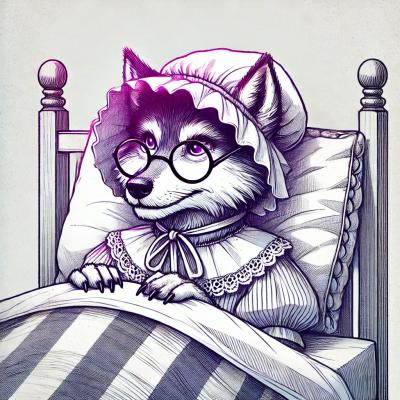
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Azure core provides shared exceptions and modules for Python SDK client libraries. These libraries follow the Azure SDK Design Guidelines for Python .
If you are a client library developer, please reference client library developer reference for more information.
Source code | Package (Pypi) | Package (Conda) | API reference documentation
Typically, you will not need to install azure core; it will be installed when you install one of the client libraries using it. In case you want to install it explicitly (to implement your own client library, for example), you can find it here.
AzureError is the base exception for all errors.
class AzureError(Exception):
def __init__(self, message, *args, **kwargs):
self.inner_exception = kwargs.get("error")
self.exc_type, self.exc_value, self.exc_traceback = sys.exc_info()
self.exc_type = self.exc_type.__name__ if self.exc_type else type(self.inner_exception)
self.exc_msg = "{}, {}: {}".format(message, self.exc_type, self.exc_value) # type: ignore
self.message = str(message)
self.continuation_token = kwargs.get("continuation_token")
super(AzureError, self).__init__(self.message, *args)
message is any message (str) to be associated with the exception.
args are any additional args to be included with exception.
kwargs are keyword arguments to include with the exception. Use the keyword error to pass in an internal exception and continuation_token for a token reference to continue an incomplete operation.
The following exceptions inherit from AzureError:
An error occurred while attempt to make a request to the service. No request was sent.
The request was sent, but the client failed to understand the response. The connection may have timed out. These errors can be retried for idempotent or safe operations.
A request was made, and a non-success status code was received from the service.
class HttpResponseError(AzureError):
def __init__(self, message=None, response=None, **kwargs):
self.reason = None
self.response = response
if response:
self.reason = response.reason
self.status_code = response.status_code
self.error = self._parse_odata_body(ODataV4Format, response) # type: Optional[ODataV4Format]
if self.error:
message = str(self.error)
else:
message = message or "Operation returned an invalid status '{}'".format(
self.reason
)
super(HttpResponseError, self).__init__(message=message, **kwargs)
message is the HTTP response error message (optional)
response is the HTTP response (optional).
kwargs are keyword arguments to include with the exception.
The following exceptions inherit from HttpResponseError:
An error raised during response de-serialization.
An error raised if peer closes the connection before we have received the complete message body.
An error response with status code 4xx. This will not be raised directly by the Azure core pipeline.
An error response, typically triggered by a 412 response (for update) or 404 (for get/post).
An error response with status code 4xx, typically 412 Conflict. This will not be raised directly by the Azure core pipeline.
An error response with status code 304. This will not be raised directly by the Azure core pipeline.
An error response with status code 4xx. This will not be raised directly by the Azure core pipeline.
An error raised when the maximum number of redirect attempts is reached. The maximum amount of redirects can be configured in the RedirectPolicy.
class TooManyRedirectsError(HttpResponseError):
def __init__(self, history, *args, **kwargs):
self.history = history
message = "Reached maximum redirect attempts."
super(TooManyRedirectsError, self).__init__(message, *args, **kwargs)
history is used to document the requests/responses that resulted in redirected requests.
args are any additional args to be included with exception.
kwargs are keyword arguments to include with the exception.
An error thrown if you try to access the stream of azure.core.rest.HttpResponse
or azure.core.rest.AsyncHttpResponse
once
the response stream has been consumed.
An error thrown if you try to access the stream of the azure.core.rest.HttpResponse
or azure.core.rest.AsyncHttpResponse
once
the response stream has been closed.
An error thrown if you try to access the content
of azure.core.rest.HttpResponse
or azure.core.rest.AsyncHttpResponse
before
reading in the response's bytes first.
When calling the methods, some properties can be configured by passing in as kwargs arguments.
