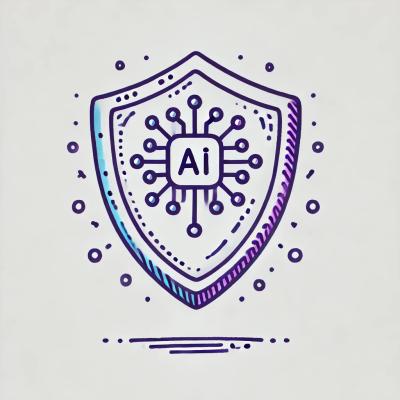
Security News
38% of CISOs Fear They’re Not Moving Fast Enough on AI
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
openmc-data-downloader
Advanced tools
A tool for selectively downloading h5 files for specified isotopes / elements from your libraries of choice
A Python package for downloading preprocessed cross section data in the h5 file format for use with OpenMC.
This package allows you to download a fully reproducible composite nuclear data library with one command.
There are several methods of obtaining complete data libraries for use with OpenMC, for example:
The package was originally conceived by Jonathan Shimwell as a means of downloading a minimal selection of data for use on continuous integration platforms. The package can also used to produce traceable and reproducible nuclear data distributions.
To install the openmc_data_downloader you need to have Python3 installed, OpenMC is also advisable if you want to run simulations using the h5 data files but not actually mandatory if you just want to download the cross sections.
pip install openmc_data_downloader
The OpenMC data downloader is able to download cross section files for isotopes from nuclear data libraries.The user specifies the nuclear data libraries in order of their preference. When an isotope is found in multiple libraries it will be downloaded from the highest preference library. This avoid duplication of isotopes and provides a reproducible nuclear data environment.
The nuclear data h5 file are downloaded from the OpenMC-data-storage repository. Prior to being added to the repository they have been automatically processed using scripts from OpenMC data repository, these scripts convert ACE and ENDF file to h5 files.
The resulting h5 files are then used in and automated test suite of simulations to help ensure they are suitable for their intended purpose.
Isotopes for downloading can be found in a variety of ways as demonstrated below.
When downloading a cross_section.xml file is automatically created and h5 files
are named with their nuclear data library and the isotope. This helps avoid
downloading files that already exist locally and the overwrite
argument
can be used to control if these files are downloaded again.
openmc_data_downloader --help
openmc_data_downloader -l FENDL-3.1d -i Li6
openmc_data_downloader -l TENDL-2019 -i Li6 Li7
openmc_data_downloader -l TENDL-2019 -e Li
openmc_data_downloader -l TENDL-2019 -e Li Si Na
openmc_data_downloader -l ENDFB-7.1-NNDC -i Be9 -d my_h5_files
openmc_data_downloader -l TENDL-2019 -e Li Si Na -i Fe56 U235
openmc_data_downloader -l TENDL-2019 -i all
openmc_data_downloader -l TENDL-2019 -i stable
openmc_data_downloader -l TENDL-2019 -m materials.xml
openmc_data_downloader -l ENDFB-7.1-NNDC TENDL-2019 -i Li6 Li7 Be9
openmc_data_downloader -l ENDFB-7.1-NNDC -e Li -p photon
openmc_data_downloader -l ENDFB-7.1-NNDC -e Li -p neutron photon
openmc_data_downloader -l ENDFB-7.1-NNDC -e Be O -s c_Be_in_BeO
When using the Python API the just_in_time_library_generator()
function
provides similar capabilities to the openmc_data_downloader
terminal
command. With one key difference being that just_in_time_library_generator()
sets the OPENMC_CROSS_SECTIONS
environmental variable to point to the
newly created cross_sections.xml by default.
import openmc
import openmc_data_downloader as odd
mat1 = openmc.Material()
mat1.add_element('Fe', 0.95)
mat1.add_element('C', 0.05)
mats = openmc.Materials([mat1])
odd.download_cross_section_data(
mats,
libraries=["FENDL-3.1d"],
set_OPENMC_CROSS_SECTIONS=True,
particles=["neutron"],
)
import openmc
import openmc_data_downloader as odd
mat1 = openmc.Material()
mat1.add_element('Fe', 0.95)
mat1.add_element('C', 0.05)
mats = openmc.Materials([mat1])
odd.download_cross_section_data(
mats,
libraries=['ENDFB-7.1-NNDC', 'TENDL-2019'],
set_OPENMC_CROSS_SECTIONS=True,
particles=["neutron"],
)
import openmc
import openmc_data_downloader as odd
my_mat = openmc.Material()
my_mat.add_element('Be', 0.5)
my_mat.add_element('O', 0.5)
my_mat.add_s_alpha_beta('Be_in_BeO')
mats = openmc.Materials([my_mat])
odd.download_cross_section_data(
mats,
libraries=['ENDFB-7.1-NNDC', 'TENDL-2019'],
set_OPENMC_CROSS_SECTIONS=True,
particles=["neutron"],
)
import openmc
import openmc_data_downloader as odd
mat1 = openmc.Material()
mat1.add_element('Fe', 0.95)
mat1.add_element('C', 0.05)
mats = openmc.Materials([mat1])
odd.download_cross_section_data(
mats,
libraries=['ENDFB-7.1-NNDC', 'TENDL-2019'],
set_OPENMC_CROSS_SECTIONS=True,
particles=["neutron", "photon"],
)
FAQs
A tool for selectively downloading h5 files for specified isotopes / elements from your libraries of choice
We found that openmc-data-downloader demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
Research
Security News
Socket researchers uncovered a backdoored typosquat of BoltDB in the Go ecosystem, exploiting Go Module Proxy caching to persist undetected for years.
Security News
Company News
Socket is joining TC54 to help develop standards for software supply chain security, contributing to the evolution of SBOMs, CycloneDX, and Package URL specifications.