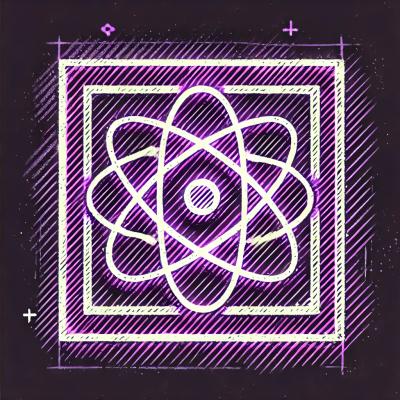
Security News
Create React App Officially Deprecated Amid React 19 Compatibility Issues
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
NexUI is a UI component library for Django, optimized for HTMX and based on Tailwind CSS. It allows you to easily add interactive elements to your Django applications while benefiting from seamless integration with HTMX for AJAX request handling.
pip install nexui
If you plan to use HTMX with your components:
pip install nexui[htmx]
Or install HTMX separately later:
pip install django-htmx
Add nexui to the list of installed apps in settings.py
:
INSTALLED_APPS = [
...
'nexui',
]
✅ Reusable UI components for Django
🔥 Smooth integration with HTMX
🎨 Based on Tailwind CSS for a modern design
📦 Easy to install and use
{% load nexui_tags %}
Check the full documentation here: Official Documentation
The button component allows you to create customizable buttons with icons and HTMX interactions.
label
: Button texttype
: Button type (button
, submit
, reset
)button_class
: Custom CSS classesicon
: Icon (supports Emoji
, Font Awesome
, and Unicode
)icon_type
: Icon type (emoji
, fa
, unicode
)icon_position
: Icon position (left
, right
)icon_size
: Icon sizeicon_color
: Icon colordisabled
: Disables the button (true
)url_name
: Django URL nameurl_params
: Dynamic parameters for the Django URLattrs
: Additional HTML
and HTMX
attributes (_hx-*
, id
, style
, data-*
, etc.){% button label="Send" %}
{% button label="Send" type="submit" button_class="bg-yellow-500 text-black" %}
{% button label="Send with HTMX" type="submit" button_class="bg-green-500" attrs="hx-post='/submit' hx-target='#result' hx-swap='innerHTML'" %}
{% button label="Dynamic URL Params" type="submit" button_class="bg-blue-500 text-white" url_name="update-user" url_params="2, tomato" attrs="hx-confirm='Are you sure?' hx-target='#result' hx-swap='innerHTML'" %}
{% button label="Download" button_class="bg-green-500" icon="⬇️" icon_position="left" icon_size="lg" %}
The input component allows you to create customizable input fields with labels, icons, and HTMX integration.
type
: Input type (text
, password
, email
, etc.)name
: Field nameid
: Field ID (default is the same as name
)value
: Default valueplaceholder
: Placeholder textcontainer_class
: CSS classes for the main containerwrapper_class
: CSS classes for the input wrapperlabel_class
: CSS classes for the labelinput_class
: CSS classes for the inputlabel
: Label textrequired
: Required field (true
/false
)disabled
: Disables the field (true
/false
)readonly
: Read-only field (true
/false
)icon
: Icon (supports Emoji
, Font Awesome
, and Unicode
)icon_type
: Icon type (emoji
, fa
, unicode
)icon_position
: Icon position (left
, right
)icon_size
: Icon sizeicon_color
: Icon colorurl_name
: Django URL name for HTMX
method
: HTTP method for HTMX (post
by default)attrs
: Additional HTML
and HTMX
attributes{% input_field %}
{% input_field name="email" label="Email" %}
{% input_field name="email" label="Email" icon="fas fa-envelope" icon_type="fa" icon_position="left" placeholder="Email" %}
{% input_field type="password" icon="🔒" %}
{% input_field name="email" label="Email" icon="fas fa-envelope" icon_type="fa" url_name="submit-form" attrs='hx-confirm="Are you okay?"' %}
{% input_field name="search" label="Search" icon="fas fa-search" icon_type="fa" icon_position="left" url_name="search_suggestions" attrs='hx-trigger="keyup changed delay:500ms" hx-target="#suggestions"' %}
{% input_field container_class="py-2 flex items-center" name="email" label="Email" label_class="ml-2 text-gray-700 font-bold" input_class="w-44 pl-10 pr-3 py-2 rounded-md border border-gray-300" icon="fas fa-envelope" icon_type="fa" icon_position="left" %}
NexUI provides pre-built authentication components that you can easily integrate into your Django applications. Below are examples of login and registration forms built with NexUI components.