Parameters | Description |
---|---|
headers | The HTTP Request headers. |
request_id | The request id to be added into header. |
user_agent | If specified, this will be added in front of the user agent string. |
logging_enable | Use to enable per operation. Defaults to False . |
logger | If specified, it will be used to log information. |
response_encoding | The encoding to use if known for this service (will disable auto-detection). |
raw_request_hook | Callback function. Will be invoked on request. |
raw_response_hook | Callback function. Will be invoked on response. |
network_span_namer | A callable to customize the span name. |
tracing_attributes | Attributes to set on all created spans. |
permit_redirects | Whether the client allows redirects. Defaults to True . |
redirect_max | The maximum allowed redirects. Defaults to 30 . |
retry_total | Total number of retries to allow. Takes precedence over other counts. Default value is 10 . |
retry_connect | How many connection-related errors to retry on. These are errors raised before the request is sent to the remote server, which we assume has not triggered the server to process the request. Default value is 3 . |
retry_read | How many times to retry on read errors. These errors are raised after the request was sent to the server, so the request may have side-effects. Default value is 3 . |
retry_status | How many times to retry on bad status codes. Default value is 3 . |
retry_backoff_factor | A backoff factor to apply between attempts after the second try (most errors are resolved immediately by a second try without a delay). Retry policy will sleep for: {backoff factor} * (2 ** ({number of total retries} - 1)) seconds. If the backoff_factor is 0.1, then the retry will sleep for [0.0s, 0.2s, 0.4s, ...] between retries. The default value is 0.8 . |
retry_backoff_max | The maximum back off time. Default value is 120 seconds (2 minutes). |
retry_mode | Fixed or exponential delay between attempts, default is Exponential . |
timeout | Timeout setting for the operation in seconds, default is 604800 s (7 days). |
connection_timeout | A single float in seconds for the connection timeout. Defaults to 300 seconds. |
read_timeout | A single float in seconds for the read timeout. Defaults to 300 seconds. |
connection_verify | SSL certificate verification. Enabled by default. Set to False to disable, alternatively can be set to the path to a CA_BUNDLE file or directory with certificates of trusted CAs. |
connection_cert | Client-side certificates. You can specify a local cert to use as client side certificate, as a single file (containing the private key and the certificate) or as a tuple of both files' paths. |
proxies | Dictionary mapping protocol or protocol and hostname to the URL of the proxy. |
cookies | Dict or CookieJar object to send with the Request . |
connection_data_block_size | The block size of data sent over the connection. Defaults to 4096 bytes. |
The async transport is designed to be opt-in. AioHttp is one of the supported implementations of async transport. It is not installed by default. You need to install it separately.
MatchConditions is an enum to describe match conditions.
class MatchConditions(Enum):
Unconditionally = 1 # Matches any condition
IfNotModified = 2 # If the target object is not modified. Usually it maps to etag=<specific etag>
IfModified = 3 # Only if the target object is modified. Usually it maps to etag!=<specific etag>
IfPresent = 4 # If the target object exists. Usually it maps to etag='*'
IfMissing = 5 # If the target object does not exist. Usually it maps to etag!='*'
A metaclass to support case-insensitive enums.
from enum import Enum
from azure.core import CaseInsensitiveEnumMeta
class MyCustomEnum(str, Enum, metaclass=CaseInsensitiveEnumMeta):
FOO = 'foo'
BAR = 'bar'
A falsy sentinel object which is supposed to be used to specify attributes
with no data. This gets serialized to null
on the wire.
from azure.core.serialization import NULL
assert bool(NULL) is False
foo = Foo(
attr=NULL
)
This project welcomes contributions and suggestions. Most contributions require you to agree to a Contributor License Agreement (CLA) declaring that you have the right to, and actually do, grant us the rights to use your contribution. For details, visit https://cla.microsoft.com.
When you submit a pull request, a CLA-bot will automatically determine whether you need to provide a CLA and decorate the PR appropriately (e.g., label, comment). Simply follow the instructions provided by the bot. You will only need to do this once across all repos using our CLA.
This project has adopted the Microsoft Open Source Code of Conduct. For more information, see the Code of Conduct FAQ or contact opencode@microsoft.com with any additional questions or comments.
