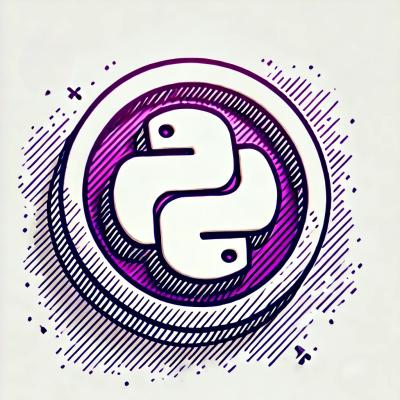
Security News
Introducing the Socket Python SDK
The initial version of the Socket Python SDK is now on PyPI, enabling developers to more easily interact with the Socket REST API in Python projects.
python-barcode-spreadSheet
Advanced tools
A simple Python tool to generate bar-codes and programmatically insert them into Google Spreadsheets for printing.
A simple Python tool to generate bar-codes and programmatically insert them into Google Spreadsheets for printing.
Download and get the two plugins up and running before proceeding.
Import the script::
import BarcodePythonScript as bps
Logging into the spreadsheet client::
client=bps.spreadsheet_login(your_google_email, your_google_password)
Generating bar-code::
bps.BarcodeGen(barcode_type, barcode_gen_value, image_name)
where 'barcode_type' is the type of bar-code desired, 'barcode_gen_value' is the value to be used with the bar-code and 'image_name' is the desired name of the bar-code image PNG file.
Types of bar-codes:
Code 39, PZN, EAN-13, EAN-8, JAN, ISBN-13, ISBN-10, ISSN, UPC-A
Writing bar-code image to spreadsheet::
bps.BarcodeWrite(client, spreadsheet_key, image_url, cell_id, sheet_id)
where 'client' is the spreadseet client, 'spreadsheet_key' is the key of the spreadsheet found in its URL. For example, in 'docs.google.com/spreadsheet/ccc?key=0AslEqHKkTxw1dGFSQmpyQnBKWXhYelRRQ3hldjFWS0E&usp=sharing#gid=14', the key is '0AslEqHKkTxw1dGFSQmpyQnBKWXhYelRRQ3hldjFWS0E'.
image_url is the absolute url of the image saved above, cell_id is the address of the spreadsheet cell where the bar-code is to be inserted (A21, F34, C6 etc) and sheet_id is the index of the sheet (0 being the first).
FAQs
A simple Python tool to generate bar-codes and programmatically insert them into Google Spreadsheets for printing.
We found that python-barcode-spreadSheet demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The initial version of the Socket Python SDK is now on PyPI, enabling developers to more easily interact with the Socket REST API in Python projects.
Security News
Floating dependency ranges in npm can introduce instability and security risks into your project by allowing unverified or incompatible versions to be installed automatically, leading to unpredictable behavior and potential conflicts.
Security News
A new Rust RFC proposes "Trusted Publishing" for Crates.io, introducing short-lived access tokens via OIDC to improve security and reduce risks associated with long-lived API tokens.