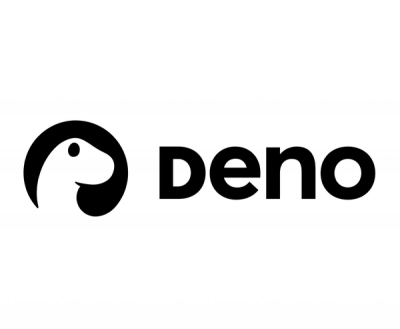
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
This Python module implements an event handling framework (`EventEmitter`) with support for prioritization (`PriorityEvent`), event filtering, and processing using threading, queues (`queue` module), heap operations (`heapq` module), and logging (`logging` module) capabilities.
This Python module implements an event handling framework (EventEmitter
) that supports prioritization (PriorityEvent
), event filtering, and processing using threading, queues (queue
module), heap operations (heapq
module), and logging (logging
module) capabilities.
Defines an interface for handling events.
Extends EventHandler
and handles notifications with specific ID
and Message
attributes.
Represents a notification entity with attributes ID
, Message
, and CreatedAt
.
Represents an event with its associated priority for queueing and processing.
Manages event registration, emission, and handling. Features include:
main.py
import threading
import time
import queue
import logging
from pyeventhub import EventEmitter, NotificationHandler, Notification
if __name__ == "__main__":
logging.basicConfig(level=logging.INFO)
# Example usage
emitter = EventEmitter(logging=True)
# Register notification handlers
handler1 = NotificationHandler(name="EmailHandler")
handler2 = NotificationHandler(name="SMSHandler")
emitter.on_event("email_notification", handler1)
emitter.on_event("sms_notification", handler2)
# Registering listeners with filters
def email_filter(data):
if isinstance(data, Notification):
return data.ID > 0 # Example filter: process only notifications with ID > 0
return False
chEmail = emitter.on("email_notification", email_filter)
chSMS = emitter.on("sms_notification")
# Simulate sending notifications with priority
def send_notifications():
emitter.emit_with_context(None, "email_notification", Notification(ID=1, Message="New email received", CreatedAt=time.time()), 2) # Higher priority
emitter.emit_with_context(None, "sms_notification", Notification(ID=2, Message="You have a new SMS", CreatedAt=time.time()), 1) # Lower priority
# Handle notifications asynchronously
def handle_notifications():
while True:
if not chEmail.empty():
notification = chEmail.get()
print("Received email notification")
handler1.handle(notification)
if not chSMS.empty():
notification = chSMS.get()
print("Received SMS notification")
handler2.handle(notification)
send_thread = threading.Thread(target=send_notifications)
handle_thread = threading.Thread(target=handle_notifications)
send_thread.start()
handle_thread.start()
# Allow some time for notifications to be processed
time.sleep(2)
# Clean up
emitter.close()
FAQs
This Python module implements an event handling framework (`EventEmitter`) with support for prioritization (`PriorityEvent`), event filtering, and processing using threading, queues (`queue` module), heap operations (`heapq` module), and logging (`logging` module) capabilities.
We found that pyeventhub demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.