BearerTokenCredentialPolicy
and AsyncBearerTokenCredentialPolicy
.tracing_attributes
keyword argument wasn't being handled at the request/method level. #38164HttpLoggingPolicy
.SupportsTokenInfo
and AsyncSupportsTokenInfo
, to offer more extensibility in supporting various token acquisition scenarios. #36565
get_token_info
method that returns an AccessTokenInfo
object.TokenRequestOptions
class, which is a TypedDict
with optional parameters, that can be used to define options for token requests through the get_token_info
method. #36565AccessTokenInfo
class, which is returned by get_token_info
implementations. This class contains the token, its expiration time, and optional additional information like when a token should be refreshed. #36565BearerTokenCredentialPolicy
and AsyncBearerTokenCredentialPolicy
now first check if a credential has the get_token_info
method defined. If so, the get_token_info
method is used to acquire a token. Otherwise, the get_token
method is used. #36565
refresh_on
attribute when determining if a new token request should be made.opentelemetry
is imported, then OpenTelemetry will be used to trace Azure SDK operations. #35050DistributedTracingPolicy
will now set an attribute, http.request.resend_count
, on HTTP spans for resent requests to indicate the resend attempt number. #35069error.type
attribute if an error status code is returned. #34619retry_after
header. #34203azure.core.rest.HttpRequest
#33948files
with duplicate field names azure.core.rest.HttpRequest
#34021anyio
. #33282AsyncBearerTokenCredentialPolicy
to work properly with trio
concurrency mechanisms. (#33307)anyio
>=3.0,<5.0requests
to 2.21.0.multipart/form-data
in the async transport where data
was not getting encoded into the request body. #32473message
cannot be None
in AzureError
. #31564AsyncTokenCredential.__aexit__()
#31573typing-extensions
version to 4.6.0.enabled_cae
unless it is explicitly enabled.enable_cae
was added to the get_token
method of the TokenCredential
protocol. #31012BearerTokenCredentialPolicy
and AsyncBearerTokenCredentialPolicy
now accept enable_cae
keyword arguments in their constructors. This is used in determining if Continuous Access Evaluation (CAE) should be enabled for each get_token
request. #31012RequestIdPolicy
. #30772SensitiveHeaderCleanupPolicy
that cleans up sensitive headers if a redirect happens and the new destination is in another domain. #28349AsyncBearerTokenCredentialPolicy
. #30381TokenCredential
protocol definitions #25187Azure-core is supported on Python 3.7 or later. For more details, please read our page on Azure SDK for Python version support policy.
CaseInsensitiveDict
implementation in azure.core.utils
removing dependency on requests
and aiohttp
ContentDecodePolicy
can now correctly deserialize more JSON bodies with different mime types #22410azure.core.paging.ItemPaged
#24548SerializationError
and DeserializationError
in azure.core.exceptions
for errors raised during serialization / deserialization #24312content
kwarg of azure.core.rest.HttpRequest
from objects with a read
method #23578Improve intellisense type hinting for service client methods. #22891
Add a case insensitive dict case_insensitive_dict
in azure.core.utils
. #23206
HttpLoggingPolicy
#22990typing-extensions
>= 4.0.1final-state-via
scope to POST until consuming SDKs has been fixed to use this option properly on PUT. #22989[This version is deprecated.]
final-state-via
LRO option in core. #22713HttpResponseError
if we're not able to parse out information #22302AttributeError
when calling azure.core.pipeline.transport.__bases__ #22469azure.core.exceptions.DecodeError
rather than requests.exceptions.ContentDecodingError
HttpResponseError
if we're not able to parse out information #21800send_request
onto the azure.core.PipelineClient
and azure.core.AsyncPipelineClient
. This method takes in
requests and sends them through our pipelines.azure.core.rest
. azure.core.rest
is our new public simple HTTP library in azure.core
that users will use to create requests, and consume responses.StreamConsumedError
, StreamClosedError
, and ResponseNotReadError
to azure.core.exceptions
. These errors
are thrown if you mishandle streamed responses from the azure.core.rest
moduleiter_raw
and iter_bytes
#21529json
kwarg in HttpRequest
#21504IncompleteReadError
which is raised if peer closes the connection before we have received the complete message body.Content-Length
header in a http response is strictly checked against the actual number of bytes in the body,
rather than silently truncating data in case the underlying tcp connection is closed prematurely.
