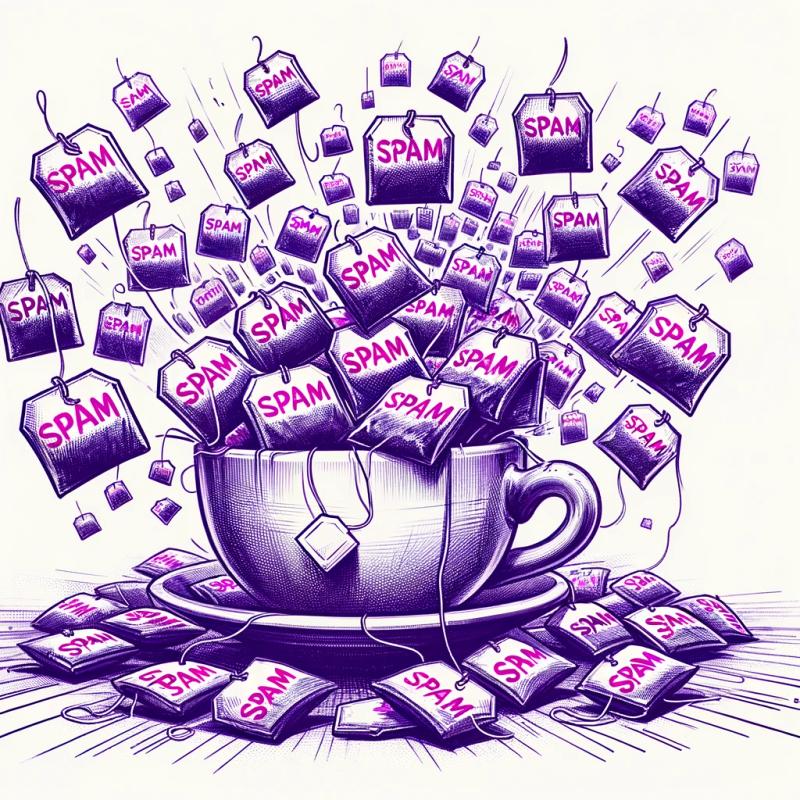
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Utility for building templated metric extraction queries that can be traversed through time.
Readme
Utility for building templated metric extraction queries that can be traversed through time.
You will need the following to run this code:
To be determined...
In order to extract a given metric, a Metric
object needs to be instantiated:
metric = Metric(
query="""
SELECT count(*) AS total
FROM `project.dataset.table`
WHERE DATETIME_TRUNC(created_datetime, DAY) = '{{ reference_time | format_date('%Y-%m-%d') }}'
""",
reader = BigQueryReader(json_credentials_path='/path/to/creds.json')
)
The query
parameter is a templated query where you can format the reference_time
datetime
object to the required format using template filters.
The reader
parameter is the object that is actually going to connect to the desired database and perform the queries.
The metric
object can now be used to fetch metrics for a given point in time as follows:
result = metric.fetch(reference_time=datetime.date(2019, 10, 21))
The result is returned as a list of dictionaries.
Jinja2 is used as the templating engine. All built in Jinja filters are thus available. Relevant custom template filters have been added though for convenience:
Specify format of datetime:
'{{ reference_time | format_date('%Y-%m-%d') }}'
Change a given datetime object by a specified number of days:
'{{ reference_time | day_delta(-7) | format_date('%Y-%m-%d') }}'
Any reader will implement the following method that is used to execute queries:
def execute(self, query) -> List[Dict[str, Any]]:
...
The underlying client is required to be authenticated with the necessary priviledges to read from the requested BigQuery tables.
If you authenticate with:
gcloud auth login
or
export GOOGLE_APPLICATION_CREDENTIALS="/path/to/keyfile.json"
then you can just instantiate your Reader
like this:
reader = BigQueryReader()
The other option is to explicitly authenticate with a service account key file:
reader = BigQueryReader(json_credentials_path='/path/to/creds.json')
Coming soon...
FAQs
Utility for building templated metric extraction queries that can be traversed through time.
We found that metric-builder demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.