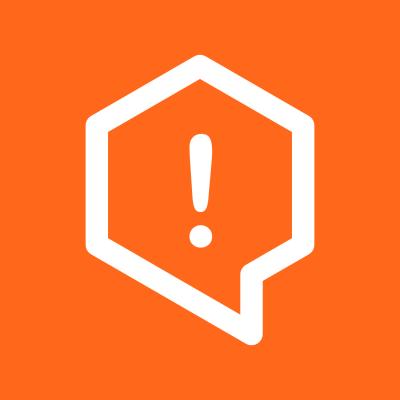
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Python async client for the Harbor REST API v2.0 based on the official Harbor REST API specification.
NOTE: The official Harbor API spec is hand-written, and numerous errors and inconsistencies have been found in it. This library attempts to work around these issues as much as possible, but errors may still occur. If you find any errors, please open an issue.
pip install harborapi
Documentation is available here. The documentation is still a work in progress, and you may have to dig around a bit to find what you're looking for.
Creating proper documentation for the Pydantic models is priority number one right now, but is largely blocked by the lack of inheritance support in the mkdocstrings python plugin.
from harborapi import HarborAsyncClient
client = HarborAsyncClient(
url="https://demo.goharbor.io/api/v2.0/",
username="username",
secret="secret",
# OR
basicauth="base64-basic-auth-credentials",
# OR
credentials_file="/path/to/robot-credentials-file.json",
)
import asyncio
from harborapi import HarborAsyncClient
client = HarborAsyncClient(
# ...
)
async def main() -> None:
# Get all projects
projects = await client.get_projects()
for project in projects:
print(project.name)
# If you have rich installed:
import rich
for project in projects:
rich.print(project)
asyncio.run(main())
import asyncio
from harborapi import HarborAsyncClient
from harborapi.models import ProjectReq, ProjectMetadata
client = HarborAsyncClient(
# ...
)
async def main() -> None:
project_path = await client.create_project(
ProjectReq(
project_name="test-project",
metadata=ProjectMetadata(
public=True,
),
)
)
print(f"Project created: {project_path}")
asyncio.run(main())
All endpoints are documented in the endpoints documentation.
harborapi
makes use of code generation for its data models, but it doesn't entirely rely on it like, for example, githubkit. Thus, while the library is based on the Harbor REST API specification, it is not beholden to it. The official schema contains several inconsistencies and errors, and this package takes steps to rectify some of these locally until they are fixed in the official Harbor API spec.
harborapi
attempts to improve endpoint descriptions where possible and fix models with fields given the wrong type or wrongly marked as required. Without these changes, the validation provided by the library would be unusable for certain endpoints, as these endpoints can, in certain cases, return data that is inconsistent with the official API specification, thus breaking the model validation.
To return the raw API responses without validation and type conversion, set raw=True
when instantiating the client. For more information, check the documentation on validation.
HarborAsyncClient.get_project_artifacts()
changes intended for 0.26.0.HarborAsyncClient.get_project_artifacts()
with_sbom_overview
parameter for HarborAsyncClient.get_artifact()
and HarborAsyncClient.get_artifacts()
to include SBOM overview in the response.harborapi.models.base.RootModel
base class.harborapi.models.base.StrDictRootModel
harborapi.models.base.StrRootModel
ProjectMetadata.proxy_speed_kb
Configurations.ldap_group_attach_parallel
ConfigurationsResponse.ldap_group_attach_parallel
Robot.creator
Artifact.artifact_type
Artifact.repository_name
HarborVulnerabilityReport.severity
when report contains no vulnerabilities.default=
kwarg for default values. This prevents certain type checking errors when the model is instantiated without specifying the field.harborapi.models.mappings.FirstDict
which is a subclass of Python's built-in dict
that provides a first()
method to get the first value in the dict (or None
if the dict is empty).HarborAsyncClient.get_artifact_vulnerability_reports()
now returns FirstDict
to provide easier access to the first (and likely only) report for the artifact.HarborAsyncClient.get_permissions()
HarborAsyncClient.get_project_immutable_tag_rules()
HarborAsyncClient.create_project_immutable_tag_rule()
HarborAsyncClient.update_project_immutable_tag_rule()
HarborAsyncClient.enable_project_immutable_tagrule()
HarborAsyncClient.delete_project_immutable_tag_rule()
HarborAsyncClient.get_artifact_vulnerability_reports()
HarborAsyncClient.get_robots_v1()
HarborAsyncClient.create_robot_v1()
HarborAsyncClient.get_robot_v1()
HarborAsyncClient.update_robot_v1()
HarborAsyncClient.delete_robot_v1()
HarborAsyncClient.search()
.HarborAsyncClient.get_artifact_vulnerabilities()
in favor of HarborAsyncClient.get_artifact_vulnerability_reports()
.
