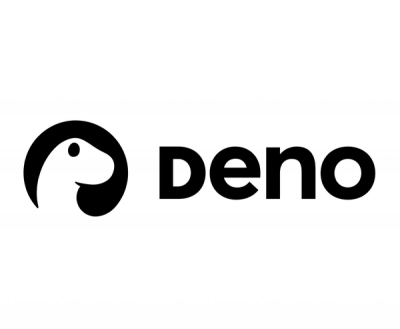
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
SlackChatter is a simple-to-use Slack API wrapper for ruby which makes it quick and easy to constantly annoy your friends, co-workers, and loved ones with waaayyyyy to many process status updates. It provides easy-access to all of the functionallity supported by the Slack Api with the following exceptions:
Release Notes:
Add this line to your application's Gemfile:
gem 'slack_chatter'
And then execute:
$ bundle
Or install it yourself as:
$ gem install slack_chatter
To use this client you will need a slack api access token which can be found here
client = SlackChatter::Client.new("slack-api-token")
opts = {:icon_url => "http://www.example.com/slack-bot-profile-picture.jpg"}
client = SlackChatter::Client.new("slack-api-token", opts)
opts = {:username => "slack_chatter"}
client = SlackChatter::Client.new("slack-api-token", opts)
client.api.test
=> #<SlackChatter::Response ok=true, args={"token"=>"xoxp-4114452838-4114452844-6031365557-2cc510"}, response=#<HTTParty::Response:0x7fed152c6c08 ...>, code=200>
client.auth.test
=> #<SlackChatter::Response ok=true, args={"token"=>"xoxp-4114452838-4114452844-6031365557-2cc510"}, response=#<HTTParty::Response:0x7fed152c6c08 ...>, code=200>
The api methods follow the following format
client.method_namespace.action(required, arguments, {optional: arguments})
# Example:
client.chat.post_message("some-channel-id", "some message to post", {optional_param: 'and its value'})
These methods directly correspond to those found in the Slack API Docs
NOTE: Not all methods accept an options hash. However, all methods with optional parameters do accept one.
response = client.channels.list
=> #<SlackChatter::Response ok=true, response=#<HTTPARTY::RESPONSE:0x7fed1528eba0 ...>, channels=[...], code=200>
response.success?
=> true
response.code
=> 200
response.channels
=> channels [
{
"id"=>"C043CDARC",
"name"=>"general",
"is_channel"=>true,
"created"=>1426800248,
"creator"=>"U043CDAQU",
"is_archived"=>false,
"is_general"=>true,
"is_member"=>true,
"members"=>["U043CDAQU"],
"topic"=>{
"value"=>"",
"creator"=>"",
"last_set"=>0
},
"purpose"=>{
"value"=>"This channel is for team-wide communication and announcements. All team members are in this channel.",
"creator"=>"",
"last_set"=>0
},
"num_members"=>1
},
{...},
...
]
response = client.channels.find_by_name("wiggle-room")
=> {
"id"=>"C06817EC8",
"name"=>"wiggle-room",
"is_channel"=>true,
"created"=>1434045083,
"creator"=>"U043CDAQU",
"is_archived"=>false,
"is_general"=>false,
"is_member"=>true,
"members"=>["U043CDAQU"],
"topic"=>{
"value"=>"",
"creator"=>"",
"last_set"=>0
},
"purpose"=>{
"value"=>"",
"creator"=>"",
"last_set"=>0
},
"num_members"=>1
}
channel_id = response["id"]
=> "C06817EC8"
response = client.channels.create("new channel name")
=> #<SlackChatter::Response ok=true, response=#<HTTPARTY::RESPONSE:0x7fed1528eba0 ...>, channel={"id"=>"C0680SVU2", ...}, code=200>
channel_id = response.channel["id"]
=> "C0680SVU2"
response = client.channels.join("wiggle-room")
=> #<SlackChatter::Response ok=true, response=#<HTTPARTY::RESPONSE:0x7fed1528eba0 ...>, channel={"id"=>"C06817EC8", "name"=>"wiggle-room", "is_channel"=>true, "created"=>1434045083, "creator"=>"U043CDAQU", "is_archived"=>false, "is_general"=>false, "is_member"=>true, "last_read"=>"1434045087.000003", "latest"=>{"user"=>"U043CDAQU", "type"=>"message", "subtype"=>"channel_leave", "text"=>"<@U043CDAQU|sly_fox> has left the channel", "ts"=>"1434045087.000003"}, "unread_count"=>0, "unread_count_display"=>0, "members"=>["U043CDAQU"], "topic"=>{"value"=>"", "creator"=>"", "last_set"=>0}, "purpose"=>{"value"=>"", "creator"=>"", "last_set"=>0}}, code=200>
response = client.channels.history(channel_id)
=> #<SlackChatter::Response ok=true, response=#<HTTPARTY::RESPONSE:0x7fed1528eba0 ...>, latest="1434027718", messages=[...], has_more=false, code=200, success?=true>