Create a beautiful registration form with social authentication options:
<div class="max-w-md w-full p-6 bg-white rounded-lg shadow-md">
<h1 class="text-2xl font-bold mb-2">Create an account</h1>
<p class="text-gray-600 mb-6">Enter your email below to create your account</p>
<div class="space-y-4">
{# OAuth Buttons #}
<div class="flex gap-4 mb-4">
{% button label="GitHub" button_class="w-full border border-gray-300 bg-white hover:bg-gray-50"
icon="fa-brands fa-github fa-lg" icon_type="fa" icon_position="left" %}
{% button label="Google" button_class="w-full border border-gray-300 bg-white hover:bg-gray-50"
icon="fa-brands fa-google fa-lg" icon_type="fa" icon_position="left" %}
</div>
{# Separator #}
<div class="relative my-6">
<div class="absolute inset-0 flex items-center">
<span class="w-full border-t"></span>
</div>
<div class="relative flex justify-center text-xs uppercase">
<span class="bg-white px-2 text-gray-500">OR CONTINUE WITH</span>
</div>
</div>
{# Email Field #}
{% input_field type="email" name="register_email" label="Email"
placeholder="nexui@example.com" container_class="mb-4"
input_class="h-10" icon="fa-solid fa-envelope"
icon_type="fa" required="true" %}
{# Password Field #}
{% input_field type="password" name="register_password" label="Password"
container_class="mb-6" input_class="h-10"
icon="fa-solid fa-lock" icon_type="fa" required="true" %}
{# Submit Button #}
{% button label="Create account" type="submit"
button_class="w-full bg-gray-900 text-white hover:bg-gray-800" %}
</div>
</div>
Create a matching login form with social authentication:
<div class="max-w-md w-full p-6 bg-white rounded-lg shadow-md">
<h1 class="text-2xl font-bold mb-2">Login</h1>
<p class="text-gray-600 mb-6">Welcome back! Please enter your details</p>
<div class="space-y-4">
{# OAuth Buttons #}
<div class="flex gap-4 mb-4">
{% button label="GitHub" button_class="w-full border border-gray-300 bg-white hover:bg-gray-50"
icon="fa-brands fa-github fa-lg" icon_type="fa" icon_position="left" %}
{% button label="Google" button_class="w-full border border-gray-300 bg-white hover:bg-gray-50"
icon="fa-brands fa-google fa-lg" icon_type="fa" icon_position="left" %}
</div>
{# Separator #}
<div class="relative my-6">
<div class="absolute inset-0 flex items-center">
<span class="w-full border-t"></span>
</div>
<div class="relative flex justify-center text-xs uppercase">
<span class="bg-white px-2 text-gray-500">OR CONTINUE WITH</span>
</div>
</div>
{# Email Field #}
{% input_field type="email" name="login_email" label="Email"
placeholder="nexui@example.com" container_class="mb-4"
input_class="h-10" icon="fa-solid fa-envelope"
icon_type="fa" required="true" %}
{# Password Field #}
{% input_field type="password" name="login_password" label="Password"
container_class="mb-2" input_class="h-10"
icon="fa-solid fa-lock" icon_type="fa" required="true" %}
{# Forgot Password Link #}
<div class="flex justify-end mb-6">
<a href="#" class="text-sm text-black hover:underline">Forgot password?</a>
</div>
{# Submit Button #}
{% button label="Sign in" type="submit"
button_class="w-full bg-gray-900 text-white hover:bg-gray-800" %}
</div>
</div>
Both forms include:
You can customize these forms by:
Modifying
the Tailwind CSS classesAdding
or removing
social authentication providersCustomizing
the icons using Font Awesome classesHTMX
attributes for enhanced interactivity
Adjusting the layout and spacingThe project is currently in its early testing phase. We encourage interested users to provide feedback on the library.
If you want to contribute to NexUI or have suggestions:
Fork
the project 📌Test
the components and provide feedback 🛠️Create
an issue to share your suggestions or report problems ✅We cannot accept direct contributions at the moment, but your feedback is essential for NexUI's evolution.
This project is licensed under the MIT license. See the LICENSE file for more details.
FAQs
Library of modern UI components with optional HTMX support
We found that nexui demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.
Security News
The Linux Foundation is warning open source developers that compliance with global sanctions is mandatory, highlighting legal risks and restrictions on contributions.