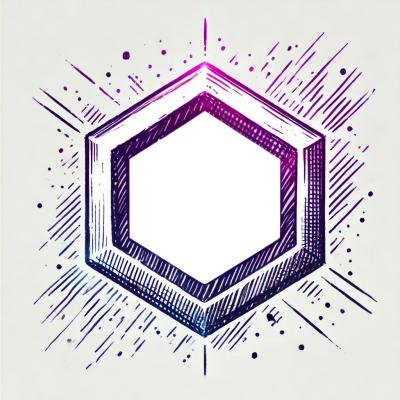
Security News
Maven Central Adds Sigstore Signature Validation
Maven Central now validates Sigstore signatures, making it easier for developers to verify the provenance of Java packages.
Implement CAPTCHA verification for Flask in a straightforward manner.
pip install flask-tjfu-captcha
from datetime import timedelta
from flask import Flask
from flask_tjfu_captcha import TjfuCaptcha
app = Flask(__name__)
tjfu_captcha = TjfuCaptcha(
app,
True # Must be in Debug mode
)
@app.route('/get_captcha')
def get_captcha():
return tjfu_captcha.generate_image_captcha(
secret_key="YOUR_SECRET_KEY",
length=4,
expires_in=timedelta(minutes=5),
only_digits=True
).to_dict()
@app.route('/verify_captcha')
@tjfu_captcha.required_captcha(
"YOUR_SECRET_KEY"
)
def verify_captcha():
return "OK"
if __name__ == '__main__':
app.run(debug=True)
/get_captcha
will generate a JSON with two attributes:
encrypted_code
: encoded information of the generated captcha.img_base64
: base64 encoded string of the captcha image.{
"encrypted_code": "...",
"img_base64": "..."
}
/verify_captcha
uses required_captcha
to enforce captcha verification before executing requests
within verify_captcha
. To pass captcha verification, the client must provide two headers: Tjfu-Captcha-Code
containing the captcha code visible in the captcha image requested, and Tjfu-Captcha-Encrypted-Code
containing the
encoded information mentioned above.
GET http://0.0.0.0:5000/verify_captcha
Tjfu-Captcha-Encrypted-Code: <encrypted_code>
Tjfu-Captcha-Code: <code>
To bypass captcha verification during testing, you can use the Tjfu-Captcha-Debugging-Ignore
header with any value,
and TjfuCaptcha must be in Debug mode.
from flask import Flask
from flask_tjfu_captcha import TjfuCaptcha
app = Flask(__name__)
tjfu_captcha = TjfuCaptcha(
app,
True # Must be in Debug mode
)
GET http://0.0.0.0:5000/verify_captcha
Tjfu-Captcha-Debugging-Ignore: <you can put any value>
You can customize headers for captcha verification
using TJFU_CAPTCHA_ENCRYPTED_CODE_HEADER_KEY
, TJFU_CAPTCHA_CAPTCHA_CODE_HEADER_KEY
, TJFU_CAPTCHA_DEBUGGING_IGNORE_HEADER_KEY
,
and TJFU_CAPTCHA_FONTS
to adjust captcha image fonts.
from flask import Flask
app = Flask(__name__)
app.config['TJFU_CAPTCHA_FONTS'] = "['your_font_path', 'your_other_font_path']"
# Change Tjfu-Captcha-Encrypted-Code header to Request-ID
app.config['TJFU_CAPTCHA_ENCRYPTED_CODE_HEADER_KEY'] = 'Request-ID'
# Change Tjfu-Captcha-Code header to My-Captcha-Code
app.config['TJFU_CAPTCHA_CAPTCHA_CODE_HEADER_KEY'] = 'My-Captcha-Code'
# Change Tjfu-Captcha-Debugging-Ignore header to Ignore-Captcha
app.config['TJFU_CAPTCHA_DEBUGGING_IGNORE_HEADER_KEY'] = 'Ignore-Captcha'
You can also customize responses for missing headers (on_missing_header
) and invalid captcha
codes (on_invalid_captcha_code
).
from flask import Flask
from flask_tjfu_captcha import TjfuCaptcha
app = Flask(__name__)
tjfu_captcha = TjfuCaptcha(
app,
True
)
def on_missing_header(header, status_code):
return {
"error": f"Missing header: {header}",
"status_code": status_code
}
def on_invalid_captcha_code(status_code):
return {
"error": "Invalid captcha code",
"status_code": status_code
}
tjfu_captcha.on_missing_header(
on_missing_header
)
tjfu_captcha.on_invalid_captcha_code(
on_invalid_captcha_code
)
Each section in this changelog provides a summary of what was added, changed, fixed, or removed in each release of the software. This helps users and developers understand the evolution of the project over time and highlights important updates or improvements made in each version.
FAQs
Implement CAPTCHA verification for Flask in a straightforward manner.
We found that flask-tjfu-captcha demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Maven Central now validates Sigstore signatures, making it easier for developers to verify the provenance of Java packages.
Security News
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
Research
Security News
Socket researchers uncovered a backdoored typosquat of BoltDB in the Go ecosystem, exploiting Go Module Proxy caching to persist undetected for years.