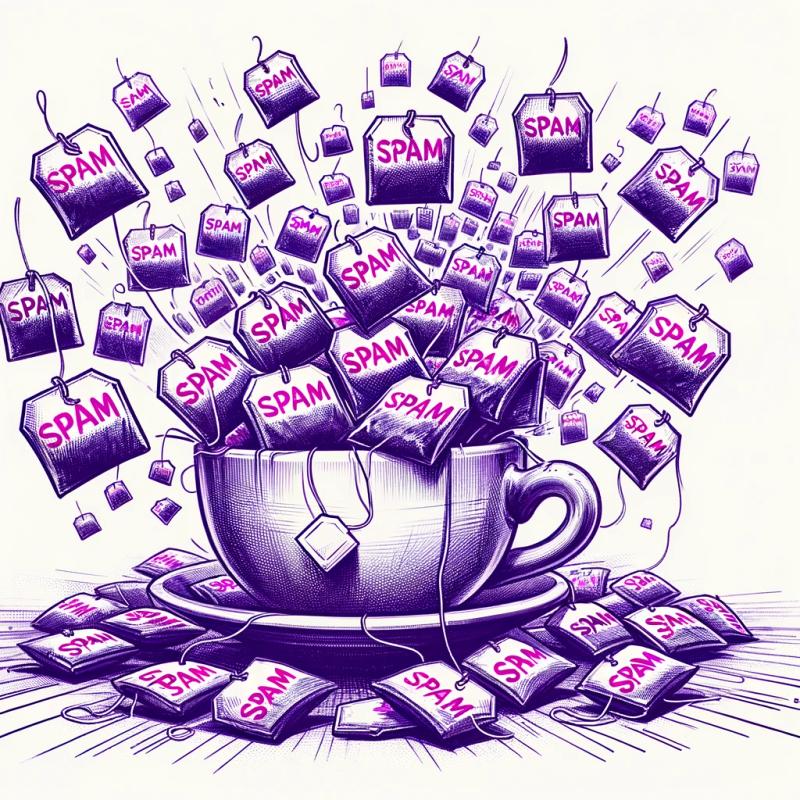
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Readme
Query builder for EdgeDB
Many examples of queries are given in the documentation directory.
from edgeql_qb import EdgeDBModel
from edgeql_qb.types import int16
from edgedb.blocking_client import create_client
client = create_client()
Movie = EdgeDBModel('Movie')
Person = EdgeDBModel('Person')
insert = Movie.insert.values(
title='Blade Runner 2049',
year=int16(2017),
director=(
Person.select()
.where(Person.c.id == director_id)
.limit1
),
actors=Person.insert.values(
first_name='Harrison',
last_name='Ford',
),
).build()
select = (
Movie.select(
Movie.c.title,
Movie.c.year,
Movie.c.director(
Movie.c.director.first_name,
Movie.c.director.last_name,
),
Movie.c.actors(
Movie.c.actors.first_name,
Movie.c.actors.last_name,
),
)
.where(Movie.c.title == 'Blade Runner 2049')
.build()
)
delete = Movie.delete.where(Movie.c.title == 'Blade Runner 2049').build()
decade = (Movie.c.year // 10).label('decade')
group = Movie.group().using(decade).by(decade).build()
client.query(insert.query, **insert.context)
result = client.query(select.query, **select.context)
movies_by_decade = client.query(group.query, **group.context)
client.query(delete.query, **delete.context)
Queries:
Types:
Functions
FAQs
EdgeQL Query Builder
We found that elgeql-qb demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.