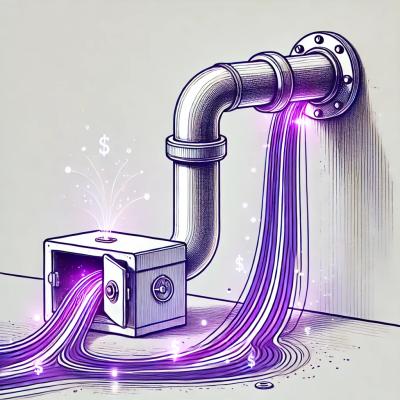
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
This is an unofficial Ruby wrapper for the Kucoin exchange REST and WebSocket APIs.
#match?
The gem itself should work find with any Ruby 2.x and higher and perhaps 1.9.x, but has certainly not been tested. YMMV!Add this line to your application's Gemfile:
gem 'kucoin-api'
And then execute:
$ bundle
Or install it yourself as:
$ gem install kucoin-api
Basic implementation of REST API
Basic implementation of WebSocket API
Exception handling with responses
High level abstraction
Require Kucoin API:
require 'kucoin/api'
Create a new instance of the REST Client:
# If you only plan on touching public API endpoints, you can forgo any arguments
client = Kucoin::Api::REST.new
# Otherwise provide an api_key as keyword arguments
client = Kucoin::Api::REST.new api_key: 'your.api_key', api_secret: 'your.api_secret', api_passphrase: 'your.api_passphrase'
# You can provide a sandbox as argument to change into Sandbox environment
client = Kucoin::Api::REST.new sandbox: true
Environment | BaseUri |
---|---|
Production DEFAULT | https://openapi-v2.kucoin.com |
Sandbox | https://openapi-sandbox.kucoin.com |
ALTERNATIVELY, set your API key in exported environment variable:
export KUCOIN_API_KEY=your.api_key
export KUCOIN_API_SECRET=your.api_secret
export KUCOIN_API_PASSPHRASE=your.api_passphrase
Then you can instantiate client without parameters as in first variation above.
Create various requests:
# Others / Time / Server Time
client.other.timestamp
# => 1554213599244
# Currencies Plugin / List exchange rate of coins
client.currency.all
# => {"rates"=>{"TRAC"=>{"CHF"=>0.02, "HRK"=>0.14...}}
# Public Market Data / Tick
client.market.tick(symbol: 'KCS-BTC')
# => {"coinType"=>"KCS", "trading"=>true, "symbol"=>"KCS-BTC", "lastDealPrice"=>0.00016493,
# "buy"=>0.00016493, "sell"=>0.00016697, "change"=>2.41e-06, "coinTypePair"=>"BTC", "sort"=>0,
# "feeRate"=>0.001, "volValue"=>19.92555026, "high"=>0.00016888, "datetime"=>1546427934000,
# "vol"=>120465.9024, "low"=>0.000161, "changeRate"=>0.0148
# }
# Trading / Create an order
client.order.create 'KCS-BTC', type: 'BUY', price: 0.000127, amount: 22
# => { "orderOid": "596186ad07015679730ffa02" }
# Assets Operation / Get coin deposit address
client.account.wallet_address('KCS')
# => {
# "oid": "598aeb627da3355fa3e851ca",
# "address": "598aeb627da3355fa3e851ca",
# "context": null,
# "userOid": "5969ddc96732d54312eb960e",
# "coinType": "KCS",
# "createdAt": 1502276446000,
# "deletedAt": null,
# "updatedAt": 1502276446000,
# "lastReceivedAt": 1502276446000
# }
Required and optional parameters, as well as enum values, can currently be found on the Kucoin Apiary Page. Parameters should always be passed to client methods as keyword arguments in snake_case form. symbol, when a required parameter is simply passed as first parameter for most API calls.
REST endpoints are in order as documented on the Kucoin Apiary page (linked above).
Endpoints are accessible by following resourceful structure given in Kucoin API documentation. For example - user.accounts
is for endpoints given in Kucoin API documentation under "User/Accounts".
The following lists only the method names, aliases (if any) and parameters of the methods to access endpoints.
For the most part, method names follow RESTful action names and alias method follows the title/name given in Kucoin API documentation.
There were some deviations where there would otherwise be name clashes/overloading.
