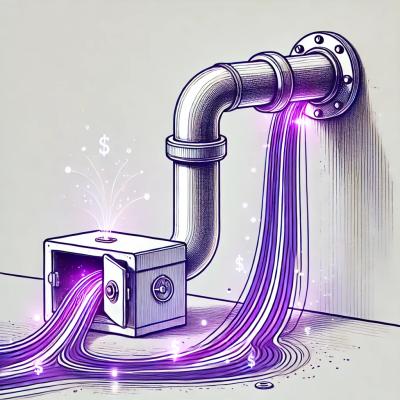
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Python, Pip, Poetry.
To install poetry, on osx, linux or bashonwindows terminals, type it:
curl -sSL https://raw.githubusercontent.com/python-poetry/poetry/master/get-poetry.py | python -
Alternatively, poetry could be installed by pip (supposing you have python and pip already installed):
pip install poetry
That is only a suggestion, you could run anansi on any python terminal. Only tested on linux.
Perform the commands:
poetry install
poetry run python -m ipykernel install --user --name=$(basename $(pwd))
poetry run jupyter notebook > jupyterlog 2>&1 &
from anansi.tradingbot.models import *
from anansi.tradingbot import traders
from anansi.tradingbot.views import create_user, create_default_operation
my_user_first_name = "John"
create_user(first_name=my_user_first_name,
last_name="Doe",
email = "{}@email.com".format(my_user_first_name.lower()))
my_user = User[1]
create_default_operation(user=my_user)
my_op = Operation.get(id=1)
my_trader = traders.DefaultTrader(operation=my_op)
my_trader.run()
users = select(user for user in User)
users.show()
id|first_name|last_name|login_displayed_name|email
--+----------+---------+--------------------+--------------
1 |John |Doe | |john@email.com
my_user.first_name
'John'
my_op.stop_loss.name
'StopTrailing3T'
my_trader.Classifier.parameters.time_frame
'6h'
before_update = my_trader.operation.position.side, my_trader.operation.position.exit_reference_price
my_trader.operation.position.update(side="Long", exit_reference_price=1020.94)
after_update = my_trader.operation.position.side, my_trader.operation.position.exit_reference_price
before_update, after_update
(('Zeroed', None), ('Long', 1020.94))
The example below uses the 'KlinesFromBroker' class from the 'handlers' module ('marketdata' package), which works as an abstraction over the data brokers, not only serializing requests (in order to respect brokers' limits), but also conforming the klines like a pandas dataframe, extended with market indicator methods.
from anansi.marketdata.handlers import KlinesFromBroker
BinanceKlines = KlinesFromBroker(
broker_name="binance", ticker_symbol="BTCUSDT", time_frame="1h")
newest_klines = BinanceKlines.newest(2167)
newest_klines
Open_time | Open | High | Low | Close | Volume | |
---|---|---|---|---|---|---|
0 | 2020-06-17 11:00:00 | 9483.25 | 9511.53 | 9466.00 | 9478.61 | 1251.802697 |
1 | 2020-06-17 12:00:00 | 9478.61 | 9510.88 | 9477.35 | 9499.25 | 1120.426332 |
2 | 2020-06-17 13:00:00 | 9499.24 | 9565.00 | 9432.00 | 9443.48 | 4401.693008 |
3 | 2020-06-17 14:00:00 | 9442.50 | 9464.83 | 9366.09 | 9410.95 | 4802.211120 |
4 | 2020-06-17 15:00:00 | 9411.27 | 9436.54 | 9388.43 | 9399.24 | 2077.135281 |
... | ... | ... | ... | ... | ... | ... |
2162 | 2020-09-15 13:00:00 | 10907.94 | 10917.96 | 10834.00 | 10834.71 | 3326.420940 |
2163 | 2020-09-15 14:00:00 | 10834.71 | 10879.00 | 10736.63 | 10764.19 | 4382.021477 |
2164 | 2020-09-15 15:00:00 | 10763.37 | 10815.47 | 10745.63 | 10784.46 | 3531.309654 |
2165 | 2020-09-15 16:00:00 | 10785.23 | 10827.61 | 10700.00 | 10784.23 | 3348.735166 |
2166 | 2020-09-15 17:00:00 | 10784.23 | 10812.44 | 10738.33 | 10794.84 | 1931.035921 |
2167 rows × 6 columns
indicator = newest_klines.apply_indicator.trend.simple_moving_average(number_of_candles=35)
indicator.name, indicator.last(), indicator.serie
('sma_ohlc4_35',
10669.49407142858,
0 NaN
1 NaN
2 NaN
3 NaN
4 NaN
...