harborapi.models.SBOMOverview
which represents the SBOM overview for an artifact. Can be accessed via Artifact.sbom_overview
.*audit_log_rotation*
methods are now deprecated and will be removed in a future release.StrDictRootModel
and StrRootModel
.HarborAsyncClient.authenticate(verify=...)
will no longer instantiate a new client object if the verify
value is identical to current one.example
keyword arguments.HarborAsyncClient.authenticate(verify=...)
causing the client to fail to discard Harbor CSRF cookies.verify
parameter for HarborAsyncClient.authenticate()
.ProjectMetadata.auto_sbom_generation
ReplicationFilter.value
validation failing due to receiving non-dict value. Now accepts str
, dict[str, Any]
and None
.The big Pydantic V2 update. This is a major update in terms of both scope and API compatibility.
str
fields will no longer coerce int
values to strings.pydantic.RootModel
and have a single field called root
. These models have a special __getitem__
method, so they can be accessed like a dict. Currently, these are:
harborapi.models.ExtraAttrs
harborapi.models.Annotations
harborapi.models.ResourceList
harborapi.models.QuotaRefObject
harborapi.models.EventType
harborapi.models.NotifyType
harborapi.models.PayloadFormatType
harborapi.models.AdditionLinks
harborapi.models.InternalConfigurationsResponse
harborapi.models.ScanOverview
harborapi.models.AdditionLinks
harborapi.models.ProjectMetadata
:
retention_id
now accepts both string and integer arguments. Band-aid fix until Harbor fixes their API spec and specifies that all retention IDs should be ints, not strings.harborapi.models.Artifact
:
scan_overview
is now a mapping of MIME types and their associated scan overview. Previously, we used some dirty metaprogramming to populate this field with the first scan overview found in the mapping. A new scan
attribute has been added to access the first scan overview in the mapping, which is the same as the old behavior. This ensures serializing the model matches the original JSON from the API.harborapi.ext.report.ArtifactReport
:
artifacts
attribute instead.harborapi.models.ProjectMetadata
model. This aberrant behavior is limited to ProjectMetadata, so the converter has been moved to the model itself. This band-aid fix will remain until Harbor changes their API spec and make these fields bools.missing_ok
parameter for all relevant client methods.harborapi.models._models
module. All main API spec models are now defined harborapi.models.models
.harborapi.models._scanner
module. All scanner models are now defined in harborapi.models.scanner
.httpx
>= 0.22.0typing_extensions
>= 4.4limit
argument for methods that fetch multiple resources:
HarborAsyncClient.get_replication_tasks
HarborAsyncClient.search_usergroups
HarborAsyncClient.get_webhook_policy_last_trigger
HarborAsyncClient.search_ldap_groups
HarborAsyncClient.search_ldap_users
HarborAsyncClient.get_registry_adapters
HarborAsyncClient.get_artifact_build_history
HarborAsyncClient.get_replication_tasks
argument order changed. Now status
and resource_type
follow the required argument project_id
instead of after the optional query
, sort
, page
, page_size
and limit
arguments.harborapi.models.ScannerProperties
now takes an arbitrary number of extra fields instead of using a __root__
field.bf7c82b
):
harborapi.models.Tag.signed
harborapi.models.GeneralInfo.with_notary
HarborAsyncClient.delete_scanner
now raises HarborAPIException
if no scanner response is returned from the API (was UnprocessableEntity
before).BaseModel.get_model_fields
, a class method which returns a list of names of all fields defined in the model's schema.PayloadFormatType
PayloadFormat
SupportedWebhookEventTypes.payload_formats
Accessory.subject_artifact_digest
Accessory.subject_artifact_repo
Access.resource
descriptionAccess.action
descriptionComponentHealthStatus.status
descriptionRobot.name
descriptionRobot.expires_at
descriptionRobotCreate.name
descriptionAccessory.subject_artifact_id
ChartMetadata
(deprecated)ChartVersion
(deprecated)SearchResult
(deprecated)Project.chart_count
(deprecated)BaseModel.convert_to
