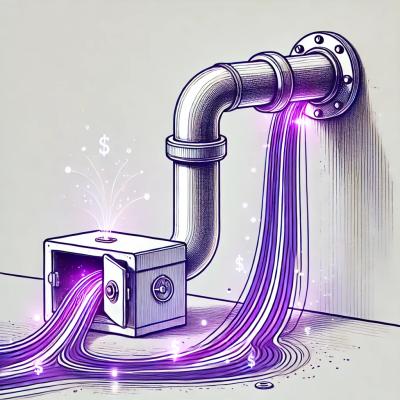
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
.. list-table:: :stub-columns: 1
.. |travis| image:: https://img.shields.io/travis/danilobellini/audiolazy/master.svg :target: https://travis-ci.org/danilobellini/audiolazy :alt: Travis CI builds
.. |coveralls| image:: https://img.shields.io/coveralls/danilobellini/audiolazy/master.svg :target: https://coveralls.io/r/danilobellini/audiolazy :alt: Coveralls coverage report
.. |v| image:: https://img.shields.io/pypi/v/audiolazy.svg :target: https://pypi.python.org/pypi/audiolazy :alt: Last stable version (PyPI)
.. |pyversions| image:: https://img.shields.io/pypi/pyversions/audiolazy.svg :target: https://pypi.python.org/pypi/audiolazy :alt: Python versions (PyPI)
.. |implementation| image:: https://img.shields.io/pypi/implementation/audiolazy.svg :target: https://pypi.python.org/pypi/audiolazy :alt: Python implementations (PyPI)
.. |format| image:: https://img.shields.io/pypi/format/audiolazy.svg :target: https://pypi.python.org/pypi/audiolazy :alt: Distribution format (PyPI)
.. |status| image:: https://img.shields.io/pypi/status/audiolazy.svg :target: https://pypi.python.org/pypi/audiolazy :alt: Project status (PyPI)
.. |l| image:: https://img.shields.io/pypi/l/audiolazy.svg :target: https://pypi.python.org/pypi/audiolazy :alt: License (PyPI)
There are several tools and packages that let the Python use and expressiveness look like languages such as MatLab and Octave. However, the eager evaluation done by most of these tools make it difficult, perhaps impossible, to use them for real time audio processing. To avoid such eagerness, one can make the calculations only when data is requested, not when the path to the data is given. This is the core idea in laziness that allows:
Another difficulty concerns expressive code creation for audio processing in blocks through indexes and vectors. Sometimes, that's unavoidable, or at least such avoidance would limit the power of the system that works with sequence data.
Block sequences can be found from sample sequences being both objects, where the latter can be the result of a method or function over the former. The information needed for such is the block size and where would start the next block. Although one can think about the last block and the exact index where it would start, most of the time spent in steps like this one happens to be an implementation issue that just keep the focus away from the problem being worked on. To allow a thing like an endless data sequence, there should be no need to know when something stops.
Probably an engineer would find the use of equations and structures from
electrical engineering theory much cleaner to understand than storing
everything into data arrays, mainly when common operations are done to these
representations. What is the product of the filter with numerator
[1, 7, 2]
and denominator [1, 0.5, 0.2]
as its system equation with
the one that has the arrays reversed like [2, 7, 1]
? That might be simple,
and the reversed would avoid questions like "what comes first, the zero or the
[minus] two exponent?", but maybe we could get more efficient ourselves if we
had something easier: multiplication could be written once and for all and
with a representation programmers are used to see. This would be even more
expressive if we could get rid from the asymmetry of a method call like
filt1.multiply_by(filt2)
, since multiplication in this case should be
commutative. The use of natural operators is possible in a language that
allows operator overloading, but for such we need to describe
those equations and structures as objects and object relationships.
The name Hz
can be a number that would allow conversion to a default DSP
internal rad/samples unit, so one can write things like freq = 440 * Hz
.
