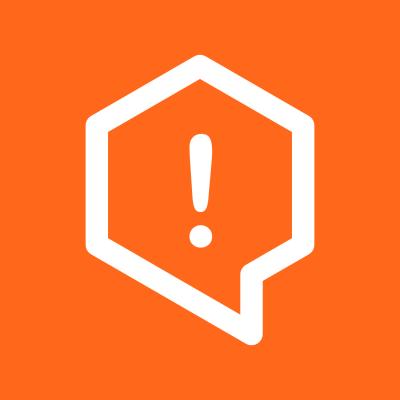
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
alternative parser for bitbake recipes
Find the full API docs here
from oelint_parser.cls_stash import Stash
# create an stash object
_stash = Stash()
# add any bitbake like file
_stash.AddFile("/some/file")
# Resolves proper cross file dependencies
_stash.Finalize()
# Use _stash.GetItemsFor() method to filter the stash
To get variables from the stash object do
from oelint_parser.cls_item import Variable
# get all variables of the name PV from all files
for x in _stash.GetItemsFor(attribute=Variable.ATTR_VAR, attributeValue="PV"):
print(x)
this returns the raw object representation
from oelint_parser.cls_item import Variable
# get all variables of the name PV from all files
for x in _stash.GetItemsFor(attribute=Variable.ATTR_VAR, attributeValue="PV"):
# raw unexpanded variable
print(x.VarValue)
# raw unexpanded variable without quotes
print(x.VarValueStripped)
# expanded variable
print(expand_term(stash, "/some/file", x.VarValueStripped))
# single items from a list
print(x.get_items())
# expanded single items from a list
print([_stash.ExpandTerm("/some/file", y, objref=x) for y in x.get_items()])
You can filter by multiple items
from oelint_parser.cls_item import Variable
# get all variables of the name PV or BPV from all files
for x in _stash.GetItemsFor(attribute=Variable.ATTR_VAR, attributeValue=["PV", "BPV"]):
# variable name
print(x.VarName)
# raw unexpanded variable
print(x.VarValue)
# raw unexpanded variable without quotes
print(x.VarValueStripped)
and you can reduce the list after the initial filtering even more
from oelint_parser.cls_item import Variable
# get all variables of the name PV or BPV from all files if the value is '1.0'
for x in _stash.GetItemsFor(attribute=Variable.ATTR_VAR, attributeValue=["PV", "BPV"]).reduce(
attribute=Variable.ATTR_VARVAL, attributeValue=["1.0"]):
# variable name
print(x.VarName)
# raw unexpanded variable -> "1.0"
print(x.VarValue)
# raw unexpanded variable without quotes -> 1.0
print(x.VarValueStripped)
but if you need copies from a wider list to smaller lists use
from oelint_parser.cls_item import Variable
_all = _stash.GetItemsFor(attribute=Variable.ATTR_VAR, attributeValue=["PV", "BPV"])
_pv = _stash.Reduce(_all, attribute=Variable.ATTR_VAR, attributeValue="PV")
_bpv = _stash.Reduce(_all, attribute=Variable.ATTR_VAR, attributeValue="BPV")
for x in _pv:
# variable name
print(x.VarName)
# raw unexpanded variable -> "1.0"
print(x.VarValue)
# raw unexpanded variable without quotes -> 1.0
print(x.VarValueStripped)
To get the effective value of a Variable after parsing you can use
from oelint_parser.cls_item import Variable
result_set = _stash.ExpandVar(attribute=Variable.ATTR_VAR, attributeValue=["PV"]):
print(result_set.get('PV'))
By default the parser will expand parsable and known inline blocks (${@oe.utils.something(...)}
) to the branch
value that would match if the programmed condition is true
.
You can invert this selection by setting negative_inline
to True
in the Stash
object.
E.g.
with some/file
being
VAR_1 = "${@bb.utils.contains('BUILDHISTORY_FEATURES', 'image', 'foo', 'bar', d)}"
and
from oelint_parser.cls_stash import Stash
# create an stash object
_stash = Stash()
# add any bitbake like file
_stash.AddFile("/some/file")
# Resolves proper cross file dependencies
_stash.Finalize()
the VAR_1
's VarValue
value would be foo
.
With
from oelint_parser.cls_stash import Stash
# create an stash object
_stash = Stash(negative_inline=True)
# add any bitbake like file
_stash.AddFile("/some/file")
# Resolves proper cross file dependencies
_stash.Finalize()
the VAR_1
's VarValue
value would be bar
.
For this library a few basic sets of constant information, such as basic package definitions, known machines and functions are needed. Those can be easily modified, in case you have additional information to add/remove/modify.
The actual database is not directly accessible by the user, but a few methods in the oelint_parse.constants.CONSTANT
class do exist.
Each of the method accepts a dictionary with the same key mapping as listed below (multilevel paths are displayed a JSON pointer)
key | type | description | getter for information |
---|---|---|---|
functions/known | list | known functions | oelint_parse.constants.CONSTANT.FunctionsKnown |
functions/order | list | preferred order of core functions | oelint_parse.constants.CONSTANT.FunctionsOrder |
images/known-classes | list | bbclasses to be known to be used in images | oelint_parse.constants.CONSTANT.ImagesClasses |
images/known-variables | list | variables known to be used in images | oelint_parse.constants.CONSTANT.ImagesVariables |
replacements/distros | list | known distro overrides | oelint_parse.constants.CONSTANT.DistrosKnown |
replacements/machines | list | known machine overrides | oelint_parse.constants.CONSTANT.MachinesKnown |
replacements/mirrors | dict | known mirrors | oelint_parse.constants.CONSTANT.MirrorsKnown |
variables/known | list | known variables | oelint_parse.constants.CONSTANT.VariablesKnown |
variables/mandatory | list | variables mandatory to a recipe | oelint_parse.constants.CONSTANT.VariablesMandatory |
variables/order | list | preferred order of variables | oelint_parse.constants.CONSTANT.VariablesOrder |
variables/protected | list | variables not to be used in recipes | oelint_parse.constants.CONSTANT.VariablesProtected |
variables/protected-append | list | variables not to be used in bbappends | oelint_parse.constants.CONSTANT.VariablesProtectedAppend |
variables/suggested | list | suggested variable in a recipe | oelint_parse.constants.CONSTANT.VariablesSuggested |
sets/base | dict | base set of variables always used for value expansion | oelint_parse.constants.CONSTANT.SetsBase |
For additional constants
from oelint_parser.constants import CONSTANTS
CONSTANTS.GetByPath('/-joined path')
will offer access
Before any contribution please run the following
python3 -m venv --clear .env
. .env/bin/activate
pip install -r requirements.txt -r requirements-dev.txt
flake8 oelint_parser/ tests/
pytest
./gendoc.sh
FAQs
Alternative parser for bitbake recipes
We found that oelint-parser demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.