# List Accounts
user.accounts.list options={}
# Get an Account
user.accounts.get account_id
# Create an Account
user.accounts.create currency, type
# Get Account Ledgers
user.accounts.ledgers account_id, options={}
# Get Holds
user.accounts.holds account_id
# Inner Transfer
user.accounts.inner_transfer client_oid, pay_account_id, rec_account_id, amount
# Create Deposit Address
user.deposits.create currency
# Get Deposit Address
user.deposits.get currency
# Get Deposit List
user.deposits.list options={}
# Get Withdrawals List
user.withdrawals.list options={}
# Get Withdrawal Quotas
user.withdrawals.quotas currency
# Apply Withdraw
user.withdrawals.apply currency, address, amount, options={}
# Cancel Withdrawal
user.withdrawals.cancel withdrawal_id
# Place a new order
trade.orders.place client_oid, side, symbol, options={}
# Cancel an order
trade.orders.cancel order_id
# Cancel all orders
trade.orders.cancel_all options={}
# List Orders
trade.orders.list options={}
# Recent Orders
trade.orders.recent
# Get an order
trade.orders.get order_id
# List Fills
trade.fills.list
# Recent Fills
trade.fills.recent
# Get Symbols List
markets.all
# Get Ticker
markets.stats symbol
# Get All Tickers
markets.tickers.all
# Get 24hr Stats
markets.tickers.inside symbol
# Get Market List
markets.symbols.all options={}
# Get Part Order Book(aggregated)
markets.order_book.part symbol, depth
# Get Full Order Book(aggregated)
markets.order_book.full_aggregated symbol
# Get Full Order Book(atomic)
markets.order_book.full_atomic symbol
# Get Trade Histories
markets.histories.trade symbol
# Get Klines
markets.histories.klines symbol, type, options={}
# Get Currencies
markets.currencies.all
# Get Currency Detail
markets.currencies.detail currency
# Get Fiat Price
markets.currencies.fiat options= {}
# Server Time
other.timestamp
Create a new instance of the WebSocket Client:
# If you only plan on touching public topics
client = Kucoin::Api::Websocket.new
# Changing the rest_client argument for different authentication
client = Kucoin::Api::Websocket.new rest_client: Kucoin::Api::REST.new(sendbox: true)
Subscribe various topics:
# Public Channels / Symbol Ticker
methods = { message: proc { |event| puts event.data } }
client.ticker symbols: 'ETH-BTC', methods: methods
# => {"id":"259173795477643264","type":"ack"}
# => {"data":{"sequence":...}, "subject":"trade.ticker","topic":"/market/ticker:ETH-BTC","type":"message"}
# => {"data":{"sequence":...}, "subject":"trade.ticker","topic":"/market/ticker:ETH-BTC","type":"message"}
# => ...
# Private Channels / Stop order received event
client.stop_order_received_event symbols: 'BTC-USDT', methods: methods
# => {"id":"259173795477643264","type":"ack"}
# => {"data":{"sequence":...}, "subject":"trade.l3received","topic":"/market/level3:BTC-USDT","type":"message"}
# => {"data":{"sequence":...}, "subject":"trade.l3received","topic":"/market/level3:BTC-USDT","type":"message"}
# => ...
Multiplex:
channel = nil
message = proc do |event|
puts event.data
data = JSON.parse(event.data)
if data['type'] == 'ack' && data['id'] == '1222'
Kucoin::Api::Websocket.subscribe(channel: channel, params: { topic: "/market/ticker:ETH-BTC", tunnelId: 'bt1' })
end
end
methods = { message: message }
channel = client.multiplex stream: { newTunnelId: 'bt1', id: '1222' }, methods: methods
# => {"id":"1222","type":"ack"}
# => {"tunnelId":"bt1","id":"170022900","type":"ack"}
# => {"data":{"sequence":...},"subject":"trade.ticker","tunnelId":"bt1","topic":"/market/ticker:ETH-BTC","type":"message"}
# => {"data":{"sequence":...},"subject":"trade.ticker","tunnelId":"bt1","topic":"/market/ticker:ETH-BTC","type":"message"}
# Using the one physical connection - `channel`,
# you could open different multiplex tunnels to subscribe different topics for different data.
All subscription topic method will expect "methods" in argument(As shown above). It's The Hash which contains the event handler methods to pass to the WebSocket client methods. Proc is the expected value of each event handler key. Following are list of expected event handler keys.
Subscribe topics are in order as documented on the Kucoin Apiary page (linked above).
# Symbol Ticker
ticker symbols:, methods:
# All Symbols Ticker
all_ticker methods:
# Symbol Snapshot
# Market Snapshot
snapshot symbol:, methods:
# Level-2 Market Data
level_2_market_data symbols:, methods:
# Match Execution Data
match_execution_data symbols:, methods:, private_channel: false
# Full MatchEngine Data(Level 3)
full_match_engine_data symbols:, methods:, private_channel: false
# Stop order received event
# Stop order activate event
stop_order_received_event symbols:, methods:
# Account balance notice
balance methods:
Bug reports and pull requests are welcome on GitHub at https://github.com/mwlang/kucoin-api.
The inspiration for architectural layout of this gem comes nearly one-for-one from the Binance gem by craysiii.
The gem is available as open source under the terms of the MIT License.
FAQs
Unknown package
We found that kucoin-api demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.