This isn't difficult in probably any language, but can help in expressiveness,
already. If (almost) everything would need data in "samples" or "rad/sample"
units, constants for converting these from "second" and "hertz" would help
with the code expressiveness. A comb filter comb.tau(delay=30*s, tau=40*s)
can represent a comb filter with the given delay and time constant, both in
samples, but with a more clear meaning for the reader than it would have with
an expression like [1] + [0] * 239999 + [alpha]
. Would it be needed to
store all those zeros while just using the filter to get a frequency response
plot?
It's possible to avoid some of these problems with well-chosen constants, duck typing, overloaded operators, functions as first-class citizens, object oriented together with functional style programming, etc.., resources that the Python language gives us for free.
Prioritizing code expressiveness, clarity and simplicity, without precluding the lazy evaluation, and aiming to be used together with Numpy, Scipy and Matplotlib as well as default Python structures like lists and generators, AudioLazy is a package written in pure Python proposing digital audio signal processing (DSP), featuring:
Stream
class for finite and endless signals representation with
elementwise operators (auto-broadcast with non-iterables) in a common
Python iterable container accepting heterogeneous data;Stream.blocks(size, hop)
method;ControlStream
;Streamix
mixer for iterables given their starting time deltas;filt = 1 / (1 - .3 * z ** -1)
), including linear time variant filters
(i.e., the a
in a * z ** k
can be a Stream instance), cascade
filters (behaves as a list of filters), resonators, etc.. Each
LinearFilter
instance is compiled just in time when called;ZFilter
instances, from
which you can find PARCOR coeffs and LSFs;StrategyDict
instances:
callable dictionaries that allows the same name to have several different
implementations (e.g. erb
, gammatone
, lowpass
, resonator
,
lpc
, window
);The package works both on Linux and on Windows. You can find the last stable
version at PyPI <http://pypi.python.org/pypi/audiolazy>
_ and install it with
the usual Python installing mechanism::
python setup.py install
If you have pip, you can go directly (use -U
for update or reinstall)::
pip install audiolazy
for downloading (from PyPI) and installing the package for you, or::
pip install -U .
To install from a path that has the setup.py
file and the package data
uncompressed previously.
For the bleeding-edge version, you can install directly from the github
repository (requires git
for cloning)::
pip install -U git+git://github.com/danilobellini/audiolazy.git
For older versions, you can install from the PyPI link or directly from the
github repository, based on the repository tags. For example, to install the
version 0.04 (requires git
for cloning)::
pip install -U git+git://github.com/danilobellini/audiolazy.git@v0.04
The package doesn't have any strong dependency for its core besides the Python itself (versions 2.7, 3.2 or newer) as well as its standard library, but you might need:
AudioIO
class);LinearFilter.plot
method and several examples;Beside examples and tests, only the filter plotting with plot
and
zplot
methods needs MatPlotLib. Also, the routines that needs NumPy up to
now are:
zeros
and poles
properties (filter classes) or
with roots
property (Poly class);lpc
) strategies: nautocor
,
autocor
and covar
;lsf
and lsf_stable
functions.Before all examples below, it's easier to get everything from audiolazy namespace:
.. code-block:: python
from audiolazy import *
All modules starts with "lazy_", but their data is already loaded in the main namespace. These two lines of code do the same thing:
.. code-block:: python
from audiolazy.lazy_stream import Stream from audiolazy import Stream
Endless iterables with operators (be careful with loops through an endless iterator!):
.. code-block:: python
a = Stream(2, -2, -1) # Periodic b = Stream(3, 7, 5, 4) # Periodic c = a + b # Elementwise sum, periodic c.take(15) # First 15 elements from the Stream object [5, 5, 4, 6, 1, 6, 7, 2, 2, 9, 3, 3, 5, 5, 4]
