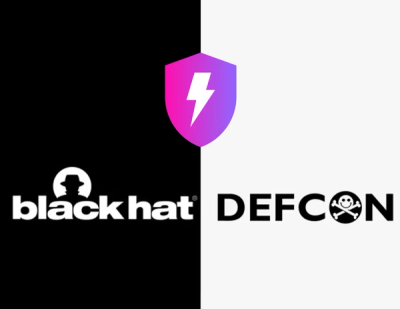
Security News
Meet Socket at Black Hat and DEF CON 2025 in Las Vegas
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
common-expression-language
Advanced tools
The Common Expression Language (CEL) is a non-Turing complete language designed for simplicity, speed, and safety. CEL is primarily used for evaluating expressions in a variety of applications, such as policy evaluation, state machine transitions, and graph traversals.
This Python package wraps the Rust implementation cel-interpreter.
Install from PyPI:
pip install common-expression-language
Basic usage:
from cel import evaluate
expression = "age > 21"
result = evaluate(expression, {"age": 18})
print(result) # False
Simply pass the CEL expression and a dictionary of context to the evaluate
function. The function
returns the result of the expression evaluation converted to Python primitive types.
CEL supports a variety of operators, functions, and types
evaluate(
'resource.name.startsWith("/groups/" + claim.group)',
{
"resource": {"name": "/groups/hardbyte"},
"claim": {"group": "hardbyte"}
}
)
True
This Python library supports user defined Python functions in the context:
from cel import evaluate
def is_adult(age):
return age > 21
evaluate("is_adult(age)", {'is_adult': is_adult, 'age': 18})
# False
You can also explicitly create a Context object:
from cel import evaluate, Context
def is_adult(age):
return age > 21
context = Context()
context.add_function("is_adult", is_adult)
context.update({"age": 18})
evaluate("is_adult(age)", context)
# False
uv run pytest --log-cli-level=debug
The package (plans to) provides a command line interface for evaluating CEL expressions:
$ python -m cel '1 + 2'
3
FAQs
Unknown package
We found that common-expression-language demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.