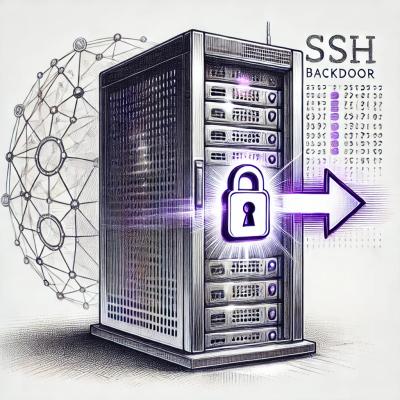
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Simple Web API controller framework for FastAPI
To install this package, use pip:
pip install webapicontrollers
from fastapi import FastAPI, HTTPException, Request
from fastapi.responses import JSONResponse
from src.webapicontrollers import APIController, Get, Post, Patch, Delete, Put, RoutePrefix
@RoutePrefix('/test')
class TestController(APIController):
def __init__(self, app: FastAPI) -> None:
super().__init__(app)
@Get('/')
async def get(self) -> dict:
return {"method": "GET", "path": "/"}
@Get('/400')
async def get_bad_request(self) -> dict:
raise HTTPException(status_code=400, detail="Bad Request")
@Get('/401')
async def get_not_authorized(self) -> dict:
raise HTTPException(status_code=401, detail="Not Authorized")
@Get('/403')
async def get_forbidden(self) -> dict:
raise HTTPException(status_code=403, detail="Forbidden")
@Get('/404')
async def get_not_found(self) -> dict:
raise HTTPException(status_code=404, detail="Not Found")
@Get('/405')
async def get_method_not_allowed(self) -> dict:
raise HTTPException(status_code=405, detail="Method Not Allowed")
@Get('/500')
async def get_internal_server_error(self) -> dict:
raise HTTPException(status_code=500, detail="Internal Server Error")
@Get('/{arg}')
async def get_with_arg(self, arg) -> dict:
return {"method": "GET", "path": "/", "arg": arg}
@Post('/')
async def post(self) -> dict:
return {"method": "POST", "path": "/"}
@Post('/{arg}')
async def post_with_arg(self, arg) -> dict:
return {"method": "POST", "path": "/", "arg": arg}
@Put('/')
async def put(self) -> dict:
return {"method": "PUT", "path": "/"}
@Put('/{arg}')
async def put_with_arg(self, arg) -> dict:
return {"method": "PUT", "path": "/", "arg": arg}
@Patch('/')
async def patch(self) -> dict:
return {"method": "PATCH", "path": "/"}
@Patch('/{arg}')
async def patch_with_arg(self, arg) -> dict:
return {"method": "PATCH", "path": "/", "arg": arg}
@Delete('/')
async def delete(self) -> dict:
return {"method": "DELETE", "path": "/"}
@Delete('/{arg}')
async def delete_with_arg(self, arg) -> dict:
return {"method": "DELETE", "path": "/", "arg": arg}
def bad_request(self, request: Request, exc: HTTPException) -> JSONResponse:
# Custom handling code
return super().bad_request(request, exc)
def not_authorized(self, request: Request, exc: HTTPException) -> JSONResponse:
# Custom handling code
return super().not_authorized(request, exc)
def forbidden(self, request: Request, exc: HTTPException) -> JSONResponse:
# Custom handling code
return super().forbidden(request, exc)
def not_found(self, request: Request, exc: HTTPException) -> JSONResponse:
# Custom handling code
return super().not_found(request, exc)
def method_not_allowed(self, request: Request, exc: HTTPException) -> JSONResponse:
# Custom handling code
return super().method_not_allowed(request, exc)
def internal_server_error(self, request: Request, exc: HTTPException) -> JSONResponse:
# Custom handling code
return super().internal_server_error(request, exc)
app = FastAPI()
TestController(app)
If you overide the handler methods such as not_found etc. in more than one cotnroller only one handler will be registered on a last one wins basis. Implementing a per route prefix handling system is on the to do list.
If you create a base controller class and then overide it's methods in a derived class the path needs to be the same in both methods. If you don't do this then FastAPI gets confused about which handler maps to which path.
This project is in a very early state and might not be very useful to anyone yet. There is no support avilable, use at your own risk.
This project is licensed under the MIT License.
FAQs
A basic API controller framework built on top of FastAPI
We found that webapicontrollers demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.