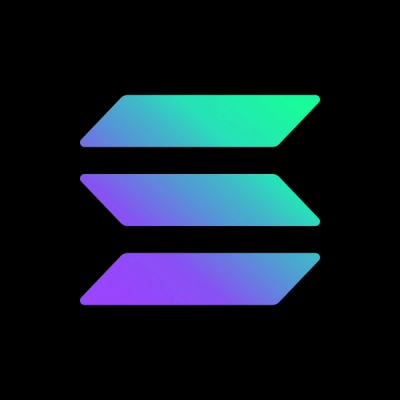
Security News
Supply Chain Attack Detected in Solana's web3.js Library
A supply chain attack has been detected in versions 1.95.6 and 1.95.7 of the popular @solana/web3.js library.
Auto Number Plate Recognition for EU countries
🦋 Available Countries: (We are adding more countries.)
🇦🇱 Albania 🇨🇿 Czechia 🇦🇩 Andorra 🇩🇰 Denmark 🇦🇹 Austria 🇫🇮 Finland
🇧🇪 Belgium 🇫🇷 France 🇧🇦 Bosnia and Herzegovina
🇩🇪 Germany 🇧🇬 Bulgaria 🇬🇷 Greece 🇭🇷 Croatia 🇭🇺 Hungary 🇨🇾 Cyprus 🇮🇪 Ireland
🦋 Recognisable Characters:
char_list = [
"-", ".", "0", "1", "2", "3", "4", "5", "6", "7", "8", "9",
"A", "B", "C", "D", "E", "F", "G", "H", "I", "J", "K", "L", "M", "N",
"O", "P", "Q", "R", "S", "T", "U", "V", "W", "X", "Y", "Z", "a", "d", "i",
"m", "o", "Ö", "Ü", "Ć", "Č", "Đ", "Š", "Ž", "П"
]
한국 자동차 번호판 인식 솔루션
인식 가능 문자:
char_list = [
'0', '1', '2', '3', '4', '5', '6', '7', '8', '9',
'가', '강', '거', '경', '고', '광', '구', '기',
'나', '남', '너', '노', '누',
'다', '대', '더', '도', '동', '두',
'라', '러', '로', '루',
'마', '머', '모', '무', '문',
'바', '배', '버', '보', '부', '북',
'사', '산', '서', '세', '소', '수',
'아', '어', '오', '우', '울', '원', '육', '인',
'자', '저', '전', '제', '조', '종', '주',
'천', '충',
'하', '허', '호'
]
To install the MareArts ANPR package, use the following pip command:
pip install marearts-anpr
For private keys, please visit MareArts ANPR Solution. For inquiries about private keys, contact us at hello@marearts.com.
Check here to see the license plate recognition results in YouTube videos.
Here's an example of how to use the updated SDK:
# pip install marearts-anpr
import cv2
from PIL import Image
from marearts_anpr import ma_anpr_detector
from marearts_anpr import ma_anpr_ocr
from marearts_anpr import marearts_anpr_from_pil
from marearts_anpr import marearts_anpr_from_image_file
from marearts_anpr import marearts_anpr_from_cv2
if __name__ == '__main__':
#################################
## Initiate MareArts ANPR
print("EU ANPR")
user_name = "your_email"
serial_key = "your_serial_key"
detector_model_version = "middle" # Options: middle, v10_small, v10_middle, v10_large
ocr_model_version = "eu" # Options: eu, kr
# MareArts ANPR Detector Inference
anpr_d = ma_anpr_detector(detector_model_version, user_name, serial_key, conf_thres=0.3, iou_thres=0.5)
# MareArts ANPR OCR Inference
anpr_r = ma_anpr_ocr(ocr_model_version, user_name, serial_key)
#################################
#################################
# Routine Task 1 - Predict from File
image_path = './sample_images/eu_test1.jpg'
output = marearts_anpr_from_image_file(anpr_d, anpr_r, image_path)
print(output)
# Routine Task 2 - Predict from cv2
img = cv2.imread(image_path)
output = marearts_anpr_from_cv2(anpr_d, anpr_r, img)
print(output)
# Routine Task 3 - Predict from Pillow
pil_img = Image.open(image_path)
output = marearts_anpr_from_pil(anpr_d, anpr_r, pil_img)
print(output)
#################################
#################################
## Initiate MareArts ANPR for Korea
print("ANPR Korean")
# user_name, serial_key are already defined
# anpr_d is also already initiated before
ocr_model_version = "kr"
# MareArts ANPR OCR Inference
anpr_r = ma_anpr_ocr(ocr_model_version, user_name, serial_key)
#################################
# Routine Task 1 - Predict from File
image_path = './sample_images/kr_test2.jpg'
output = marearts_anpr_from_image_file(anpr_d, anpr_r, image_path)
print(output)
# Routine Task 2 - Predict from cv2
img = cv2.imread(image_path)
output = marearts_anpr_from_cv2(anpr_d, anpr_r, img)
print(output)
# Routine Task 3 - Predict from Pillow
pil_img = Image.open(image_path)
output = marearts_anpr_from_pil(anpr_d, anpr_r, pil_img)
print(output)
#################################
The output from the ANPR will be similar to:
{
'results': [
{'ocr': 'SL593LM', 'ocr_conf': 99, 'ltrb': [819, 628, 1085, 694], 'ltrb_conf': 90}
],
'ltrb_proc_sec': 0.22,
'ocr_proc_sec': 0.15
}
{
'results': [
{'ocr': '123가4568', 'ocr_conf': 99, 'ltrb': [181, 48, 789, 186], 'ltrb_conf': 83},
{'ocr': '123가4568', 'ocr_conf': 99, 'ltrb': [154, 413, 774, 557], 'ltrb_conf': 82},
{'ocr': '123가4568', 'ocr_conf': 99, 'ltrb': [154, 601, 763, 746], 'ltrb_conf': 80},
{'ocr': '123가4568', 'ocr_conf': 99, 'ltrb': [156, 217, 773, 369], 'ltrb_conf': 80}
],
'ltrb_proc_sec': 0.23,
'ocr_proc_sec': 0.6
}
API key limits: 1000 requests per day.
User ID: marearts@public
X-API-Key: J4K9L2Wory34@G7T1Y8rt-PP83uSSvkV3Z6ioSTR!
To make an API call for EU ANPR, use the following command:
#!bin/bash
curl -X POST https://we303v9ck8.execute-api.eu-west-1.amazonaws.com/Prod/marearts_anpr_eu \
-H "Content-Type: image/jpeg" \
-H "x-api-key: your-api-key" \
-H "user-id: your-user-id" \
--data-binary "@./path/upload.jpg"
To make an API call for Korean ANPR, use the following command:
#!bin/bash
curl -X POST https://we303v9ck8.execute-api.eu-west-1.amazonaws.com/Prod/marearts_anpr \
-H "Content-Type: image/jpeg" \
-H "x-api-key: your-api-key" \
-H "user-id: your-user-id" \
--data-binary "@./path/upload.jpg"
email : hello@marearts.com
home page : https://marearts.com
blog : http://study.marearts.com
paypal : https://study.marearts.com/p/anpr-lpr-solution.html
live test : http://live.marearts.com
🙇🏻♂️ Thank you!
FAQs
MareArts ANPR (Automatic Number Plate Recognition) library
We found that marearts-anpr demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
A supply chain attack has been detected in versions 1.95.6 and 1.95.7 of the popular @solana/web3.js library.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.