And also finite iterators (you can think on any Stream as a generator with elementwise operators):
.. code-block:: python
a = Stream([1, 2, 3, 2, 1]) # Finite, since it's a cast from an iterable b = Stream(3, 7, 5, 4) # Periodic c = a + b # Elementwise sum, finite list(c) [4, 9, 8, 6, 4]
LTI Filtering from system equations (Z-transform). After this, try summing, composing, multiplying ZFilter objects:
.. code-block:: python
filt = 1 - z ** -1 # Diff between a sample and the previous one filt 1 - z^-1 data = filt([.1, .2, .4, .3, .2, -.1, -.3, -.2]) # Past memory has 0.0 data # This should have internally [.1, .1, .2, -.1, -.1, -.3, -.2, .1] <audiolazy.lazy_stream.Stream object at ...> data *= 10 # Elementwise gain [int(round(x)) for x in data] # Streams are iterables [1, 1, 2, -1, -1, -3, -2, 1] data_int = filt([1, 2, 4, 3, 2, -1, -3, -2], zero=0) # Now zero is int list(data_int) [1, 1, 2, -1, -1, -3, -2, 1]
LTI Filter frequency response plot (needs MatPlotLib):
.. code-block:: python
(1 + z ** -2).plot().show()
.. image:: https://raw.github.com/danilobellini/audiolazy/master/images/filt_plot.png
The matplotlib.figure.Figure.show
method won't work unless you're
using a newer version of MatPlotLib (works on MatPlotLib 1.2.0), but you still
can save the above plot directly to a PDF, PNG, etc. with older versions
(e.g. MatPlotLib 1.0.1):
.. code-block:: python
(1 + z ** -2).plot().savefig("my_plot.pdf")
On the other hand, you can always show the figure using MatPlotLib directly:
.. code-block:: python
from matplotlib import pyplot as plt # Or "import pylab as plt" filt = 1 + z ** -2 fig1 = filt.plot(plt.figure()) # Argument not needed on the first figure fig2 = filt.zplot(plt.figure()) # The argument ensures a new figure plt.show()
CascadeFilter instances and ParallelFilter instances are lists of filters with the same operator behavior as a list, and also works for plotting linear filters. Constructors accepts both a filter and an iterable with filters. For example, a zeros and poles plot (needs MatPlotLib):
.. code-block:: python
filt1 = CascadeFilter(0.2 - z ** -3) # 3 zeros filt2 = CascadeFilter(1 / (1 -.8 * z ** -1 + .6 * z ** -2)) # 2 poles
filt = (filt1 * 5 + filt2 * 10) # 15 zeros and 20 poles filt.zplot().show()
.. image:: https://raw.github.com/danilobellini/audiolazy/master/images/cascade_plot.png
Linear Predictive Coding (LPC) autocorrelation method analysis filter frequency response plot (needs MatPlotLib):
.. code-block:: python
lpc([1, -2, 3, -4, -3, 2, -3, 2, 1], order=3).plot().show()
.. image:: https://raw.github.com/danilobellini/audiolazy/master/images/lpc_plot.png
Linear Predictive Coding covariance method analysis and synthesis filter, followed by the frequency response plot together with block data DFT (MatPlotLib):
.. code-block:: python
data = Stream(-1., 0., 1., 0.) # Periodic blk = data.take(200) analysis_filt = lpc.covar(blk, 4) analysis_filt 1 + 0.5 * z^-2 - 0.5 * z^-4 residual = list(analysis_filt(blk)) residual[:10] [-1.0, 0.0, 0.5, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0] synth_filt = 1 / analysis_filt synth_filt(residual).take(10) [-1.0, 0.0, 1.0, 0.0, -1.0, 0.0, 1.0, 0.0, -1.0, 0.0] amplified_blk = list(Stream(blk) * -200) # For alignment w/ DFT synth_filt.plot(blk=amplified_blk).show()
.. image:: https://raw.github.com/danilobellini/audiolazy/master/images/dft_lpc_plot.png
AudioLazy doesn't need any audio card to process audio, but needs PyAudio to play some sound:
.. code-block:: python
rate = 44100 # Sampling rate, in samples/second s, Hz = sHz(rate) # Seconds and hertz ms = 1e-3 * s note1 = karplus_strong(440 * Hz) # Pluck "digitar" synth note2 = zeros(300 * ms).append(karplus_strong(880 * Hz)) notes = (note1 + note2) * .5 sound = notes.take(int(2 * s)) # 2 seconds of a Karplus-Strong note with AudioIO(True) as player: # True means "wait for all sounds to stop" player.play(sound, rate=rate)
See also the docstrings and the "examples" directory at the github repository for more help. Also, the huge test suite might help you understanding how the package works and how to use it.