2162 10619.190500
2163 10632.213571
2164 10644.682643
2165 10657.128857
2166 10669.494071
Length: 2167, dtype: float64)
newest_klines
Open_time | Open | High | Low | Close | Volume | |
---|---|---|---|---|---|---|
0 | 2020-06-17 11:00:00 | 9483.25 | 9511.53 | 9466.00 | 9478.61 | 1251.802697 |
1 | 2020-06-17 12:00:00 | 9478.61 | 9510.88 | 9477.35 | 9499.25 | 1120.426332 |
2 | 2020-06-17 13:00:00 | 9499.24 | 9565.00 | 9432.00 | 9443.48 | 4401.693008 |
3 | 2020-06-17 14:00:00 | 9442.50 | 9464.83 | 9366.09 | 9410.95 | 4802.211120 |
4 | 2020-06-17 15:00:00 | 9411.27 | 9436.54 | 9388.43 | 9399.24 | 2077.135281 |
... | ... | ... | ... | ... | ... | ... |
2162 | 2020-09-15 13:00:00 | 10907.94 | 10917.96 | 10834.00 | 10834.71 | 3326.420940 |
2163 | 2020-09-15 14:00:00 | 10834.71 | 10879.00 | 10736.63 | 10764.19 | 4382.021477 |
2164 | 2020-09-15 15:00:00 | 10763.37 | 10815.47 | 10745.63 | 10784.46 | 3531.309654 |
2165 | 2020-09-15 16:00:00 | 10785.23 | 10827.61 | 10700.00 | 10784.23 | 3348.735166 |
2166 | 2020-09-15 17:00:00 | 10784.23 | 10812.44 | 10738.33 | 10794.84 | 1931.035921 |
2167 rows × 6 columns
indicator = newest_klines.apply_indicator.trend.simple_moving_average(
number_of_candles=35, indicator_column="SMA_OHLC4_n35")
newest_klines
Open_time | Open | High | Low | Close | Volume | SMA_OHLC4_n35 | |
---|---|---|---|---|---|---|---|
0 | 2020-06-17 11:00:00 | 9483.25 | 9511.53 | 9466.00 | 9478.61 | 1251.802697 | NaN |
1 | 2020-06-17 12:00:00 | 9478.61 | 9510.88 | 9477.35 | 9499.25 | 1120.426332 | NaN |
2 | 2020-06-17 13:00:00 | 9499.24 | 9565.00 | 9432.00 | 9443.48 | 4401.693008 | NaN |
3 | 2020-06-17 14:00:00 | 9442.50 | 9464.83 | 9366.09 | 9410.95 | 4802.211120 | NaN |
4 | 2020-06-17 15:00:00 | 9411.27 | 9436.54 | 9388.43 | 9399.24 | 2077.135281 | NaN |
... | ... | ... | ... | ... | ... | ... | ... |
2162 | 2020-09-15 13:00:00 | 10907.94 | 10917.96 | 10834.00 | 10834.71 | 3326.420940 | 10619.190500 |
2163 | 2020-09-15 14:00:00 | 10834.71 | 10879.00 | 10736.63 | 10764.19 | 4382.021477 | 10632.213571 |
2164 | 2020-09-15 15:00:00 | 10763.37 | 10815.47 | 10745.63 | 10784.46 | 3531.309654 | 10644.682643 |
2165 | 2020-09-15 16:00:00 | 10785.23 | 10827.61 | 10700.00 | 10784.23 | 3348.735166 | 10657.128857 |
2166 | 2020-09-15 17:00:00 | 10784.23 | 10812.44 | 10738.33 | 10794.84 | 1931.035921 | 10669.494071 |
2167 rows × 7 columns
DISCLAIMER: Requests here are not queued! There is a risk of banning the IP or even blocking API keys if some limits are exceeded. Use with caution.
