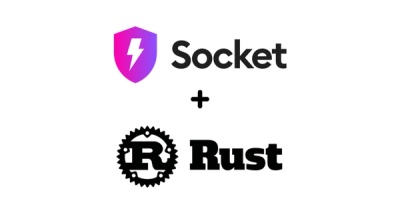
Product
Introducing Rust Support in Socket
Socket now supports Rust and Cargo, offering package search for all users and experimental SBOM generation for enterprise projects.
img-show
is a Python package that simplifies the process of displaying images using OpenCV. It allows you to load, coerce, and display images of various types, shapes, and data formats effortlessly, handling the complexities of image preprocessing and window management for you.
You can install img-show
via pip:
pip install img-show
img-show
provides a simple show_img
function to display images.
from img_show import show_img
# Assuming 'img' is a NumPy array or a PyTorch tensor
show_img(img)
img-show
can handle images in various formats, including NumPy arrays and PyTorch tensors.
import numpy as np
import torch
from img_show import show_img
# NumPy array
img_numpy = np.random.rand(256, 256, 3)
show_img(img_numpy)
# PyTorch tensor
img_tensor = torch.randn(3, 256, 256)
show_img(img_tensor)
You can customize the window name, wait delay, and whether to wait for a key press.
from img_show import show_img
show_img(img, window_name='My Image', wait_delay=5000, do_wait=True)
If the image is larger than the screen, img-show
automatically resizes the window to fit the screen while maintaining the aspect ratio.
from img_show import show_img
# Display a large image
large_img = np.random.rand(4000, 6000, 3)
show_img(large_img)
img-show
can coerce images with extra dimensions (e.g., singleton dimensions) into valid shapes for display.
import numpy as np
from img_show import show_img
# Image with an extra leading dimension
extra_dim_img = np.random.rand(1, 256, 256, 3)
show_img(extra_dim_img)
# Image with multiple singleton dimensions
multiple_extra_dims_img = np.random.rand(1, 1, 256, 256, 3)
show_img(multiple_extra_dims_img)
# Image with singleton channel dimension
singleton_channel_img = np.random.rand(256, 256, 1)
show_img(singleton_channel_img)
img-show
automatically handles different channel orders (e.g., channels-first vs. channels-last).
import numpy as np
from img_show import show_img
# Channels-first format (e.g., PyTorch)
channels_first_img = np.random.rand(3, 256, 256)
show_img(channels_first_img)
channels_first_img = np.random.rand(1, 1, 3, 256, 256, 1)
show_img(channels_first_img)
# Channels-last format (e.g., Numpy)
channels_last_img = np.random.rand(256, 256, 3)
show_img(channels_last_img)
channels_last_img = np.random.rand(1, 1, 256, 256, 3, 1)
show_img(channels_last_img)
show_img
FunctionDisplays an image using OpenCV's imshow
function, automatically handling image coercion and window sizing.
show_img(img: Any, window_name: str = ' ', wait_delay: int = 0, do_wait: bool = True)
img
: The image to display. Can be a NumPy array or a PyTorch tensor.window_name
: The name of the display window (default is a blank space).wait_delay
: The delay in milliseconds for cv2.waitKey()
(default is 0
, which waits indefinitely).do_wait
: Whether to wait for a key press after displaying the image (default is True
).coerce_img
Converts the image to a NumPy array with a valid shape and data type for display.
coerce_img(img: Any) -> np.ndarray
img-show
is licensed under the MIT License.
Ben Elfner
FAQs
A opinionated tool for coercing data into something displayable
We found that img-show demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports Rust and Cargo, offering package search for all users and experimental SBOM generation for enterprise projects.
Product
Socket’s precomputed reachability slashes false positives by flagging up to 80% of vulnerabilities as irrelevant, with no setup and instant results.
Product
Socket is launching experimental protection for Chrome extensions, scanning for malware and risky permissions to prevent silent supply chain attacks.