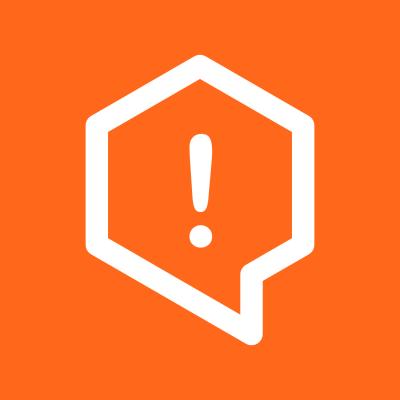
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Title Estuary is a gem/plugin that makes it easy to set page titles. It it built with internationalization in mind. It requires ActiveSupport's I18N, but should be usable with frameworks other than Rails.
=== How-To
==== 1: tell Rails about the gem:
config.gem 'title_estuary', :source => 'http://gemcutter.org'
==== 2: have Rails install the gem:
[sudo] rake gems:install
==== 3: set up your layout:
==== 4. customize your titles:
Use the format "page.title.controller_name.action_name"
en: page: title: accounts: new: "Sign Up" sessions: new: "Sign In" projects: index: "Your projects" new: "Start a new project"
If you want to use any interpolated strings, make sure you define an #interpolation_options method in your controller:
en: page: title: projects: index: "All {{number_of_projects}} of your projects" show: "Project {{project_name}}" edit: "Edit project {{project_name}}"
class ProjectsController < ActionController::Base # An example definition of #interpolation_options # that sets certain project-related values as applicable. # # @return [Hash] a hash of interpolation values def interpolation_options returning({}) do |result| result[:number_of_projects] = @projects.size if @projects.present? result[:project_name] = @project.name if @project.present? result[:project_id] = params[:id] end end hide_action :interpolation_options end
If you need more dynamic titles than simple interpolation affords, set the title manually in an action:
class ProjectsController < ActionController::Base def show @project = Project.find(params[:id]) self.page_title = "A title based on #{@project.some_complex_string}" end end
or in a filter:
class ProjectsController < ActionController::Base before_filter :set_title private def set_title self.page_title = "Some complex title" end end
or from a view:
<% content_for :page_title do %> Some complex page title <% end %>
=== Integration with Inherited Resources
When included in a controller class that inherits from InheritedResources::Base, Title Estuary uses :resource_class when building default page names.
@see TitleEstuary::InheritedResourcesSupport @see http://github.com/josevalim/inherites_resources
=== Integration with High Voltage
When included in a controller class that inherits from HighVoltage::PagesController, Title Estuary uses the page title as the default title. It also changes the i18n key scheme to "page.title..<page_name>" to let you define different titles for each page. For example:
en: page: title: pages: faq: "Frequently Asked Questions"
Nota Bene: if you include +TitleEstuary+ in your +ApplicationController+, you'll need to re-include it in the +PagesController+. If you're using High Voltage's default controller, you can do so in an initializer:
HighVoltage::PagesController.class_eval do include TitleEstuary end
If, on the other hand, you're defining your own +PagesController+, you can include it there:
class PagesController < HighVoltage::PagesController include TitleEstuary end
FAQs
Unknown package
We found that title_estuary demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.