, which allows converting a model to another model type that has a similar schema, such as Project
to ProjectReq
.BaseModel
now correctly handles assignments.HarborAsyncClient.no_retry
HarborAsyncClient.no_validation
HarborAsyncClient.raw_mode
password
kwarg for HarborAsyncClient
constructor, while emitting warning for it. It isn't encouraged (or documented) to use password
, but it might be more natural for many users than secret
, so we allow it, since we already accept arbitrary kwargs.RetrySettings.max_tries
now only accepts values greater than 0.RetrySettings.max_time
now only accepts values greater or equal to 0.models.base.BaseModel.bool_converter
renamed to models.base.BaseModel._bool_converter
to hide it in IDE autocompletion. This shouldn't be a breaking change for anyone, since it was never documented or intended to be used by anyone outside of the library.RetrySettings
class, which can be passed to the HarborAsyncClient
constructor with the retry
keyword argument. See the retry docs for more information.Accept
header have been fixed. This includes:
HarborAsyncClient.download_scan_export
HarborAsyncClient.get_system_certificate
harborapi.errors.StatusError.status_code
is now always an integer. Returns 0 if no request is associated with the error.
harborapi.HarborAsyncClient.delete_retention_policy
, warning that it can break a project due to a bug in Harbor.harborapi.client.ResponseLog
and harborapi.client.ResponseLogEntry
to harborapi.responselog
module.harborapi.models.VulnerabilityItem.severity
field has had its type reverted to Severity
from Optional[Severity]
, which was a regression introduced in v0.16.0.harborapi.ext.regex.match
return type annotation is now correctly marked as Optional[Match[str]]
instead of Match[str]
.harborapi.HarborAsyncClient.get_retention_tasks
missing limit
parameter in docstring.NativeReportSummary
to Artifact.scan_overview
no longer raises a ValidationError
exception.harborapi.models.models
to provide better documentation both in the code and in the generated documentation. This change should be backwards-compatible.harborapi.HarborAsyncClient.add_group_member
now has a clearner warning describing its purpose and the alternative methods to use instead.harborapi.models.RetentionRule.params
harborapi.models.ImutableRule.params
Until the official API spec is fixed, this is the best we can do.harbor
being added as an executable script installed by the project. This was a mistake, as the harbor
executable script is intended to be exposed by harbor-cli.HarborAsyncClient.authenticate()
. This method can be used to re-authenticate the client with new credentials without having to create a new client instance.HarborAsyncClient.get_artifact_vulnerabilities()
now always returns a harborapi.models.HarborVulnerabilityReport
object. If the artifact has no vulnerabilities or the report cannot be processed, an exception is raised.config
argument from HarborAsyncClient.__init__()
. The config
argument was never implemented.HarborAsyncClient.get_project_retention_id()
HarborAsyncClient.get_retention_policy()
HarborAsyncClient.create_retention_policy()
HarborAsyncClient.update_retention_policy()
HarborAsyncClient.delete_retention_policy()
HarborAsyncClient.get_retention_tasks()
HarborAsyncClient.get_retention_metadata()
HarborAsyncClient.get_retention_execution_task_log()
HarborAsyncClient.get_retention_executions()
HarborAsyncClient.start_retention_execution()
HarborAsyncClient.stop_retention_execution()
verify
kwarg for HarborAsyncClient
and HarborClient
which is passed to the underlying httpx.AsyncClient
. This is useful for self-signed certificates, or if you want to control the SSL verification yourself. See httpx documentation for more information.harborapi.exceptions.StatusError.response
which holds the HTTPX response object that caused the exception.harborapi.ext
, where HarborAsyncClient
is imported as a type annotation.limit
kwarg now treats 0
as no limit. Previously, 0
meant no results would be returned.harborapi.ext.artifact.ArtifactInfo.tags