(thanks to @jochen-ott-by for the contribution) #20412text/plain
and content length header for users who pass unicode strings to the content
kwarg of HttpRequest
in 2.7 #21550ContentDecodePolicy
#21318data
input to azure.core.rest
for python 2.7 users #21341charset_normalizer
if chardet
is not installed to migrate aiohttp 3.8.0 changes.azure.core.rest
packageazure.core.rest.HttpResponse
and azure.core.rest.AsyncHttpResponse
are now abstract base classes. They should not be initialized directly, instead
your transport responses should inherit from them and implement them.
The properties of the azure.core.rest
responses are now all read-only
HttpLoggingPolicy integrates logs into one record #19925
azure.core.serialization.AzureJSONEncoder
(introduced in 1.17.0) serializes datetime.datetime
objects in ISO 8601 format, conforming to RFC 3339's specification. #20190azure.core.serialization.AzureJSONEncoder
to serialize json
input to azure.core.rest.HttpRequest
.azure.core.rest
packagetext
property on azure.core.rest.HttpResponse
and azure.core.rest.AsyncHttpResponse
has changed to a method, which also takes
an encoding
parameter.iter_text
and iter_lines
from azure.core.rest.HttpResponse
and azure.core.rest.AsyncHttpResponse
azure.core.rest
responses now aligns across sync and async. Items can now be checked case-insensitively and without raising an error for format.from_json
method which now accepts storage QueueMessage, eventhub's EventData or ServiceBusMessage or simply json bytes to return a CloudEvent
azure.core.rest
packageazure.core.rest
will not try to guess the charset
anymore if it was impossible to extract it from HttpResponse
analysis. This removes our dependency on charset
.send_request
onto the azure.core.PipelineClient
and azure.core.AsyncPipelineClient
. This method takes in
requests and sends them through our pipelines.azure.core.rest
. azure.core.rest
is our new public simple HTTP library in azure.core
that users will use to create requests, and consume responses.StreamConsumedError
, StreamClosedError
, and ResponseNotReadError
to azure.core.exceptions
. These errors
are thrown if you mishandle streamed responses from the provisional azure.core.rest
modulefrom_dict
method of CloudEvent
when a wrong schema is sent.BearerTokenCredentialPolicy.on_challenge
and .authorize_request
to allow subclasses to optionally handle authentication challengesfrom_dict
methhod in the CloudEvent
can now convert a datetime string to datetime object when microsecond exceeds the python limitationazure.core.credentials.AzureNamedKeyCredential
credential #17548.decompress
parameter for stream_download
method. If it is set to False
, will not do decompression upon the stream. #17920Azure core requires Python 2.7 or Python 3.6+ since this release.
azure.core.utils.parse_connection_string
function to parse connection strings across SDKs, with common validation and support for case insensitive keys.~azure.core.tracing.Link
that should be used while passing Links
to AbstractSpan
.AbstractSpan
constructor can now take in additional keyword only args.This version will be the last version to officially support Python 3.5, future versions will require Python 2.7 or Python 3.6+.