Options:
Note: For all timestamps you must use epoch time, so for any Date, Time, or DateTime object you want to use as an argument use #to_i on it
(Time.now() - 5.hours).to_i
=> 1434027718 # epoch
response = client.channels.invite(channel_id, user_id)
response = client.channels.kick(channel_id, user_id)
response = client.channels.leave(channel_id)
Used for tracking read position
response = client.channels.mark(channel_id)
response = client.channels.rename(channel_id, "new_name")
response = client.channels.leave(channel_id, "Dogs acting like cats")
response = client.channels.leave(channel_id, "Post adorable videos")
response = client.channels.archive(channel_id)
=> #<SlackChatter::Response ok=true, response=#<HTTPARTY::RESPONSE:0x7fed1528eba0 ...>, code=200>
response = client.channels.unarchive(channel_id)
=> #<SlackChatter::Response ok=true, response=#<HTTPARTY::RESPONSE:0x7fed1528eba0 ...>, code=200>
response = client.chat.post_message(channel_id, "I am a robot: beep boop boop beep")
=> #<SlackChatter::Response ok=true, response=#<HTTPARTY::RESPONSE:0x7fed1528eba0 ...>, channel="C0680SVU2", ts="1434044883.000003", message={"text"=>"I am a robot: beep boop boop beep", "username"=>"bot", "type"=>"message", "subtype"=>"bot_message", "ts"=>"1434044883.000003"}, code=200>
message = response.message
=> {
"text"=>"I am a robot: beep boop boop beep",
"username"=>"bot",
"type"=>"message",
"subtype"=>"bot_message",
"ts"=>"1434044883.000003"
}
response = client.chat.update(message["ts"], channel_id, "Just kidding, not a robot")
=> #<SlackChatter::Response ok=true, response=#<HTTPARTY::RESPONSE:0x7fed1528eba0 ...>, code=200>
response = client.chat.delete(message["ts"], channel_id)
=> #<SlackChatter::Response ok=true, response=#<HTTPARTY::RESPONSE:0x7fed1528eba0 ...>, code=200>
Groups has the same methods that Channels has, with the exception that you cannot join a group (you must be invited) and it has the following additional methods:
client.groups.open(group_id)
=> #<SlackChatter::Response ok=true, response=#<HTTPARTY::RESPONSE:0x7fed1528eba0 ...>, code=200>
client.groups.close(group_id)
=> #<SlackChatter::Response ok=true, response=#<HTTPARTY::RESPONSE:0x7fed1528eba0 ...>, code=200>
This method takes an existing private group and performs the following steps:
The new group will have a special parent_group property pointing to the original archived group. This will only be returned for members of both groups, so will not be visible to any newly invited members.
client.groups.create_child(group_id)
=> #<SlackChatter::Response ok=true, response=#<HTTPARTY::RESPONSE:0x7fed1528eba0 ...>, code=200>
This method opens a direct message channel with another member of your Slack team.
client.im.open(user_id)
=> #<SlackChatter::Response ok=true, response=#<HTTPARTY::RESPONSE:0x7fed1528eba0 ...>, code=200>
response = client.im.history(user_id, opts_hash)
=> #<SlackChatter::Response ok=true, response=#<HTTPARTY::RESPONSE:0x7fed1528eba0 ...>, latest="1434027718", messages=[...], has_more=false, code=200>
Options:
Note: For all timestamps you must use epoch time, so for any Date, Time, or DateTime object you want to use as an argument use #to_i on it
(Time.now() - 5.hours).to_i
=> 1434027718 # epoch
response = client.im.list
=> #<SlackChatter::Response ok=true, response=#<HTTPARTY::RESPONSE:0x7fed1528eba0 ...>, latest="1434027718", channels=[...], has_more=false, code=200>
This method closes a direct message channel with another member of your Slack team.
client.im.close(user_id)
=> #<SlackChatter::Response ok=true, response=#<HTTPARTY::RESPONSE:0x7fed1528eba0 ...>, code=200>
Used for tracking read position
response = client.im.mark(channel_id)
response = client.files.delete(file_id)
response = client.files.info(file_id, options)
Options:
The file object contains information about the uploaded file.
