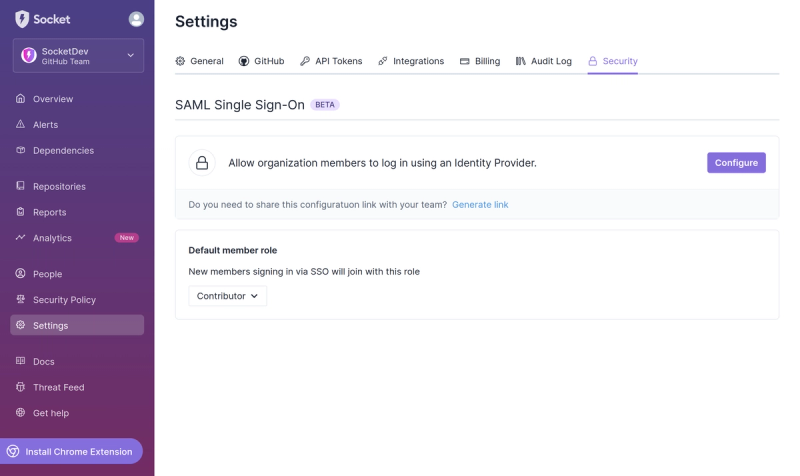
Product
Introducing SSO
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
Readme
To install the latest stable version of analytix, use the following command:
pip install analytix
You can also install the latest development version using the following command:
pip install git+https://github.com/parafoxia/analytix
You may need to prefix these commands with a call to the Python interpreter depending on your OS and Python configuration.
Below is a list of analytix's dependencies. Note that the minimum version assumes you're using CPython 3.8. The latest versions of each library are always supported.
Name | Min. version | Required? | Usage |
---|---|---|---|
urllib3 | 1.10.0 | Yes | Making HTTP requests |
jwt | 1.2.0 | No | Decoding JWT ID tokens (from v5.1) |
openpyxl | 3.0.0 | No | Exporting report data to Excel spreadsheets |
pandas | 1.4.0 | No | Exporting report data to pandas DataFrames |
polars | 0.15.17 | No | Exporting report data to Polars DataFrames |
pyarrow | 5.0.0 | No | Exporting report data to Apache Arrow tables and file formats |
All requests to the YouTube Analytics API need to be authorised through OAuth 2. In order to do this, you will need a Google Developers project with the YouTube Analytics API enabled. You can find instructions on how to do that in the API setup guide.
Once a project is set up, analytix handles authorisation — including token refreshing — for you.
More details regarding how and when refresh tokens expire can be found on the Google Identity documentation.
The following example creates a CSV file containing basic info for the 10 most viewed videos, from most to least viewed, in the US in 2022:
from datetime import date
from analytix import Client
client = Client("secrets.json")
report = client.fetch_report(
dimensions=("video",),
filters={"country": "US"},
metrics=("estimatedMinutesWatched", "views", "likes", "comments"),
sort_options=("-estimatedMinutesWatched",),
start_date=date(2022, 1, 1),
end_date=date(2022, 12, 31),
max_results=10,
)
report.to_csv("analytics.csv")
If you want to analyse this data using additional tools such as pandas, you can directly export the report as a DataFrame or table using the to_pandas()
, to_arrow()
, and to_polars()
methods of the report instance.
You can also save the report as a .tsv
, .json
, .xlsx
, .parquet
, or .feather
file.
There are more examples in the GitHub repository.
You can also fetch groups and group items:
from analytix import Client
# You can also use the client as context manager!
with Client("secrets.json") as client:
groups = client.fetch_groups()
group_items = client.fetch_group_items(groups[0].id)
If you want to see what analytix is doing, you can enable the packaged logger:
import analytix
analytix.enable_logging()
This defaults to showing all log messages of level INFO and above. To show more (or less) messages, pass a logging level as an argument.
CPython versions 3.8 through 3.12 and PyPy versions 3.8 through 3.10 are officially supported*. CPython 3.13-dev is provisionally supported*. Windows, MacOS, and Linux are all supported.
*For base analytix functionality; support cannot be guaranteed for functionality requiring external libraries.
Contributions are very much welcome! To get started:
The analytix module for Python is licensed under the BSD 3-Clause License.
FAQs
A simple yet powerful SDK for the YouTube Analytics API.
We found that analytix demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.