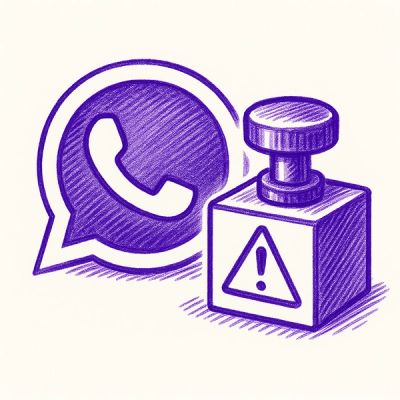
Research
/Security News
Malicious npm Packages Target WhatsApp Developers with Remote Kill Switch
Two npm packages masquerading as WhatsApp developer libraries include a kill switch that deletes all files if the phone number isn’t whitelisted.
Small childly ORM for Redis
Add this line to your application's Gemfile:
gem 'active_redis', '0.0.9'
And then execute:
$ bundle
Or install it yourself as:
$ gem install active_redis
In model you may add attributes with next types: string, integer, time
class Article < ActiveRedis::Base
attributes title: :string, link: :string
end
By class method attributes you must define own attributes.
By using accessible methods
article = Article.new
article.title = "Some title"
article.link = "http://someblog.com/1"
p article.title # => Some title
p article.link # => http://someblog.com/1
Or by attributes method
article = Article.new
article.attributes = {title: "Some title", link: "http://someblog.com/1"}
p article.attributes # => {title: "Some title", link: "http://someblog.com/1"}
p article.title # => Some title
p article.link # => http://someblog.com/1
ActiveRedis::Base inherited model respond to all CRUD method such as create, update, destroy and save
article = Article.new(title: "Some title", link: "http://someblog.com/1")
article.save # => true
another_article = Article.create(title: "Another title", link: "http://someblog.com/2") # => true
article.update(title: "New article title") # => true
p article.title # => New article title
another_article.destroy
Article.destroy_all
p Article.count # => 0
You may find 'row' by it's id
Article.find(1) # => [#<Article:0x007fec2526f4f8 @updated_at="1382608780", @link="http://someblog.com/1", @id="1", @title="Some title", @created_at="1382608780">]
Also gem add support for some aggregation functions like sum, min, max, pluck
class Article < ActiveRedis::Base
attributes link: :string, title: :string, views: :integer
end
Article.create(views: 1000, link: "http://someblog.com/1", title: "Title article")
Article.create(views: 3000, link: "http://someblog.com/2", title: "Title article")
From version 0.0.2 you are able to search item by multiple attributes using method where
Article.where(title: "Title article", views: 1000)
In version 0.0.4 is implemented model generator
rails g active_redis:model ModelName attribute1 attribute2
For example:
Sergeys-MacBook-Pro-2:test_active_redis sergey$ rails g active_redis:model User name:string city:string
create app/models/user.rb
The result is app/models/user.rb with stub content
class User < ActiveRedis::Base
attributes name: :string, city: :string
end
Method chaining. Now you may calling where, order, limit something like this:
Article.where(title: "Article title").where(views: 1000).order(title: :asc).limit(per_page: 20, page: 3)
Using aggregation functions with method chaining functional.
git checkout -b my-new-feature
)git commit -am 'Add some feature'
)git push origin my-new-feature
)FAQs
Unknown package
We found that active_redis demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
Two npm packages masquerading as WhatsApp developer libraries include a kill switch that deletes all files if the phone number isn’t whitelisted.
Research
/Security News
Socket uncovered 11 malicious Go packages using obfuscated loaders to fetch and execute second-stage payloads via C2 domains.
Security News
TC39 advances 11 JavaScript proposals, with two moving to Stage 4, bringing better math, binary APIs, and more features one step closer to the ECMAScript spec.