from anansi.marketdata import data_brokers
BinanceBroker = data_brokers.BinanceDataBroker()
my_klines = BinanceBroker.get_klines(ticker_symbol="BTCUSDT", time_frame="1m")
my_klines
Open_time | Open | High | Low | Close | Volume | |
---|---|---|---|---|---|---|
0 | 1600165560 | 10688.12 | 10691.14 | 10684.88 | 10684.88 | 21.529835 |
1 | 1600165620 | 10684.88 | 10686.15 | 10681.84 | 10685.99 | 18.487428 |
2 | 1600165680 | 10686.00 | 10687.65 | 10684.92 | 10687.09 | 22.246376 |
3 | 1600165740 | 10687.09 | 10689.54 | 10683.86 | 10687.26 | 18.818481 |
4 | 1600165800 | 10687.26 | 10687.26 | 10683.71 | 10685.76 | 38.281582 |
... | ... | ... | ... | ... | ... | ... |
494 | 1600195200 | 10762.43 | 10763.48 | 10760.35 | 10760.75 | 8.572210 |
495 | 1600195260 | 10760.75 | 10762.48 | 10759.30 | 10759.31 | 11.089815 |
496 | 1600195320 | 10759.30 | 10762.22 | 10755.39 | 10761.26 | 27.070820 |
497 | 1600195380 | 10761.26 | 10761.26 | 10751.74 | 10756.02 | 15.482246 |
498 | 1600195440 | 10755.61 | 10756.57 | 10748.03 | 10748.04 | 61.153777 |
499 rows × 6 columns
my_klines = BinanceBroker.get_klines(ticker_symbol="BTCUSDT", time_frame="1m", show_only_desired_info=False)
my_klines
Open_time | Open | High | Low | Close | Volume | Close_time | Quote_asset_volume | Number_of_trades | Taker_buy_base_asset_volume | Taker_buy_quote_asset_volume | Ignore | |
---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | 1600165560 | 10688.12 | 10691.14 | 10684.88 | 10684.88 | 21.529835 | 1600165619 | 230126.587773 | 373.0 | 10.279415 | 109864.149822 | 0.0 |
1 | 1600165620 | 10684.88 | 10686.15 | 10681.84 | 10685.99 | 18.487428 | 1600165679 | 197536.180849 | 336.0 | 8.256498 | 88223.566054 | 0.0 |
2 | 1600165680 | 10686.00 | 10687.65 | 10684.92 | 10687.09 | 22.246376 | 1600165739 | 237738.839831 | 415.0 | 13.378805 | 142975.243246 | 0.0 |
3 | 1600165740 | 10687.09 | 10689.54 | 10683.86 | 10687.26 | 18.818481 | 1600165799 | 201100.293663 | 539.0 | 9.062957 | 96849.611844 | 0.0 |
4 | 1600165800 | 10687.26 | 10687.26 | 10683.71 | 10685.76 | 38.281582 | 1600165859 | 409068.511314 | 534.0 | 16.799813 | 179523.708531 | 0.0 |
... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... |
494 | 1600195200 | 10762.43 | 10763.48 | 10760.35 | 10760.75 | 8.572210 | 1600195259 | 92253.016477 | 292.0 | 2.394778 | 25771.715413 | 0.0 |
495 | 1600195260 | 10760.75 | 10762.48 | 10759.30 | 10759.31 | 11.089815 | 1600195319 | 119341.014647 | 277.0 | 3.064458 | 32976.256534 | 0.0 |
496 | 1600195320 | 10759.30 | 10762.22 | 10755.39 | 10761.26 | 27.070820 | 1600195379 | 291245.877535 | 490.0 | 14.654896 | 157679.926758 | 0.0 |
497 | 1600195380 | 10761.26 | 10761.26 | 10751.74 | 10756.02 | 15.482246 | 1600195439 | 166520.446192 | 353.0 | 7.390407 | 79491.160961 | 0.0 |
498 | 1600195440 | 10755.61 | 10756.57 | 10748.03 | 10748.04 | 61.153777 | 1600195499 | 657520.935924 | 585.0 | 13.436657 | 144474.084684 | 0.0 |
499 rows × 12 columns
FAQs
A tool to analyze data and perform operations in markets
We found that anansi-toolkit demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.