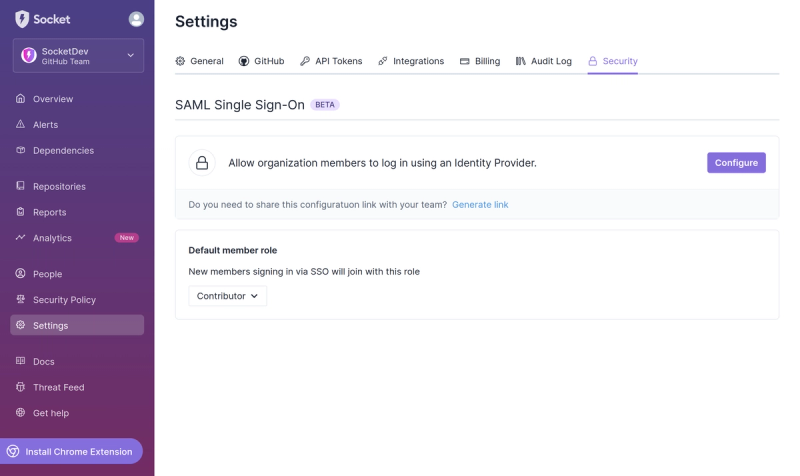
Product
Introducing SSO
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
python-return-youtube-dislike
A simple Python library to interact with the Return YouTube Dislike API
Readme
A simple Python library to work with the Return YouTube Dislike API
PyPi page: https://pypi.org/project/python-return-youtube-dislike/
To install the package, run the following command:
pip install python-return-youtube-dislike
Once installed, import it into your script with the following code:
from pythonryd import *
All functions in this library take in a parameter called video_id. This is the part after the watch?v= of a YouTube URL.
For the YouTube URL https://www.youtube.com/watch?v=SvcttIw9qBM the video_id is SvcttIw9qBM
The package contains the following commands:
ryd_getvideoinfo(video_id)
video = ryd_getvideoinfo("SvcttIw9qBM") # Using SvcttIw9qBM as an example
print(video)
Response:
{'id': 'SvcttIw9qBM', 'dateCreated': '2022-01-23T04:44:33.634409Z', 'likes': 645, 'dislikes': 167, 'rating': 4.177339901477833, 'viewCount': 300777, 'deleted': False}
ryd_getvideolikes(video_id)
likes = ryd_getvideolikes("SvcttIw9qBM") # Using SvcttIw9qBM as an example
print(likes)
Response:
645
ryd_getvideodislikes(video_id)
dislikes = ryd_getvideodislikes("SvcttIw9qBM") # Using SvcttIw9qBM as an example
print(dislikes)
Response:
167
ryd_getvideorating(video_id)
rating = ryd_getvideorating("SvcttIw9qBM") # Using SvcttIw9qBM as an example
print(rating)
Response:
4.177339901477833
ryd_getvideoviews(video_id)
views = ryd_getvideoviews("SvcttIw9qBM") # Using SvcttIw9qBM as an example
print(views)
Response:
300777
ryd_isvideodeleted(video_id)
deleted = ryd_isvideodeleted("SvcttIw9qBM") # Using SvcttIw9qBM as an example
print(deleted) # Returns True if deleted, False if not deleted (It's a string, not a bool)
Response:
False
ryd_getvideodatecreated(video_id)
dateCreated = ryd_getvideodatecreated("SvcttIw9qBM") # Using SvcttIw9qBM as an example
print(dateCreated)
Response:
2022-01-23T04:44:33.634409Z
FAQs
A simple Python library to interact with the Return YouTube Dislike API
We found that python-return-youtube-dislike demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.