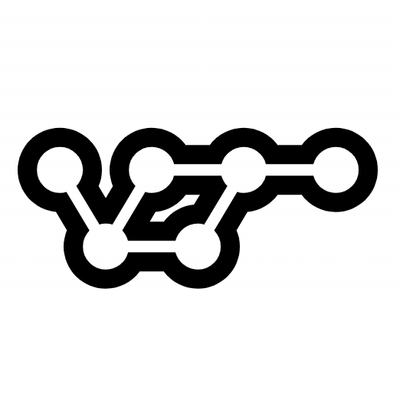
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
A Python module for handling rectangular regions of interest (ROI) in images.
To install the roi-rectangle
module, use:
pip install roi-rectangle
Here's an example of how to use the RoiRectangle
module:
from roi_rect import RoiRectangle
import numpy as np
import matplotlib.pyplot as plt
# Create a test image
test_image = np.random.random((100, 100))
# Create an instance of RoiRectangle
roi = RoiRectangle(x1=20, y1=30, x2=70, y2=80)
# Print ROI information
print("Initial ROI:")
print(roi)
# Get the center coordinate of the ROI
print("Center Coordinate:", roi.center)
# Get the width and height of the ROI
print("Width:", roi.width)
print("Height:", roi.height)
# Move the ROI to a new center
new_center = (50, 50)
roi.move_to_center(new_center)
print("\nAfter Moving to Center:")
print(roi)
# Resize the ROI
new_width, new_height = 30, 40
roi.resize(new_width, new_height)
print("\nAfter Resizing:")
print(roi)
# Slice the ROI region from the test image
roi_slice = roi.slice(test_image)
print("\nROI Slice:")
print(roi_slice)
# Visualize the original image and the sliced ROI
plt.figure(figsize=(8, 4))
plt.subplot(1, 2, 1)
plt.title('Original Image')
plt.imshow(test_image, cmap='gray')
plt.subplot(1, 2, 2)
plt.title('ROI Slice')
plt.imshow(roi_slice, cmap='gray')
plt.gca().add_patch(plt.Rectangle((roi.x1, roi.y1), roi.width, roi.height, linewidth=2, edgecolor='r', facecolor='none'))
plt.show()
This project is licensed under the MIT License.
FAQs
A module for handling rectangular regions of interest (ROI) in images.
We found that roi-rectangle demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.