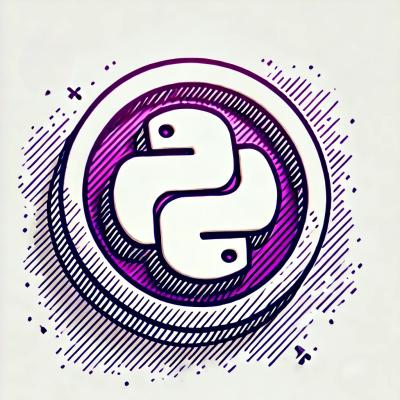
Security News
Introducing the Socket Python SDK
The initial version of the Socket Python SDK is now on PyPI, enabling developers to more easily interact with the Socket REST API in Python projects.
slinkedList is a module to automate the operations that can be performed using linked list such as creating a new node, adding a new element,inserting an element at the head node, tail node and required location, merging the linked lists,sorting,duplicates removing and deleting the elements etc.
The source code is currently hosted on GitHub at: here
Use the package manager pip to install slinkedList.
slinkedList can apply the following operations:
slinkedList requires Python 3 .
To install using pip, use
pip install slinkedList
import slinkedList as ll
creating a empty linked list
empty_list= ll.SingleLinkedList()
temp = ll.SingleLinkedList([1,2,3,4])
Some most commonly used operations
myList=ll.SingleLinkedList([1,2,3,4,5,6,7,8]) #creating ll with data
myList.addToEnd(12) #adds 12 to the ending
# printing Length
print(myList.length())
# printing indexes of given elements
print(myList.index([2,3,4]))
# printing elements at a particular indexes
print(myList.atIndex([3,1]))
# removing an element
print(myList.removeItem([12,1]))
# removing elements from a particular positions
myList.removeAtPosition([3,2,4])
#returns a list with descending order
new_list=myList.new_sorted(reverse=True)
1)
import slinkedList as ll
# creating LinkedList
m= ll.SingleLinkedList(data=[1,2,3,4])
n= ll.SingleLinkedList(data=[5,6,7])
o=m+n
print(o)
returns,
sllist([1, 2, 3, 4, 5, 6, 7])
2)
import slinkedList as ll
m= ll.SingleLinkedList(data=[1,2,3,4,5,6,7,8])
print(m[:4].toString("->"))
returns,
1->2->3->4
3)
import slinkedList as ll
m= ll.SingleLinkedList(data=[1,2,3,4])
print(reversed(m))
returns,
sllist([4, 3, 2, 1])
The official documentation is hosted on Drive : doc
Pull requests are welcome. For major changes, please open an issue first to discuss what you would like to change.
Please make sure to update tests as appropriate.
For any questions, issues, bugs, and suggestions please visit here
FAQs
In this package we simplifying the linked list concepts with useful functions
We found that slinkedList demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The initial version of the Socket Python SDK is now on PyPI, enabling developers to more easily interact with the Socket REST API in Python projects.
Security News
Floating dependency ranges in npm can introduce instability and security risks into your project by allowing unverified or incompatible versions to be installed automatically, leading to unpredictable behavior and potential conflicts.
Security News
A new Rust RFC proposes "Trusted Publishing" for Crates.io, introducing short-lived access tokens via OIDC to improve security and reduce risks associated with long-lived API tokens.