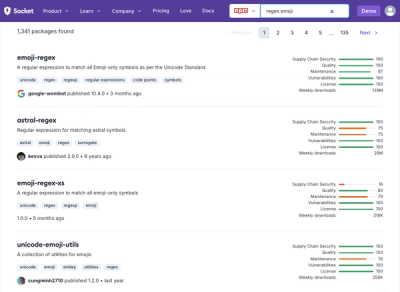
Security News
Weekly Downloads Now Available in npm Package Search Results
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
github.com/kohestanimahdi/gopagination
This is a package for pagination and filtering all slice and arrays of struct
Package gopagination is a package that you can filter your slice and Array of any struct. This can help you to filter and pagin any data, for example you can use this package to fliter or pagination datas in web api. Clinet send us what data needs and this package send response that client expected.
For install this package you should execute this
go get github.com/kohestanimahdi/go-pagination-filtering
The main struct of pagination, this has six property :
type PaginationConfig struct {
SortColumn string // The column name of struct that we want to sort by them
IsAscending bool // Ascending sort or Descending ?
Take int // The count of data that we need
Skip int // The count of data that we want to skip
AndLogic bool // And(&) between ExpressionFilters ?
ExpressionFilters []ExpressionFilter // Filters - If we need filters
}
The struct of Filters, this has three property :
type ExpressionFilter struct {
PropertyName string // The column name of struct that we want to filter on this
Value interface{} // Value of that column name
Comparison int // Comparison
}
Comparison is one of :
0 for Equal (=) // This use for numeric,time and string fields
1 for LessThan (<) // This use for numeric and time fields
2 for LessThanOrEqual (<=) // This use for numeric and time fields
3 for GreaterThan (>) // This use for numeric and time fields
4 for GreaterThanOrEqual (>=) // This use for numeric and time fields
5 for NotEqual (!=) // This use for numeric,time and string fields
6 for Contains // This use for string fields
7 for StartsWith // This use for string fields
8 for EndsWith // This use for string fields
Imagine we have a struct named Person
like this :
type Person struct {
Name string
Average float32
Birthday time.Time
}
And a slice of Persons name 'persons' like :
var persons []Person
p1 := Person{
Name: "Mahdi Kohestani",
Average: 19.5,
Birthday: time.Date(1997, time.December, 25, 0, 0, 0, 0, time.UTC),
}
p2 := Person{
Name: "Mahdi Malverdi",
Average: 18,
Birthday: time.Date(1997, time.November, 3, 0, 0, 0, 0, time.UTC),
}
p3 := Person{
Name: "Amir Sartipi",
Average: 19,
Birthday: time.Date(1997, time.September, 28, 0, 0, 0, 0, time.UTC),
}
p4 := Person{
Name: "Mahdi Rangraz",
Average: 12,
Birthday: time.Date(1998, time.February, 10, 0, 0, 0, 0, time.UTC),
}
p5 := Person{
Name: "Mohammad Saleh",
Average: 13,
Birthday: time.Date(1998, time.June, 16, 0, 0, 0, 0, time.UTC),
}
persons = append(persons, p1, p2, p3, p4, p5)
If we want all datas and sort ascending by Name
column
pg := pagination.PaginationConfig{
SortColumn: "Name", //Sort by `Name` column
IsAscending: true, // Ascending
Take: 0, // Get all datas
Skip: 0, // skip zero
AndLogic: true, // And between filters
ExpressionFilters: nil, // We do not need filter
}
newdatas,err :=pg.DoPagination(persons)
if err!= nil{
panic(err)
}
fmt.Println(newdatas)
If we want all datas that sort ascending by Name
column and Average
grather than 15
pg := pagination.PaginationConfig{
SortColumn: "Name", //Sort by `Name` column
IsAscending: true, // Ascending
Take: 0, // Get all datas
Skip: 0, // skip zero
AndLogic: true, // And between filters
ExpressionFilters: make([]pagination.ExpressionFilter, 0),
}
pg.ExpressionFilters = append(pg.ExpressionFilters, pagination.ExpressionFilter{
PropertyName: "Average",
Value: 15.0,
Comparison: 3,
})
newdatas,err :=pg.DoPagination(persons)
if err!= nil{
panic(err)
}
fmt.Println(newdatas)
If we want datas that sort ascending by Name
column and Average
grather than 15 or Name
contains 'Mahdi'
pg := pagination.PaginationConfig{
SortColumn: "Name", //Sort by `Name` column
IsAscending: true, // Ascending
Take: 0, // Get all datas
Skip: 0, // skip zero
AndLogic: false, // Or between filters
ExpressionFilters: make([]pagination.ExpressionFilter, 0),
}
pg.ExpressionFilters = append(pg.ExpressionFilters, pagination.ExpressionFilter{
PropertyName: "Average",
Value: 15.0,
Comparison: 3,
})
pg.ExpressionFilters = append(pg.ExpressionFilters, pagination.ExpressionFilter{
PropertyName: "Name",
Value: "Mahdi",
Comparison: 6,
})
newdatas,err :=pg.DoPagination(persons)
if err!= nil{
panic(err)
}
fmt.Println(newdatas)
If we want only 2 data that sort ascending by Name
column and Average
grather than 15 or Name
contains 'Mahdi'
pg := pagination.PaginationConfig{
SortColumn: "Name", //Sort by `Name` column
IsAscending: true, // Ascending
Take: 2, // Get 2 data
Skip: 0, // skip zero
AndLogic: false, // Or between filters
ExpressionFilters: make([]pagination.ExpressionFilter, 0),
}
pg.ExpressionFilters = append(pg.ExpressionFilters, pagination.ExpressionFilter{
PropertyName: "Average",
Value: 15.0,
Comparison: 3,
})
pg.ExpressionFilters = append(pg.ExpressionFilters, pagination.ExpressionFilter{
PropertyName: "Name",
Value: "Mahdi",
Comparison: 6,
})
newdatas,err :=pg.DoPagination(persons)
if err!= nil{
panic(err)
}
fmt.Println(newdatas)
If we want only second and third data (skip 1) that sort ascending by Name
column and Average
grather than 15 or Name
contains 'Mahdi'
pg := pagination.PaginationConfig{
SortColumn: "Name", //Sort by `Name` column
IsAscending: true, // Ascending
Take: 2, // Get 2 data
Skip: 1, // skip zero
AndLogic: false, // Or between filters
ExpressionFilters: make([]pagination.ExpressionFilter, 0),
}
pg.ExpressionFilters = append(pg.ExpressionFilters, pagination.ExpressionFilter{
PropertyName: "Average",
Value: 15.0,
Comparison: 3,
})
pg.ExpressionFilters = append(pg.ExpressionFilters, pagination.ExpressionFilter{
PropertyName: "Name",
Value: "Mahdi",
Comparison: 6,
})
newdatas,err :=pg.DoPagination(persons)
if err!= nil{
panic(err)
}
fmt.Println(newdatas)
FAQs
Unknown package
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
Security News
A Stanford study reveals 9.5% of engineers contribute almost nothing, costing tech $90B annually, with remote work fueling the rise of "ghost engineers."
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.