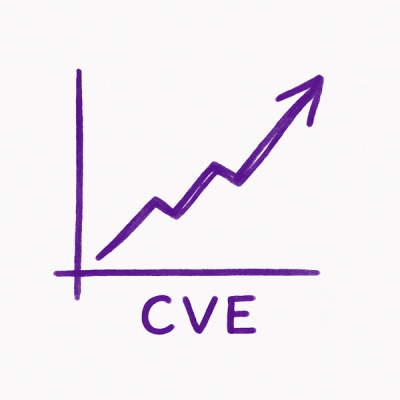
Security News
New CVE Forecasting Tool Predicts 47,000 Disclosures in 2025
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.
Pypartial is a simple Python library that allows the use of partial function application notation in native Python. It is extremely useful when trying to write concise and clear code, and extends the ease offered by Python's functional programming features.
Example:
import pypartial #use <_ notation to partial apply functions
binary = int<_(base=2) #you can pass keyword arguemnts
print(binary('101010100101'))
import random
dice=random.randint<_(1, 6) #you can also pass non-keywords
print(dice())
print(dice())
up_to_ten = range<_(_, 11) #you can leave args blank with '_'
print(list(up_to_ten(5)))
import operator
double = operator.mul<_(2)
print(double(7))
class Foo(object):
def bar(self, a, b):
print([a,b])
def egg(self, a, b, c=9):
print([a,b,c])
foo=Foo()
bar7 = foo.bar<_(_, b=7) #works with methods too
bar7(2)
egg1 = foo.egg<_(_, 5)
egg2 = egg1<_(c=9) #you can partially apply multiple times
egg2(6)
print(egg2) #nice string representation
FAQs
Use partial function application in Python
We found that pypartial demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.