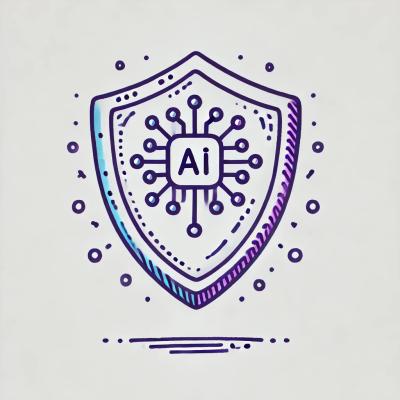
Security News
38% of CISOs Fear They’re Not Moving Fast Enough on AI
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
A Python implementation of an indexed priority queue using a min heap data structure. This data structure maintains a mapping between keys and their priorities while providing efficient O(log n) access to the minimum priority element.
Install using pip:
pip install indexed-heapq
from indexed_heapq import IndexedHeapQueue
# Create an empty queue
queue = IndexedHeapQueue()
# Add some items
queue['task1'] = 3
queue['task2'] = 1
queue['task3'] = 2
# Get the highest priority item (lowest number)
next_task, priority = queue.peek() # ('task2', 1)
# Remove and return the highest priority item
next_task, priority = queue.pop() # ('task2', 1)
# Update a priority
queue['task1'] = 0 # Move task1 to the front
# Access a priority directly
priority = queue['task3'] # 2
# Remove an item
del queue['task3']
# Check if an item exists
if 'task1' in queue:
print("Task1 is in the queue")
Operation | Time Complexity |
---|---|
getitem | O(1) |
peek() | O(1) |
pop() | O(log n) |
insert | O(log n) |
update | O(log n) |
delete | O(log n) |
The class is fully generic and type-safe:
from indexed_heap_queue import IndexedHeapQueue
# With explicit type hints
queue: IndexedHeapQueue[str, float] = IndexedHeapQueue()
queue['task1'] = 1.5
# Or let type inference work
queue = IndexedHeapQueue({ 'task1': 1.5 })
queue['task2'] = 1
This project is licensed under the MIT License - see the LICENSE file for details.
FAQs
Indexed Heap Queue (Priority Queue)
We found that indexed-heapq demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
Research
Security News
Socket researchers uncovered a backdoored typosquat of BoltDB in the Go ecosystem, exploiting Go Module Proxy caching to persist undetected for years.
Security News
Company News
Socket is joining TC54 to help develop standards for software supply chain security, contributing to the evolution of SBOMs, CycloneDX, and Package URL specifications.