There are several files and directories in the AudioLazy repository (as well as in the source distribution):
================ ============================================================= File/Directory Description ================ ============================================================= audiolazy/ AudioLazy package modules source code audiolazy/tests/ Testing subpackage docs/ Documentation generation scripts examples/ Examples for some AudioLazy use cases images/ Images referenced by some reStructuredText documentation file math/ Proof for some formula used by AudioLazy using Sympy CAS CHANGES.rst AudioLazy History, a.k.a. change log conftest.py Configuration for py.test, to work properly with doctests on StrategyDict strategies and on an environment missing Numpy COPYING.txt License file MANIFEST.in List of extra distributed files to be included in the tarball that doesn't need to be installed together with the package README.rst Some general information about the AudioLazy project setup.py General Python setup script for installation, testing, etc. tox.ini Configuration for tox, py.test and pytest-cov .travis.yml Travis-CI configuration (not in PyPI tarball/"egg" source distribution) ================ =============================================================
The examples
and the math
directories might be useful for an AudioLazy
user. All Python files in these two directories are scripts intended to run on
both Python 2 and 3 unless they need something not yet available for Python 3
(e.g. wxPython), most of them have some external dependency.
*** Version 0.6 (Examples, STFT, phon2dB, tox, CI, wave file) ***
examples:
Formant synthesis for voiced "ah-eh-ee-oh-oo"
Musical keyboard synth example with a QWERTY keyboard (also via jack!)
Random synthesis with saving and memoization
Aesthetics for the Tkinter GUI examples
Matplotlib animated plot with mic input data (also works via jack!)
Perceptual experiment with butterworth filters (Scipy) applied to white noise (based on the Demonstrations to Auditory Scene Analysis)
Psychoacoustics using ISO/FDIS 226:2003
Partial recreation of the "Windows and Figures of Merit" F. Harris comparison table and window plots
Playing/synthesizing "Ode to Joy" from its "score" written as code
All recording/playback examples now prepared for using both the default API and receiving it as an argument like "jack" or "alsa"
Realtime STFT (Short Time Fourier Transform) effects:
general:
_internals.py
module to avoid exposing package internals together
with the API, which also allowed the new simplified __init__.py
test
command now calls tox
)conftest.py
for testing doctests from strategies inside
StrategyDict instances without the need of a __test__
in the module
and for skipping doctests known to need Numpy when it's not availablemath
directory for adding scripts with symbolic math calculations
(with Sympy) done for designing (or as a proof) for parts of the
AudioLazy code. All lowpass and highpass strategies have their design
explicitly explained therelazy_analysis:
New overlap_add
StrategyDict instance, allowing resynth after block
processing/analysis, featuring block size auto-detection and window
normalization to keep the output in the [-1; 1] range. Has 2
implementations keeping the same interface:
numpy
(default): needs Numpy arrays internallylist
: uses lists instead, doesn't need Numpy and was tested on PypyNew stft
StrategyDict instance, allowing Short Time Fourier Transform
block processors / phase vocoder by:
stft
strategies as a decoratorIt was created with three Numpy-based strategies:
