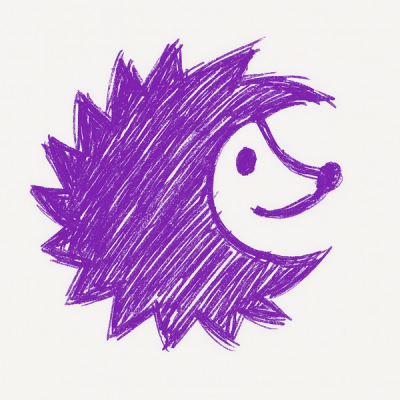
Security News
Browserslist-rs Gets Major Refactor, Cutting Binary Size by Over 1MB
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
elasticsearch-query-builder
Advanced tools
A tool for forming a single query body for further search in Elasticsearch
Requirements:
3.8
or higherpip install elasticsearch-query-builder
To use Elasticsearch query builder, you need to describe the inheritor class from the base builder class, and then describe the expected fields as class attributes
from elasticsearch_query_builder import ElasticsearchQueryBuilder, fields
class BookQueryBuilder(ElasticsearchQueryBuilder):
search = fields.MultiMatchElasticField(
query_type="best_fields",
fields=[
"field_1",
"field_2",
"field_3",
"obj.field_4",
]
)
with_reviews = fields.TermElasticField(
input_type=bool,
field_name="has_reviews"
)
author = fields.NestedElasticField(
path="authors",
child=fields.MatchElasticField(
input_type=str,
field_name="name"
)
)
The next step is to call the described class, passing it an object with request parameters in the constructor
...
builder = BookQueryBuilder({"search": "World", "with_reviews": "true", "author": "John Doe"})
query = builder.query
print(query)
As a result of execution, a query object will be constructed, which can be sent to Elasticsearch for search
{
"query": {
"bool": {
"must": [
{
"multi_match": {
"query": "World",
"type": "best_fields",
"fields": [
"field_1",
"field_2",
"field_3",
"obj.field_4"
]
}
},
{
"term": {
"has_reviews": true
}
},
{
"nested": {
"path": "authors",
"query": {
"match": {
"authors.name": {
"query": "John Doe"
}
}
}
}
}
]
}
}
}
FAQs
A tool for forming a single query body for further search in Elasticsearch
We found that elasticsearch-query-builder demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.