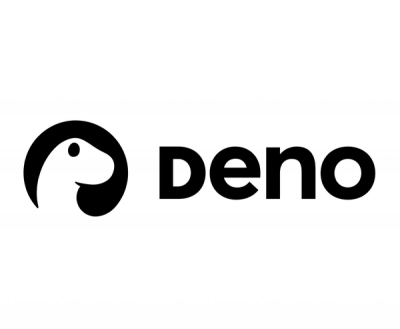
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
ALPHA VERSION
Warning: Some features may be unavailable or unstable. The client is developed by one developer in his spare time. If you are not satisfied with this, you can always add or change the client by creating a pull request.
Probably, this REST API Client will make your life with Yandex.Cloud a little easier.
pip3 install yandex-cloud-client
git clone https://github.com/akimrx/python-yc-client --recursive
cd python-yc-client
make install
or
git clone https://github.com/akimrx/python-yc-client --recursive
cd python-yc-client
python3 setup.py install
The first step is to import required client of the Yandex.Cloud Services.
Each client of a Yandex.Cloud service inherits authorization from the base client, which supports three methods:
from yandex_cloud_client import ComputeClient
client = ComputeClient(oauth_token='YOUR_OAUTH_TOKEN')
from yandex_cloud_client import ComputeClient
client = ComputeClient(iam_token='YOUR_IAM_TOKEN')
import json
from yandex_cloud_client import ComputeClient
with open('/path/to/key.json', 'r') as infile:
credentials = json.load(infile)
client = ComputeClient(service_account_key=credentials)
You can get
key.json
from Yandex Cloud CLI:yc iam key create --service-account-name my-robot -o my-robot-key.json
from yandex_cloud_client import ComputeClient
compute = ComputeClient(oauth_token='YOUR_OAUTH_TOKEN')
def show_instance_and_restart(instance_id):
instance = compute.instance(instance_id, metadata=True)
print('Name:', instance.name)
print('Cores:', instance.resources.cores)
print('Memory:', instance.resources.memory)
print('SSH-keys:', instance.metadata.ssh_keys)
if instance.running:
operation = instance.restart()
if operation.completed:
print(f'Instance {instance.name} restarted!')
print('Current instance state:', instance.status)
def boot_disk_snapshot(instance_id):
instance = compute.instance(instance_id)
if not instance.stopped: # also, you can use instance.status != 'STOPPED'
print('Stopping instance..')
instance.stop()
print('Creating snapshot for boot disk..')
instance.boot_disk.create_snapshot()
print('Starting instance without awaiting complete.')
instance.start(await_complete=False)
if __name__ == '__main__':
show_instance_and_restart('YOUR_INSTANCE_ID')
boot_disk_snapshot('YOUR_INSTANCE_ID')
See more examples here
This library uses the logging
module.
Example of usage:
import logging
logging.basicConfig(level=logging.INFO,
format='[%(levelname)s] %(message)s')
logger = logging.getLogger(__name__)
The client was written under the inspiration of architecture design:
FAQs
PUnofficial Yandex.Cloud REST API Client
We found that yandex-cloud-client demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.