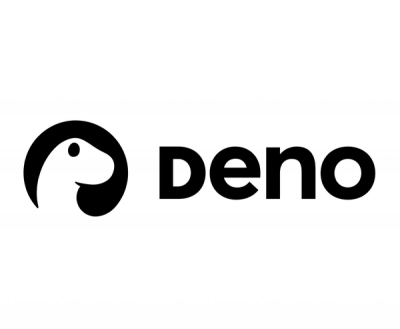
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
The Python reference implementation of BLEST (Batch-able, Lightweight, Encrypted State Transfer), an improved communication protocol for web APIs which leverages JSON, supports request batching by default, and provides a modern alternative to REST.
The Python reference implementation of BLEST (Batch-able, Lightweight, Encrypted State Transfer), an improved communication protocol for web APIs which leverages JSON, supports request batching by default, and provides a modern alternative to REST. It includes examples for Django, FastAPI, and Flask.
To learn more about BLEST, please visit the website: https://blest.jhunt.dev
For a front-end implementation in React, please visit https://github.com/jhuntdev/blest-react
Install BLEST Python from PyPI.
python3 -m pip install blest
The following example uses Flask, but you can find examples with other frameworks here.
from flask import Flask, make_response, request
from blest import Router
# Instantiate the Router
router = Router({ 'timeout': 1000 })
# Create some middleware (optional)
@router.before_request
async def user_middleware(body, context):
context['user'] = {
# user info for example
}
return
# Create a route controller
@router.route('greet')
async def greet_controller(body, context):
return {
'greeting': f"Hi, {body['name']}!"
}
# Instantiate a Flask application
app = Flask(__name__)
# Handle BLEST requests
@app.post('/')
async def index():
result, error = await router.handle(request.json, { 'headers': request.headers })
if error:
resp = make_response(error, error.status or 500)
resp.headers['Content-Type'] = 'application/json'
else:
resp = make_response(result, 200)
resp.headers['Content-Type'] = 'application/json'
return resp
from blest import HttpClient
async def main():
# Create a client
client = HttpClient('http://localhost:8080', {
'max_batch_size': 25,
'buffer_delay': 10,
'http_headers': {
'Authorization': 'Bearer token'
}
})
# Send a request
try:
result = await client.request('greet', { 'name': 'Steve' })
# Do something with the result
except Exception as error:
# Do something in case of error
This project is licensed under the MIT License.
FAQs
The Python reference implementation of BLEST (Batch-able, Lightweight, Encrypted State Transfer), an improved communication protocol for web APIs which leverages JSON, supports request batching by default, and provides a modern alternative to REST.
We found that blest demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.