now returns a list of tags instead of a comma-separated string of tags. This gives more flexibility to work with the various tags, and is more consistent with the rest of the library. If you need the comma-separated string, you can use ", ".join(artifact_info.tags)
.?q=operation={push pull}
or ?q=operation=(push pull)
.HarborAsyncClient.update_project_member_role()
now accepts integer arguments for its role_id
parameter, since RoleRequest
only has a single field (role_id
).models.VulnerabilitySummary
if summary
is None
.HarborAsyncClient.get_audit_log_rotation_schedule()
that could occur if no schedule exists. The API returns an emtpy 200 OK
response, which is now handled correctly (empty ExecHistory
object).HarborAsycClient.get_project_members
.HarborAsyncClient.export_scan_data()
now takes the arguments in the order (criteria
, scan_type
). Furthermore, scan_type
now has a default argument of "application/vnd.security.vulnerability.report; version=1.1"
, per the blog post describing this new feature. It should not be necessary specify this argument, but it is still possible to do so if you need to.group_name
parameter for HarborAsyncClient.get_usergroups()
this time.group_name
and limit
parameters for HarborAsyncClient.get_usergroups()
.group_name
and limit
parameters for HarborAsyncClient.get_usergroups()
.HarborAsyncClient.get_system_certificate()
GET /api/v2.0/systeminfo/getcert
)FileResponse
instead of a bytes object. FileResponse
contains the file contents along with its metadata. The object can be passed to bytes()
to get the response contents, otherwise it can be accessed via the FileResponse.content
attribute.HarborAsyncClient.get_purge_audit_log_status()
-> HarborAsyncClient.get_audit_log_rotation()
HarborAsyncClient.get_purge_audit_log()
-> HarborAsyncClient.get_audit_log_rotation_log()
HarborAsyncClient.stop_purge_audit_log()
-> HarborAsyncClient.stop_audit_log_rotation()
HarborAsyncClient.get_purge_audit_log_schedule()
-> HarborAsyncClient.get_audit_log_rotation_schedule()
HarborAsyncClient.create_purge_audit_log_schedule()
-> HarborAsyncClient.create_audit_log_rotation_schedule()
HarborAsyncClient.update_purge_audit_log_schedule()
-> HarborAsyncClient.update_audit_log_rotation_schedule()
HarborAsyncClient.get_purge_audit_logs()
-> HarborAsyncClient.get_audit_log_rotation_history()
harborapi.ext.artifact.ArtifactInfo.name_with_digest_full
which returns the artifact name with the full SHA256 digest, not just the first 15 characters like name_with_digest
.HarborAsyncClient.get_purge_audit_log_status()
HarborAsyncClient.get_purge_audit_log()
HarborAsyncClient.stop_purge_audit_log()
HarborAsyncClient.get_purge_audit_log_schedule()
HarborAsyncClient.create_purge_audit_log_schedule()
HarborAsyncClient.update_purge_audit_log_schedule()
HarborAsyncClient.get_purge_audit_logs()
HarborAsyncClient.get_project_deletable()
.HarborAsyncClient.get_webhook_jobs()
HarborAsyncClient.get_webhook_policies()
HarborAsyncClient.get_webhook_policy()
HarborAsyncClient.create_webhook_policy()
HarborAsyncClient.update_webhook_policy()
HarborAsyncClient.delete_webhook_policy()
HarborAsyncClient.get_webhook_policy_last_trigger()
HarborAsyncClient.get_webhook_supported_events()
HarborAsyncClient.get_scan_export()
HarborAsyncClient.get_scan_exports()
HarborAsyncClient.export_scan_data()
HarborAsyncClient.download_scan_export()
HarborAsyncClient.get_icon()
HarborAsyncClient.get_label()
HarborAsyncClient.create_label()
HarborAsyncClient.delete_label()
HarborAsyncClient.get_labels()
HarborAsyncClient.get_project_member()
HarborAsyncClient.add_project_member()
HarborAsyncClient.add_project_member_user()
HarborAsyncClient.add_project_member_group()
HarborAsyncClient.update_project_member_role()
HarborAsyncClient.remove_project_member()
HarborAsyncClient.get_project_members()
harborapi.client.ResponseLog.resize()
harborapi.client.ResponseLog.clear()
basicauth
as a parameter for HarborAsyncClient.__init__()
to pass in base64 basic auth credentials.missing_ok
parameter for DELETE methods has been deprecated. Manually handle harborapi.exceptions.NotFound
instead. This parameter will stop working in version 1.0.0, and be removed altogether in a later release.harborapi.models.Repository.split_name()
