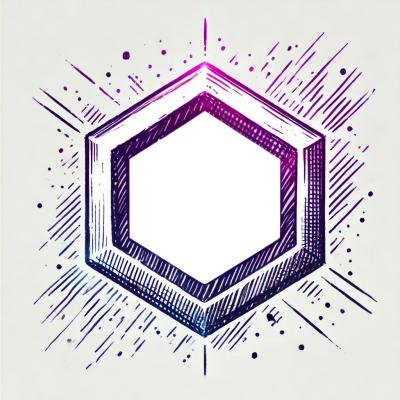
Security News
Maven Central Adds Sigstore Signature Validation
Maven Central now validates Sigstore signatures, making it easier for developers to verify the provenance of Java packages.
github.com/Lyearn/mgod
mgod
is a MongoDB ODM specifically designed for Go. It provides a structured way to map Go models to MongoDB collections, simplifying database interactions in Go applications.
go get github.com/Lyearn/mgod
Use existing MongoDB connection, or setup a new one to register a default database connection.
For existing database connection,
import "github.com/Lyearn/mgod"
func init() {
// dbConn is the database connection obtained using Go Mongo Driver's Connect method.
mgod.SetDefaultConnection(dbConn)
}
To setup a new connection,
import (
"time"
"github.com/Lyearn/mgod"
"go.mongodb.org/mongo-driver/mongo/options"
)
func init() {
// `cfg` is optional. Can rely on default configurations by providing `nil` value in argument.
cfg := &mgod.ConnectionConfig{Timeout: 5 * time.Second}
dbName := "mgod-test"
opts := options.Client().ApplyURI("mongodb://root:mgod123@localhost:27017")
err := mgod.ConfigureDefaultConnection(cfg, dbName, opts)
}
Add tags (wherever applicable) in existing struct (or define a new model).
type User struct {
Name string
EmailID string `bson:"emailId"`
Age *int32 `bson:",omitempty"`
JoinedOn string `bson:"joinedOn" mgoType:"date"`
}
Use mgod
to get the entity ODM.
import (
"github.com/Lyearn/mgod"
"github.com/Lyearn/mgod/schema/schemaopt"
)
model := User{}
schemaOpts := schemaopt.SchemaOptions{
Collection: "users",
Timestamps: true,
}
userModel, _ := mgod.NewEntityMongoModel(model, schemaOpts)
Use the entity ODM to perform CRUD operations with ease.
Insert a new document.
joinedOn, _ := dateformatter.New(time.Now()).GetISOString()
userDoc := User{
Name: "Gopher",
EmailID: "gopher@mgod.com",
JoinedOn: joinedOn,
}
user, _ := userModel.InsertOne(context.TODO(), userDoc)
Output:
{
"_id": ObjectId("65697705d4cbed00e8aba717"),
"name": "Gopher",
"emailId": "gopher@mgod.com",
"joinedOn": ISODate("2023-12-01T11:32:19.290Z"),
"createdAt": ISODate("2023-12-01T11:32:19.290Z"),
"updatedAt": ISODate("2023-12-01T11:32:19.290Z"),
"__v": 0
}
Find documents using model properties.
users, _ := userModel.Find(context.TODO(), bson.M{"name": userDoc.Name})
Output:
[]User{
User{
Name: "Gopher",
EmailID: "gopher@mgod.com",
JoinedOn: "2023-12-01T11:32:19.290Z",
}
}
Creating mgod
was driven by the need to simplify MongoDB interactions while keeping one schema for all in Go. Traditionally, working with MongoDB in Go involved either using separate structs for database and service logic or manually converting service structs to MongoDB documents, a process that was both time-consuming and error-prone. This lack of integration often led to redundant coding, especially when dealing with union types or adding meta fields for each MongoDB operation.
Inspired by the easy interface of MongoDB handling using Mongoose and Typegoose libraries available in Node, mgod
aims to streamline these processes. It offers a more integrated approach, reducing the need to duplicate code and enhancing type safety, making MongoDB operations more intuitive and efficient in Go.
The current version of mgod is a stable release. However, there are plans to add a lot more features like -
If you have any interesting feature requests, feel free to open an issue on GitHub issue tracker. We will be more than happy to discuss that!
For user facing documentation, check out docs.
For contribution guidelines, check out CONTRIBUTING.
mgod
is licensed under the MIT License.
FAQs
Unknown package
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Maven Central now validates Sigstore signatures, making it easier for developers to verify the provenance of Java packages.
Security News
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
Research
Security News
Socket researchers uncovered a backdoored typosquat of BoltDB in the Go ecosystem, exploiting Go Module Proxy caching to persist undetected for years.