rfft
, real
or base
(default): needs numpy.fft.rfft
internally, as well as its inverse, to process a block in the
frequency domain with values up to the Nyquist frequency, by assuming
the input signal is realcfft
or complex
: Alike to the default approach but uses the
numpy.fft.fft
for a full complex-valued block in frequency domain,
which means the output is a complex signalcfftr
or complex_real
: same to stft.cfft
strategy, but
keeps only the real part of the resultParameters allows changing the default zero-phase behavior (before
and after
functions), the transform and inverse transform functions,
the overlap-add strategy (as well as its parameters), and obviously the
block size and hop
The window
StrategyDict now returns the "periodic" window data
instead of the "symmetric" window to be used with the STFT directly
New wsymm
StrategyDict with the same strategies from window
but
returning a "symmetric" window
Default window
strategy is the Hann window (the same for wsymm
)
New cos
strategy for window
and wsymm
implementing cosine to
the power of alpha
lazy_auditory:
phon2dB
StrategyDict instance with the ISO/FDIS 226:2003 model
for getting a SPL (Sound Pressure Level) in dBs from a phon valuelazy_core:
@
matrix multiplication operatorOpMethod.get()
now accepts numbers "1"
and "2"
as strings for
unary and binary query for operators, and "all"
is the new default, so
OpMethod.get(without="2 ~")
would get all operators but the [reversed
or not] binary ones and the invert operator, which means it would yield
only the unary "+"
(pos) and -
(neg), as probably was expected;
OpMethod also had a bug fix regarding the shift operatorsvars(window)
) instead of
depending on the __getattr__
methodStrategyDict.strategy
method now allows the strategy function name to
be kept by using the new keep_name
keyword-only argument. It might be
helpful for adding built-in functions as well as other immutable
callables as multi-name strategies with the same behavior as the item
assignment for adding a strategykey2keys
and value2keys
to help getting a
tuple with all keys that points to the same value, ordered by the
insertion orderlazy_filters:
LinearFilter coefficients can now be a Stream of Numpy matrices, as well as Sympy symbols (including symbolic matrices).
New simple lowpass/highpass IIR filter strategies:
highpass.z
(taken as the new highpass
default)lowpass.z
highpass.pole_exp
highpass.z_exp
lowpass.z_exp
Where the "z"
name denotes the presence of a single zero (besides the
single pole) and "_exp"
denotes the exponential approximation from
a matched Z-Transform pole value from the equivalent or mirrored analog
filter equation (Laplace). The absence of such suffix denotes it was
designed directly as a digital filter.
lazy_io:
api
keyword argument for AudioIO, allowing e.g. integration with
JACK (for now this needs chunks.size = 1
)AudioIO.open
and AudioIO.record
now allows keyword arguments, to
be passed directly to PyAudio, including the now default "channels"
keyword (old "nchannels" keyword still works, for backward compatibility)lazy_math:
sign
now works on Python 3lazy_misc:
cached
decorator caching the results for a function without
keyword argumentslazy_poly:
sort
and a reverse
keyword arguments to choose the yielding order (sorted by powers, keep
creation order or reverse any of these)lazy_text:
format_docstring
decorator that use format-style templates to
assign docstrings to functions, intended to avoid docstring copies on
StrategyDict instances.lazy_wav (new!):
WavStream
class for opening Wave files. Can load 24-bit audio files!