now returns a tuple instead of a list, as its docstring states it should.credentials
as a parameter for HarborAsyncClient.__init__
is deprecated. Use basicauth
instead.HarborAsyncClient.credentials
is now a Pydantic SecretStr, which prevents it from being printed in clear text when locals are dumped, such as when printing the client object. To access the value, use HarborAsyncClient.credentials.get_secret_value()
.HarborAsyncClient.set_user_cli_secret()
and HarborAsyncClient.set_user_password()
. The exception handler handles logging if configured.Updated harborapi.models
to match the latest version of the Harbor API spec goharbor/harbor@d03f0dc.
harborapi.models.GeneralInfo.with_chartmuseum
has been removed from the API spec, but remains on the model for backwards compatibility. In the future, this field will be removed, as the API will never return this it in sufficiently new versions of Harbor.
harborapi.models.ScheduleObj
fields are now correctly validated when the Harbor API responds with a value of "Schedule"
for the field ScheduleObj.type
, which is not a valid value for the enum according to their own spec.NativeReportSummary.severity_enum
which returns the severity of the report as a harborarpi.scanner.Severity
enum, which can be used for comparisons between reports.
harborarpi.scanner.Severity
enum not having a None
value, which is observed when a report has no vulnerabilities.
limit
parameter.HarborAsyncClient.get_gc_jobs()
ignoring user parameters.HarborAsyncClient.update_robot_token
renamed to HarborAsyncClient.refresh_robot_token
to better reflect the API endpoint name and purpose.HarborAsyncClient
methods having missing or incomplete docstrings.HarborAsyncClient.get_registry_providers
now returns a RegistryProviders
object, which is a model whose only attribute providers
is a dict of RegistryProviderInfo
objects. Previously this method attempted to return a list of RegistryProviderInfo
objects, but this was incorrect.httpx.RequestError
exceptions. This is to avoid retrying on exceptions that will never succeed such as httpx.UnsupportedProtocol
.limit
parameter for all methods that return a list of items. This parameter is used to limit the number of items returned by the API. See the docs for more details.retrieve_all
parameter for all methods that return a list of items. Use the new limit
parameter to control the number of results to retrieve. Passing retrieve_all
to these methods will be silently ignored. In the future this will raise a DeprecationWarning.raw
and validate
to HarborAsyncClient
and HarborClient
to control whether the client returns the raw data from the API, and whether the client validates the data from the API, respectively. See the docs for more details.models._models
and models._scanner
, and the overrides for these models are defined in models.models
and models.scanner
respectively. This is to make it easier to regenerate the models in the future while keeping the extended functionality (such as Repository.project_name
, ScanOverview.__new__
, etc.) for these classes intact, since that is now declared separately from the generated models. Furthermore, models.models
and models.scanner
both re-export all the generated models so that the API remains unchanged. See the Justfile for more details on how the models are generated.HarborAsyncClient.search()
raising an error when finding Helm Charts with an empty engine
field.HarborAsyncClient.get_internal_config()
. This endpoint is meant for internal usage only, and the new model definitions don't seem to play well with it. If you need this endpoint, please open an issue.max_depth
parameter of the as_table()
and as_panel()
methods on all models now starts counting from 1 instead of 0.
max_depth=0
now means "no limit", and max_depth=1
means "only show the top level" (previously max_depth=0
meant "only show the top level" and max_depth=1
meant "show the top level and one level below")pip install harborapi[rich]
FAQs
Async Harbor API v2.0 client
We found that harborapi demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.