Each comment object in the comments array contains details about a single comment. Comments are returned oldest first.
The paging information contains the count of comments returned, the total number of comments, the page of results returned in this response and the total number of pages available.
response = client.files.list(options)
Options:
response = client.files.upload({file: "../robot.png"})
=> #<SlackChatter::Response ok=true, file={
"id"=>"F068PHD0R",
"created"=>1434080019,
"timestamp"=>1434080019,
"name"=>"robot.png",
"title"=>"robot",
"mimetype"=>"image/png",
"filetype"=>"png",
"pretty_type"=>"PNG",
"user"=>"U043CDAQU",
"editable"=>false,
"size"=>3446,
"mode"=>"hosted",
"is_external"=>false,
"external_type"=>"",
"is_public"=>false,
"public_url_shared"=>false,
"url"=>"https://slack-files.com/files-pub/T043CDAQN-F068PHD0R-5ef86791d5/robot.png",
"url_download"=>"https://slack-files.com/files-pub/T043CDAQN-F068PHD0R-5ef86791d5/download/robot.png",
"url_private"=>"https://files.slack.com/files-pri/T043CDAQN-F068PHD0R/robot.png",
"url_private_download"=>"https://files.slack.com/files-pri/T043CDAQN-F068PHD0R/download/my_heart.png", "thumb_64"=>"https://slack-files.com/files-tmb/T043CDAQN-F068PHD0R-e1ebed375a/my_heart_64.png",
"thumb_80"=>"https://slack-files.com/files-tmb/T043CDAQN-F068PHD0R-e1ebed375a/my_heart_80.png",
"thumb_360"=>"https://slack-files.com/files-tmb/T043CDAQN-F068PHD0R-e1ebed375a/my_heart_360.png",
"thumb_360_w"=>227,
"thumb_360_h"=>185,
"thumb_160"=>"https://slack-files.com/files-tmb/T043CDAQN-F068PHD0R-e1ebed375a/my_heart_160.png",
"image_exif_rotation"=>1,
"permalink"=>"https://myteam.slack.com/files/sly_fox/F068PHD0R/my_heart.png",
"permalink_public"=>"https://slack-files.com/T043CDAQN-F068PHD0R-5ef86791d5",
"channels"=>[],
"groups"=>[],
"ims"=>[],
"comments_count"=>0
}, response=#<HTTParty::Response:0x1016d4a50 ...}>, code=200>
response = client.search.all("robot")
=> #<SlackChatter::Response ok=true, query="robot", messages={...}, "files"=>{...}}, response=#<HTTParty::Response:0x7fed152b9148 ...>, code=200>
response["messages"]
=> {
"total"=>1,
"pagination"=>{
"total_count"=>1,
"page"=>1,
"per_page"=>20,
"page_count"=>1,
"first"=>1,
"last"=>1
},
"paging"=>{
"count"=>20,
"total"=>1,
"page"=>1,
"pages"=>1
},
"matches"=>[
{
"type"=>"message",
"user"=>"",
"username"=>"bot",
"ts"=>"1434044883.000003",
"text"=>"I am a robot: beep boop boop beep", "channel"=>{
"id"=>"C0680SVU2",
"name"=>"new-channel-name"
},
"permalink"=>"https://myteam.slack.com/archives/new-channel-name/p1434044883000003"
},
{...},
...
]
}
Options:
response = client.search.files("robot")
=> #<SlackChatter::Response ok=true, query="robot", messages={...}, "files"=>{...}}, response=#<HTTParty::Response:0x7fed152b9148 ...>, code=200>
Options:
response = client.search.messages("robot")
=> #<SlackChatter::Response ok=true, query="robot", messages={...}, "files"=>{...}}, response=#<HTTParty::Response:0x7fed152b9148 ...>, code=200>
Options:
response = client.stars.list(options)
Options:
response = client.team.access_logs(options)
Options:
response = client.team.info
response = client.users.get_presence(user_id)
response = client.users.info(user_id)
response = client.users.list
response = client.users.set_active
response = client.users.set_presence("away") # Either "auto" or "away"
response = client.emoji.list
This method allows you to exchange a temporary OAuth code for an API access token. This is used as part of the OAuth authentication flow.
respone = client.oauth.access(client_id, client_secret, code, options)
Options:
git checkout -b my-new-feature
)git commit -am 'Add some feature'
)git push origin my-new-feature
)FAQs
Unknown package
We found that slack_chatter demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.