Tested with 8 bits files (unsigned int) and 16/24/32 bits (signed int)
mono and stereo files, both casting the data to a float in [-1;1) interval
(default) and keeping the original integer data as it ismultiple modules:
abs
to
absolute
, so no Python built-in name is ever replaced when importing
with from audiolazy import *
. Also, the built-in abs
now can be
used directly with Stream instancesfreq2lag
and lag2freq
to
use 2
instead of _to_
, and moved them to lazy_misc*** Version 0.05 (Python 2 & 3, more examples, refactoring, polinomials) ***
examples:
general:
envelope.abs
, midi2str
, StreamTeeHub.blocks
, etc.)inf
and nan
Stream.tee()
methodDEFAULT_CHUNK_SIZE
and LATEX_PI_SYMBOL
were removed:
the default values are now changeable and inside chunks
and
float_str
, respectively (see docstrings for more details)__div__
and __truediv__
(Python 2.7)lazy_compat (new!):
xmap
, xfilter
, xzip
,
xrange
, xzip_longest
. Versions with "i" are kept in lazy_itertools
module to return Stream instances (imap
, izip
, izip.longest
,
etc.), and Python 2 list-based behaviour of range
is kept as
orange
(a fruitful name)meta
function for creating metaclasses always in a "Python 3
look-alike" style, keeping the semantics (including the inheritance
hierarchy, which won't have any extra "dummy" class)lazy_core:
OpMethod
class with 33 operator method instances and queryingAbstractOperatorOverloaderMeta
to the new OpMethod-based
interface__test__
so that doctests from
strategies are found by the doctest finder.lazy_filters:
lazy_io:
stop
methodlazy_itertools:
accumulate
itertool from Python 3, available also in Python 2
yielding a Stream. This is a new StrategyDict with one more strategy in
Python 3chain.from_iterable
is now available (Stream version
itertool), and chain
is now a StrategyDictizip
is a StrategyDict, with izip.smallest
(default) and
izip.longest
strategieslazy_misc:
rint
for "round integer" operations as well as other higher step
integer quantizationalmost_eq
is a single StrategyDict with both bits
(default,
comparison by significand/mantissa bits) and diff
(absolute value
difference) strategieslazy_poly:
x
Poly object (to be used like the z
ZFilter instance)Poly.is_polynomial()
and Poly.is_laurent()
Poly.order
for common polynomialsPoly.integrate()
and Poly.diff()
methods returns Poly
instances, and the zero
from the caller Poly is always kept in
result (this includes many bugfixes)Poly.copy()
methodlazy_stream:
sHz
output as
s
(for second) you can now use my_stream.take(20 * s)
directly,
as well as a "take all" feature my_stream.take(inf)
Stream.peek()
method, allowing taking items while keeping them
as the next to be yielded by the Stream or StreamTeeHubStream.skip()
method for neglecting the leading items without
storing themStream.limit()
method, to enforce a maximum "length"skip()
, limit()
, append()
, map()
and
filter()
returns the modified copy as a Stream instance (i.e., works
like Stream(my_stream_tee_hub).method_name()
)tostream
(needed for lazy_itertools)lazy_synth:
ones()
, zeros()
, white_noise()
and
impulse()
now can be inf (besides None)one=1.
and zero=0.
argumentsgauss_noise
for Normal / Gaussian-distributed noiselazy_text (new!):
float_str
embraces older
rational/pi/auto formatters in one instance*** Version 0.04 (Documentation, LPC, Plots!) ***
examples:
general:
lazy_analysis:
acorr
and lag
"covariance" (due to lpc.covar) with lag_matrix
2 * pi
radians range but configurable
to other units and max signal difference allowedlazy_core:
lazy_filters:
ZFilter composition/substitution, e.g., (1 + z ** -1)(1 / z)
results
to the ZFilter instance 1 + z
New LinearFilter.plot() directly plots the frequency response of a LTI filter to a MatPlotLib figure. Configurable:
pi
, as well as the formatterNew LinearFilter.zplot() for plotting the zero-pole plane of a LTI filter directly into a MatPlotLib figure
New LinearFilterProperties read-only properties numpolyz
and
denpolyz
returning polynomials based on x = z
instead of the
polynomials based on x = z ** -1
returned from numpoly
and
denpoly
New LinearFilter properties poles
and zeros
, based on NumPy
New class FilterList
for filter grouping with a callables
property, for casting from lists with constant gain values as filters.
