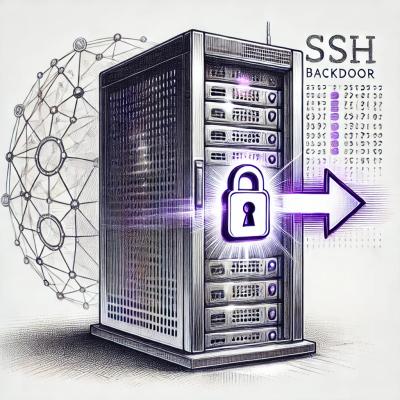
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Confingo is a Python package that simplifies configuration management using YAML and JSON files. It allows you to load, manipulate, and serialize configurations with ease, providing both attribute-style and dictionary-style access to configuration parameters.
You can install Confingo via pip:
pip install confingo
Confingo supports loading configurations from both YAML and JSON files.
from confingo import load_config
config = load_config('config.yaml') # Automatically detects YAML based on file extension
print(config.database.host)
print(config['database']['host'])
from confingo import load_config_from_json
config = load_config_from_json('config.json')
print(config.server.host)
print(config['server']['host'])
You can also load configurations from strings, bytes, or file-like objects.
from confingo import load_config_from_content
yaml_content = """
database:
host: localhost
port: 5432
"""
config = load_config_from_content(yaml_content)
print(config.database.host)
from confingo import load_config_from_content
json_content = """
{
"server": {
"host": "127.0.0.1",
"port": 8080
}
}
"""
config = load_config_from_content(json_content)
print(config.server.host)
from confingo import load_config_from_content
from io import StringIO
yaml_content = """
database:
host: localhost
port: 5432
"""
file_like_object = StringIO(yaml_content)
config = load_config_from_content(file_like_object)
print(config.database.port)
You can create configurations programmatically without using a YAML or JSON file.
from confingo import Config
config = Config()
config.database = Config()
config.database.host = 'localhost'
config.database.port = 5432
config.server = Config()
config.server.host = '127.0.0.1'
config.server.port = 8080
print(config.database.host) # Outputs: localhost
print(config['server']['port']) # Outputs: 8080
Confingo allows you to access configuration parameters using both attribute and key access.
# Attribute access
print(config.database.host)
# Key access
print(config['database']['host'])
Confingo provides methods to delete configuration parameters using both attribute-style and dictionary-style access.
from confingo import Config
config = Config()
config.database = Config()
config.database.host = 'localhost'
config.database.port = 5432
# Delete an attribute
del config.database.port
# Verify deletion
assert not hasattr(config.database, 'port')
assert 'port' not in config.database
from confingo import Config
config = Config()
config['server'] = Config()
config['server']['host'] = '127.0.0.1'
config['server']['port'] = 8080
# Delete an item
del config['server']['port']
# Verify deletion
assert 'port' not in config.server
assert not hasattr(config.server, 'port')
Confingo provides methods to serialize and deserialize configurations to and from YAML and JSON formats.
yaml_str = config.to_yaml()
print(yaml_str)
with open('output.yaml', 'w') as f:
config.dump_yaml(f)
json_str = config.to_json(indent=2)
print(json_str)
with open('output.json', 'w') as f:
config.dump_json(f, indent=2)
Given a config.yaml
file:
database:
host: localhost
port: 5432
users:
- name: admin
role: superuser
- name: guest
role: read-only
server:
host: 127.0.0.1
port: 8080
You can load and access the configuration as follows:
from confingo import load_config
config = load_config('config.yaml')
# Access database host
print(config.database.host) # Outputs: localhost
# Access server port
print(config.server.port) # Outputs: 8080
# List all users
for user in config.database.users:
print(f"{user.name} - {user.role}")
localhost
8080
admin - superuser
guest - read-only
from confingo import load_config
config = load_config('config.yaml')
# Serialize to YAML string
yaml_output = config.to_yaml()
print(yaml_output)
# Serialize to JSON string
json_output = config.to_json(indent=2)
print(json_output)
# Dump serialized YAML to a file
with open('serialized_config.yaml', 'w') as f:
config.dump_yaml(f)
# Dump serialized JSON to a file
with open('serialized_config.json', 'w') as f:
config.dump_json(f, indent=2)
Config
ClassA class representing a configuration object that supports both dictionary-style and attribute-style access to its elements.
Inherits from argparse.Namespace
and dict
to provide a flexible configuration management solution, allowing nested configurations, attribute access, and YAML/JSON-based serialization and deserialization.
to_dict()
: Converts the Config
object to a native Python dictionary.
config_dict = config.to_dict()
to_yaml(**kwargs)
: Serializes the Config
object to a YAML-formatted string.
yaml_str = config.to_yaml()
dump_yaml(stream: IO[Any], **kwargs)
: Writes the Config
object as YAML to a file-like stream.
with open('output.yaml', 'w') as f:
config.dump_yaml(f)
to_json(**kwargs)
: Serializes the Config
object to a JSON-formatted string.
json_str = config.to_json(indent=2)
dump_json(stream: IO[Any], **kwargs)
: Writes the Config
object as JSON to a file-like stream.
with open('output.json', 'w') as f:
config.dump_json(f, indent=2)
__delattr__(key: str) -> None
: Deletes an attribute from the Config
object, removing it from both attribute-style and dictionary-style access.
del config.attribute_name
__delitem__(key: str) -> None
: Deletes an item from the Config
object using dictionary-style access, removing it from both attribute-style and dictionary-style access.
del config['item_name']
load_config(path: Union[str, Path]) -> Config
: Loads a YAML or JSON configuration file from a given file path and returns it as a Config
object.
config = load_config('config.yaml')
load_config_from_content(stream: Union[str, bytes, IO[Any]]) -> Config
: Loads a YAML or JSON configuration from various input types (string, bytes, or file-like object) and returns it as a Config
object.
config = load_config_from_content(yaml_content)
config = load_config_from_content(json_content)
Confingo includes a comprehensive test suite to ensure reliability and correctness. The tests cover configuration loading, access methods, serialization, deletion, and error handling.
To run the tests, ensure you have pytest
installed and execute:
pytest
This command will automatically discover and run all the tests defined in your test suite.
The test suite covers the following aspects:
delattr
and del
operations.__str__
and __repr__
provide accurate outputs.Confingo is licensed under the MIT License.
Ben Elfner
FAQs
A Python package for easy configuration management using YAML files.
We found that confingo demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.