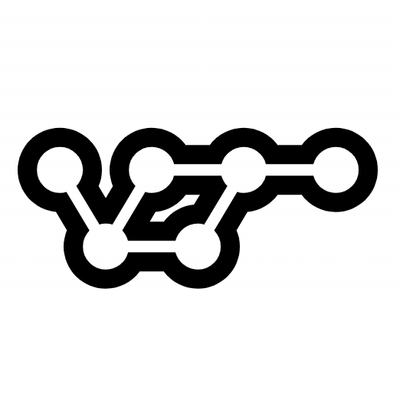
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Veem Python SDK provides an interface to call Veem Global Payments APIs (https://www.veem.com).
The Veem Python SDK provides an interface to make it easier to call Veem Global Payments APIs.
3.0.0
v1.1
pip install veem
Or
python setup.py install
Veem Python SDK utilize configuration YAML file to manage your SDK credential. Here is the sample content:
client_id: VeemTester-1234abcd
client_secret: 8djduf8e-d798-3534-afe3-123sdc3r4fe
url: https://sandbox-api.veem.com/
authorizationCode: VeemAbckeieifh
redirectUrl: http://your-veem-redirct.yourbusiness.com
To test the code locally, follow the steps below:
clientId
, clientSecret
,
authorizationCode
, and redirectUrl
(optional) in your configuration yaml.clientId
, and clientSecret
in
your configuration yaml.In order to get the access tokens from the Developer Portal;
Sign In with Veem - Sign into developer Portal .
Create an Application- Create a new application by providing the Name
, OAuth2 Redirection URLs
and Payment Status Webhooks
.
Create a Customer- Create a new customer by providing Business Name
, Country
and Primary Email
Get Credentials- Go the Application and select the Customer
and copy the Access Token
.
In order to get the access token
programmatically, get the client id, client secrets (Optional redirect url for Authorization flow).
from veem.configuration import ConfigLoader
from veem.client.authentication import AuthenticationClient
# loading SDK configuration from your yaml file
config = ConfigLoader(yaml_file='/path/to/your/configuration.yaml')
# login to Veem server with client credentials
tokenResponse= AuthenticationClient(config).getTokenFromClientCredentials()
The following example is to send invoice using Invoice Client
from veem.client.veem import VeemClient
from veem.client.requests.invoice import InvoiceRequest
# define a VeemClient Context Manager with yaml+file and auto login.
with VeemClient(yaml_file='/path/to/your/configuration.yaml',
useClientCredentials=True) as veem:
# define an InvoiceRequest
invoice = InvoiceRequest(payer=dict(type='Business',
email='username@yourbusiness.com',
firstName='Joe',
lastName='Doe',
businessName='Your Business Inc.',
countryCode='US',
phoneCountryCode='1',
phone='02222222222'),
amount=dict(number=50, currency='USD'))
# create an invoice
sentInvoice = veem.inoviceClient.create(invoice)
More Examples can be found under examples folder
FAQs
Veem Python SDK provides an interface to call Veem Global Payments APIs (https://www.veem.com).
We found that oveem demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.