It is an instance of FilterListMeta
(old CascadeFilterMeta), and
CascadeFilter now inherits from this FilterList
More LinearFilter behaviour into FilterList: Plotting (plot
and
zplot
), poles
, zeros
, is_lti
and is_causal
New ParallelFilter
class, inheriting from FilterList
Now comb is a StrategyDict too, with 3 strategies:
comb.fb
(default): Feedback comb filter (IIR or time variant)comb.tau
: Same to the feedback strategy, but with a time decay
tau
parameter (time in samples up to 1/e
amplitude, or
-8.686 dB) instead of a gain alpha
comb.ff
: Feed-forward comb filter (FIR or time variant)lazy_lpc (new!):
Linear Predictive Coding (LPC) coefficients as a ZFilter from:
lpc.autocor
(default): Auto-selects autocorrelation implementation
(Faster)lpc.nautocor
: Autocorrelation, with linear system solved by NumPy
(Safer)lpc.kautocor
: Autocorrelation, using the Levinson-Durbin algorithmlpc.covar
or lpc.ncovar
: Covariance, with linear system solved
by NumPylpc.kcovar
: Covariance, slower. Mainly for those without NumPylevinson_durbin
: Same to the lpc.kautocor
, but with the
autocorrelation vector as the input, not the signal dataToeplitz matrix as a list of lists
Partial correlation coefficients (PARCOR) or reflection coefficients
Line Spectral Frequencies (LSF)
Stability testers for filters with LSF and PARCOR
lazy_math:
sign
gets the sign of a given sequence.lazy_midi:
octaves
for finding all octaves in a frequency range given one
frequencylazy_misc:
rational_formatter
: casts floats to strings, perhaps with a symbol
string as multiplierpi_formatter
: same to rational_formatter
, but with the symbol
fixed to pi, mainly for use in MatPlotLib labelslazy_poly:
lazy_stream:
lazy_synth:
tau
time decay constant instead of
the comb filter alpha
gain.*** Version 0.03 (Time variant filters, examples, etc.. Major changes!) ***
examples (new!):
general:
lazy_analysis (new!):
New window
StrategyDict instance, with:
lazy_auditory (new!):
lazy_core:
lazy_filters:
LTI and LTIFreq no longer exists! They were renamed to LinearFilter and ZFilter since filters now can have Streams as coefficients (they don't need to be "Time Invariant" anymore)
Linear filters are now iterables, allowing:
assert almost_eq(filt1, filt2)
numerator_data, denominator_data = filt
, where
each data is a list of pairs that can be used as input for Poly,
LinearFilter or ZFilterLinearFilterProperties class, implementing numlist, denlist, numdict and dendict, besides numerator and denominator, from numpoly and denpoly
Comparison "==" and "!=" are now strict
CascadeFilter: list of filters that behave as a filter
LinearFilter.call now has the "zero" optional argument (allows non-float)
LinearFilter.call memory input can be a function or a Stream
LinearFilter.linearize: linear interpolated delay-line from fractional delays
Feedback comb filter
4 resonator filter models with 2-poles with exponential approximation for finding the radius from the bandwidth
Simple one pole lowpass and highpass filters
lazy_io:
lazy_itertools:
lazy_math (new!):
sin
, cos
, sqrt
, etc.), and the constants e
and pi
factorial
, ln
(the log
from math), log2
,
cexp
(the exp
from cmath) and phase
(from cmath)lazy_midi:
lazy_misc:
lazy_poly:
lazy_stream:
my_stream.imag
and my_stream.conjugate()
in a
stream with complex numberslazy_synth:
*** Version 0.02 (Interactive Stream objects & Table lookup synthesis!) ***
general:
lazy_midi (new!):
lazy_misc:
lazy_stream:
lazy_synth (new!):
*** Version 0.01 (First "pre-alpha" version!) ***
general:
lazy_core:
lazy_filters:
z
object, e.g.
filt = 1 / (.5 + z ** -1)
lazy_io:
lazy_itertools:
lazy_misc:
lazy_poly:
lazy_stream:
tostream
so generator functions can return Stream objectsFAQs
Real-Time Expressive Digital Signal Processing (DSP) Package for Python!
We found that audiolazy demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.