exex

Extract data from Excel documents
Installation
pip install exex
Usage
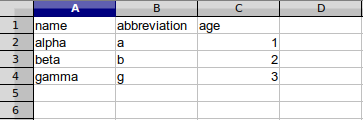
Load Excel file
from openpyxl import load_workbook
from exex import parse
book = load_workbook("sample.xlsx")
sheet = book.active
Single cell by name
parse.values(sheet["A1"])
"name"
Single cell by row/column number
parse.values(sheet.cell(row=1, column=1))
"name"
Range of cells
parse.values(sheet["A1":"B2"])
[
["name", "abbreviation"],
["alpha", "a"],
]
All cells
parse.values(sheet.values)
[
["name", "abbreviation", "age"],
["alpha", "a", 1],
["beta", "b", 2],
["gamma", "g", 3],
]
Row by number
parse.values(sheet[1])
["alpha", "a", 1]
Range of rows
parse.values(sheet[1:2])
[
["name", "abbreviation", "age"],
["alpha", "a", 1],
]
Column by name
parse.values(sheet["A"])
["name", "alpha", "beta", "gamma"]
Rangge of columns
parse.values(sheet["A:B"])
[
["name", "alpha", "beta", "gamma"],
["abbreviation", "a", "b", "g"],
]
Ways to access sheets
book.sheets[0]
book.sheets["prices"]
book.active
book.sheetnames
Development
Setup
poetry install
Tests (local Python version)
poetry run pytest
Tests (all Python versions defined in tox.ini
)
poetry run tox
Code formatting (black)
poetry run black .