azure.core.messaging.CloudEvent
model that follows the cloud event spec.azure.core.serialization.NULL
sentinel valuerepr
s for HttpRequest
and HttpResponse
s #16972CaseInsensitiveEnumMeta
class for case-insensitive enums. #16316raise_for_status
method onto HttpResponse
. Calling response.raise_for_status()
on a response with an error code
will raise an HttpResponseError
. Calling it on a good response will do nothing #16399AzureSasCredential
and its respective policy. #15946continuation_token
attribute to the base AzureError
exception, and set this value for errors raised
during paged or long-running operations.AzureKeyCredentialPolicy
will now accept (and ignore) passed in kwargs #11963http_logging_policy
property on the Configuration
object, allowing users to individually
set the http logging policy of the config #12218link
and link_from_headers
now accepts attributes #10765AzureKeyCredential
and its respective policy. #10509azure.core.polling.base_polling
module with a "Microsoft One API" polling implementation #10090
Also contains the async version in azure.core.polling.async_base_polling
enforce_https
to disable HTTPS check on authentication #9821HttpRequest.set_multipart_mixed
that will be passed into pipeline context.__enter__
and __exit__
from async context managers #9313Tracing: network span context is available with the TRACING_CONTEXT in pipeline response #7252
Tracing: Span contract now has kind
, traceparent
and is a context manager #7252
SansIOHTTPPolicy methods can now be coroutines #7497
Add multipart/mixed support #7083:
ServiceRequestError
#7542Tracing: azure.core.tracing.context
removed
Tracing: azure.core.tracing.context.tracing_context.with_current_context
renamed to azure.core.tracing.common.with_current_context
#7252
Tracing: link
renamed link_from_headers
and link
takes now a string
Tracing: opencensus implementation has been moved to the package azure-core-tracing-opencensus
Some modules and classes that were importables from several different places have been removed:
azure.core.HttpResponseError
is now only azure.core.exceptions.HttpResponseError
azure.core.Configuration
is now only azure.core.configuration.Configuration
azure.core.HttpRequest
is now only azure.core.pipeline.transport.HttpRequest
azure.core.version
module has been removed. Use azure.core.__version__
to get version number.azure.core.pipeline_client
has been removed. Import from azure.core
instead.azure.core.pipeline_client_async
has been removed. Import from azure.core
instead.azure.core.pipeline.base
has been removed. Import from azure.core.pipeline
instead.azure.core.pipeline.base_async
has been removed. Import from azure.core.pipeline
instead.azure.core.pipeline.policies.base
has been removed. Import from azure.core.pipeline.policies
instead.azure.core.pipeline.policies.base_async
has been removed. Import from azure.core.pipeline.policies
instead.azure.core.pipeline.policies.authentication
has been removed. Import from azure.core.pipeline.policies
instead.azure.core.pipeline.policies.authentication_async
has been removed. Import from azure.core.pipeline.policies
instead.azure.core.pipeline.policies.custom_hook
has been removed. Import from azure.core.pipeline.policies
instead.azure.core.pipeline.policies.redirect
has been removed. Import from azure.core.pipeline.policies
instead.azure.core.pipeline.policies.redirect_async
has been removed. Import from azure.core.pipeline.policies
instead.azure.core.pipeline.policies.retry
has been removed. Import from azure.core.pipeline.policies
instead.azure.core.pipeline.policies.retry_async
has been removed. Import from azure.core.pipeline.policies
instead.azure.core.pipeline.policies.distributed_tracing
has been removed. Import from azure.core.pipeline.policies
instead.azure.core.pipeline.policies.universal
has been removed. Import from azure.core.pipeline.policies
instead.azure.core.tracing.abstract_span
has been removed. Import from azure.core.tracing
instead.azure.core.pipeline.transport.base
has been removed. Import from azure.core.pipeline.transport
instead.azure.core.pipeline.transport.base_async
has been removed. Import from azure.core.pipeline.transport
instead.azure.core.pipeline.transport.requests_basic
has been removed. Import from azure.core.pipeline.transport
instead.azure.core.pipeline.transport.requests_asyncio
has been removed. Import from azure.core.pipeline.transport
instead.azure.core.pipeline.transport.requests_trio
has been removed. Import from azure.core.pipeline.transport
instead.azure.core.pipeline.transport.aiohttp
has been removed. Import from azure.core.pipeline.transport
instead.azure.core.polling.poller
has been removed. Import from azure.core.polling
instead.azure.core.polling.async_poller
has been removed. Import from azure.core.polling
instead.config
parameter anymore (use kwargs instead) #6372azure.core.paging
has been completely refactored #6420StreamDownloadGenerator
subclasses, response
is now an HttpResponse
, and not a transport response like aiohttp.ClientResponse
or requests.Response
. The transport response is available in internal_response
attribute #6490AsyncioRequestsTransport.sleep
is now a coroutine as expected #6490RequestsTransport
is not tight to ProxyPolicy
implementation details anymore #6372AiohttpTransport
does not raise on unexpected kwargs #6355continuation_token
and by_page()
#6420AiohttpTransport
#6372FAQs
Microsoft Azure Core Library for Python
We found that azure-core demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.