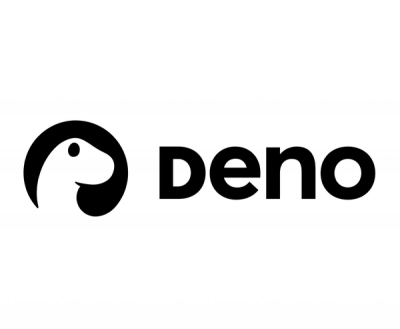
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
NB: End to end tests use Galata framework.
Traitlets and widgets to efficiently data tables (e.g. Pandas DataFrame) using the jupyter notebook
ipytablewidgets is a set of widgets and traitlets to reuse of large tables such as Pandas DataFrames across different widgets, and different packages.
Using pip:
pip install ipytablewidgets
The first step requires the following three commands to be run (requires yarn and jupyterlab>=3):
$ git clone https://github.com/progressivis/ipytablewidgets.git
$ cd ipytablewidgets
$ pip install -e .
The development of extensions for jupyter notebook and jupyter lab requires JavaScript code to be modified in-place. For this reason, lab and notebook extensions need to be configured this way:
$ jupyter nbextension install --py --overwrite --symlink --sys-prefix ipytablewidgets
$ jupyter nbextension enable --py --sys-prefix ipytablewidgets
$ jupyter labextension develop . --overwrite
The main widget for tables is the TableWidget
class. It has a main trait: A
table. This table's main purpose is simply to be a standardized way of transmitting table
data from the kernel to the frontend, and to allow the data to be reused across
any number of other widgets, but with only a single sync across the network.
import pandas as pd
from ipytableidgets import TableWidget, PandasAdapter, serialization
@widgets.register
class MyWidget(DOMWidget):
"""
My widget needing a table
"""
_view_name = Unicode('MyWidgetView').tag(sync=True)
_model_name = Unicode('MyWidgetModel').tag(sync=True)
...
data = Instance(TableWidget).tag(sync=True, **serialization)
def __init__(self, wg, **kwargs):
self.data = wg
super().__init__(**kwargs)
df = pd.DataFrame({'a': [1,2], 'b': [3.5, 4.5], 'c': ['foo','bar'])
table_widget = TableWidget(PandasAdapter(df))
my_widget = MyWidget(table_widget)
You can see EchoTableWidget which is a more realistic example, currently used for end to end testing and demo.
Or, if you prefer to use the TableType traitlet directly:
from ipytablewidgets import serialization, TableType
@widgets.register
class MyWidget(DOMWidget):
"""
My widget needing a table
"""
...
data = TableType(None).tag(sync=True, **serialization)
Developers should consider using ipytablewidgets because:
The major parts of ipyablewidgets are:
FAQs
A set of widgets to help facilitate reuse of large tables across widgets
We found that ipytablewidgets demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.