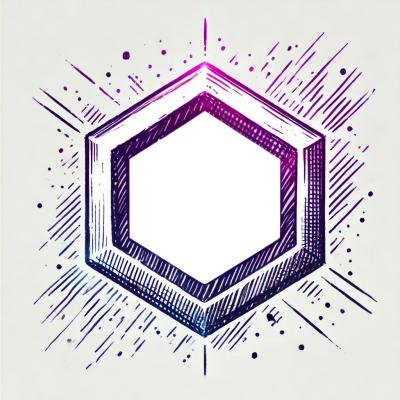
Security News
Maven Central Adds Sigstore Signature Validation
Maven Central now validates Sigstore signatures, making it easier for developers to verify the provenance of Java packages.
Unofficial Python wrapper for the BMKG (Meteorology, Climatology, and Geophysical Agency) API.
Unofficial Python wrapper for the BMKG (Meteorology, Climatology, and Geophysical Agency) API.
$ pip install bmkg
# import the module
import bmkg
import asyncio
import os
async def getweather():
# declare the client. the measuring unit used defaults to the metric system (celcius, km/h, etc.)
async with bmkg.Client(unit=bmkg.IMPERIAL) as client:
# fetch a weather forecast from a province
weather = await client.get_forecast(bmkg.Province.JAKARTA)
# get the weather forecast across various locations
for forecast in weather.forecasts:
# temperature of this forecast across various timeframes
for temp in weather.temperature:
print(f'temperature at {temp.date!r} is {temp.value!r}')
if __name__ == '__main__':
# see https://stackoverflow.com/questions/45600579/asyncio-event-loop-is-closed-when-getting-loop
# for more details
if os.name == 'nt':
asyncio.set_event_loop_policy(asyncio.WindowsSelectorEventLoopPolicy())
asyncio.run(getweather())
# import the module
import bmkg
import asyncio
import os
async def getweather():
# declare the client. the measuring unit used defaults to the metric system (celcius, km/h, etc.)
async with bmkg.Client(unit=bmkg.IMPERIAL) as client:
# fetch the latest earthquake
earthquake = await client.get_latest_earthquake()
print(repr(earthquake))
if __name__ == '__main__':
# see https://stackoverflow.com/questions/45600579/asyncio-event-loop-is-closed-when-getting-loop
# for more details
if os.name == 'nt':
asyncio.set_event_loop_policy(asyncio.WindowsSelectorEventLoopPolicy())
asyncio.run(getweather())
# import the module
import bmkg
import asyncio
import os
async def getweather():
# declare the client. the measuring unit used defaults to the metric system (celcius, km/h, etc.)
async with bmkg.Client(unit=bmkg.IMPERIAL) as client:
# fetch the most recent earthquakes magnitude 5 or higher
earthquakes = await client.get_recent_earthquakes()
# iterate through the generator
for earthquake in earthquakes:
print(repr(earthquake))
if __name__ == '__main__':
# see https://stackoverflow.com/questions/45600579/asyncio-event-loop-is-closed-when-getting-loop
# for more details
if os.name == 'nt':
asyncio.set_event_loop_policy(asyncio.WindowsSelectorEventLoopPolicy())
asyncio.run(getweather())
# import the module
import bmkg
import asyncio
import os
async def getweather():
# declare the client. the measuring unit used defaults to the metric system (celcius, km/h, etc.)
async with bmkg.Client(unit=bmkg.IMPERIAL) as client:
# fetch the most recent earthquakes regardless of their magnitude
earthquakes = await client.get_felt_earthquakes()
# iterate through the generator
for earthquake in earthquakes:
print(repr(earthquake))
if __name__ == '__main__':
# see https://stackoverflow.com/questions/45600579/asyncio-event-loop-is-closed-when-getting-loop
# for more details
if os.name == 'nt':
asyncio.set_event_loop_policy(asyncio.WindowsSelectorEventLoopPolicy())
asyncio.run(getweather())
FAQs
Unofficial Python wrapper for the BMKG (Meteorology, Climatology, and Geophysical Agency) API.
We found that bmkg demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Maven Central now validates Sigstore signatures, making it easier for developers to verify the provenance of Java packages.
Security News
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
Research
Security News
Socket researchers uncovered a backdoored typosquat of BoltDB in the Go ecosystem, exploiting Go Module Proxy caching to persist undetected for years.