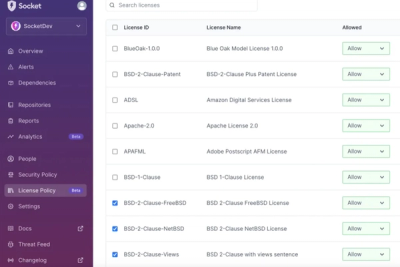
Product
Introducing License Enforcement in Socket
Ensure open-source compliance with Socket’s License Enforcement Beta. Set up your License Policy and secure your software!
Standlib 🐍
This project is a set of pre-built modules, functions and classes that were made to make your life easier when programming in Python, it works like a Swiss army knife.
Version:
Instalation:
pip install pystdlib
and then you can use it without any problems.Purpose: The Double class provides a custom implementation for double-precision floating-point numbers in Python, offering greater control over precision and formatting than the built-in float type.
Attributes:
precision (int): Specifies the number of decimal places to maintain. Methods:
Features:
Customizable precision: Control the number of decimal places to retain. Arithmetic operations: Supports addition, subtraction, multiplication, division, modulo, exponentiation, and floor division. String formatting: Provides flexible formatting options. Default precision: Sets a global default precision for all Double objects. Internal Implementation:
Stores values as strings to maintain exact precision. Implements custom arithmetic operations for accurate calculations. Handles edge cases like division by zero and large numbers. Benefits:
Improved accuracy: Avoids floating-point errors common in standard float types. Flexibility: Customize precision to suit specific use cases. Control: Offers fine-grained control over number representation. Use Cases:
Financial calculations: Where precise calculations are essential. Scientific computing: For applications requiring high-precision numbers. Custom data types: As a building block for more complex data structures.
FAQs
A custom standard library module for Python
We found that standlib demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Ensure open-source compliance with Socket’s License Enforcement Beta. Set up your License Policy and secure your software!
Product
We're launching a new set of license analysis and compliance features for analyzing, managing, and complying with licenses across a range of supported languages and ecosystems.
Product
We're excited to introduce Socket Optimize, a powerful CLI command to secure open source dependencies with tested, optimized package overrides.