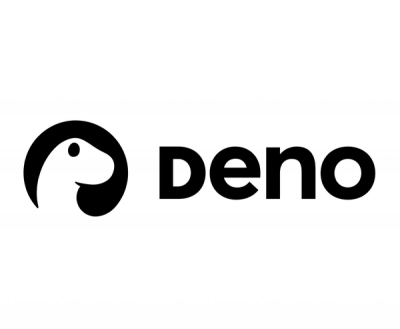
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
= FbGraph
A full-stack Facebook Graph API wrapper in Ruby.
== Installation
gem install fb_graph
== Resources
== Examples
Now FbGraph supports all objects listed here: http://developers.facebook.com/docs/reference/api/ Almost all connections for each object are also supported. (Private message connections are not supported yet)
Plus, you can play an Rails sample app here. http://fbgraphsample.heroku.com/
=== GET
==== Basic Objects
user = FbGraph::User.me(ACCESS_TOKEN)
user = FbGraph::User.fetch('matake') user.name # => 'Nov Matake' user.picture # => 'https://graph.facebook.com/matake/picture'
user = FbGraph::User.new('matake', :access_token => YOUR_ACCESS_TOKEN) user.identifier # => "matake" user.name # => nil user.link # => nil user.fetch user.name # => "Nov Matake" user.description # => "http://www.facebook.com/matake"
page = FbGraph::Page.fetch('smartfmteam') page.name # => 'smart.fm' page.picture # => 'https://graph.facebook.com/smart.fm/picture'
:
==== Connections
user = FbGraph::User.fetch('matake') user.feed user.posts user.friends user.tagged :
FbGraph::User.new('matake').friends # => raise FbGraph::Unauthorized user = FbGraph::User.fetch('matake', :access_token => ACCESS_TOKEN) user.albums user.events user.friends user.likes :
me = User.new('me', :access_token => ACCESS_TOKEN) me.home :
==== Search
FbGraph::Searchable.search("FbGraph") # => Array of Hash
FbGraph::Page.search("FbGraph") # => Array of FbGraph::Page FbGraph::User.search("matake", :access_token => ACCESS_TOKEN) # => Array of FbGraph::User
==== Pagination
user = FbGraph::User.new('matake', :access_token => ACCESS_TOKEN) likes = user.likes # => Array of FbGraph::Like likes.next # => Array of FbGraph::Like (next page) likes.previous # => Array of FbGraph::Like (previous page) likes.collection.next # => Hash for pagination options (ex. {"limit"=>"25", "until"=>"2010-08-08T03:17:21+0000"}) likes.collection.previous # => Hash for pagination options (ex. {"limit"=>"25", "since"=>"2010-08-08T06:28:20+0000"}) user.likes(likes.collection.next) # => same with likes.next user.likes(likes.collection.previous) # => same with likes.previous
results = FbGraph::Page.search("FbGraph") # => Array of FbGraph::Page results.next # => Array of FbGraph::Page (next page) results.previous # => Array of FbGraph::Page (next page) results.klass # => FbGraph::Page results.collection.next # => Hash for pagination options (ex. {"limit"=>"25", "until"=>"2010-08-08T03:17:21+0000"}) results.collection.previous # => Hash for pagination options (ex. {"limit"=>"25", "since"=>"2010-08-08T06:28:20+0000"}) results.klass.search(results.query, results.collection.next) # => same with results.next results.klass.search(results.query, results.collection.previous) # => same with results.previous
=== POST
==== Update status (wall post)
me = FbGraph::User.me(ACCESS_TOKEN) me.feed!( :message => 'Updating via FbGraph', :picture => 'https://graph.facebook.com/matake/picture', :link => 'http://github.com/nov/fb_graph', :name => 'FbGraph', :description => 'A Ruby wrapper for Facebook Graph API' )
==== Post a like/comment to a post
post = FbGraph::Page.new(117513961602338).feed.first bool = post.like!( :access_token => ACCESS_TOKEN ) comment = post.comment!( :access_token => ACCESS_TOKEN, :message => 'Hey, I'm testing you!' )
==== Post a note
page = FbGraph::Page.new(117513961602338) note = page.note!( :access_token => ACCESS_TOKEN, :subject => 'testing', :message => 'Hey, I'm testing you!' )
==== Post a link
me = FbGraph::User.me(ACCESS_TOKEN) link = me.link!( :link => 'http://github.com/nov/fb_graph', :message => 'A Ruby wrapper for Facebook Graph API.' )
==== Create Event, respond to it
me = FbGraph::User.me(ACCESS_TOKEN) event = me.event!( :name => 'FbGraph test event', :start_time => 1.week.from_now.to_i, :end_time => 2.week.from_now.to_i ) bool = event.attending!( :access_token => ACCESS_TOKEN ) bool = event.maybe!( :access_token => ACCESS_TOKEN ) bool = event.declined!( :access_token => ACCESS_TOKEN )
==== Create an album
me = FbGraph::User.me(ACCESS_TOKEN) album = me.album!( :name => 'FbGraph test', :message => 'test test test' ) # => now facebook Graph API returns weird response for this call
==== Upload a photo to an album
me = FbGraph::User.me(ACCESS_TOKEN) album = me.albums.first album.photo!( :access_token => ACCESS_TOKEN, :image => File.new('/Users/nov/Desktop/nov.gif', 'rb'), # 'rb' is needed only on windows :message => 'Hello, where is photo?' )
=== DELETE
==== Delete an object
post = FbGraph::Page.new(117513961602338).feed.first bool = post.like!( :access_token => ACCESS_TOKEN ) comment = post.comment!( :access_token => ACCESS_TOKEN, :message => 'Hey, I'm testing you!' ) comment.destroy(:access_token => ACCESS_TOKEN) post.unlike!(:access_token => ACCESS_TOKEN) post.destroy(:access_token => ACCESS_TOKEN)
=== Authentication
Both Facebook JavaScript SDK and normal OAuth2 flow is supported. Below I show simple sample code. You can also see http://github.com/nov/fb_graph_sample for more details Rails3 sample application.
==== JavaScript SDK
fb_auth = FbGraph::Auth.new(YOUR_APP_ID, YOUR_APPLICATION_SECRET) fb_auth.client # => OAuth2::Client
fb_auth.from_cookie(cookies) fb_auth.access_token # => OAuth2::AccessToken fb_auth.user # => FbGraph::User (only basic attributes) fb_auth.user.fetch # => fetch more details
==== Normal OAuth2 Flow
redirect_to fb_auth.client.web_server.authorize_url( :redirect_uri => "http://your.client.com/facebook/callback", :scope => "email,read_stream,offline_access" )
fb_auth.client.web_server.get_access_token( params[:code], :redirect_uri => callback_facebook_url ) # => OAuth2::AccessToken FbGraph::User.me(access_token).fetch # => fetch user
=== Analytics
app = FbGraph::Application.new(YOUR_APP_ID, :secret => YOUR_APPLICATION_SECRET) app.insights # => Array of FbGraph::Insight
== Documents
Currently fb_graph isn't so well documented.
I'm continuously updating RDoc now, but writing all documents in English is heavy task for me.
Please see RDoc.info (http://rdoc.info/github/nov/fb_graph), and if the document is missing or hard to understand, please contact me on github.
I'll add more documents or sample code.
=== Objects
=== Connections
== Note on Patches/Pull Requests
== Copyright
Copyright (c) 2010 nov matake. See LICENSE for details.
FAQs
Unknown package
We found that palidanx-fb_graph demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.