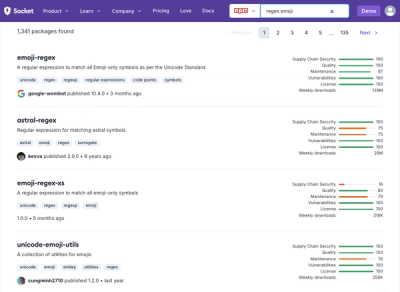
Security News
Weekly Downloads Now Available in npm Package Search Results
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
github.com/tiagomelo/go-saga
A tiny Go package that provides an implementation of the Saga pattern for managing distributed transactions.
The Saga pattern ensures that either all steps of a transaction succeed or all are compensated (rolled back) in case of failure, providing a way to maintain consistency across distributed systems.
WithStateManager
sets a custom state managergo get github.com/tiagomelo/go-saga
package main
import (
"context"
"fmt"
"github.com/tiagomelo/go-saga"
)
func main() {
// Create a new Saga instance with the default in-memory state manager.
s := saga.New()
// Add steps to the Saga.
s.AddStep(saga.NewStep("step1",
func(ctx context.Context) error {
fmt.Println("Executing Step 1")
return nil
},
func(ctx context.Context) error {
fmt.Println("Compensating Step 1")
return nil
},
))
s.AddStep(saga.NewStep("step2",
func(ctx context.Context) error {
fmt.Println("Executing Step 2")
return fmt.Errorf("Step 2 failed")
},
func(ctx context.Context) error {
fmt.Println("Compensating Step 2")
return nil
},
))
// Execute the Saga.
if err := s.Execute(context.Background()); err != nil {
fmt.Printf("Saga failed: %v\n", err)
} else {
fmt.Println("Saga completed successfully")
}
}
package main
import (
"context"
"fmt"
"github.com/yourusername/go-saga"
)
// CustomStateManager is an example implementation of the StateManager interface.
// Replace this with your actual custom state manager implementation.
type CustomStateManager struct {
// Implement the necessary fields and methods for your custom state management.
}
func (c *CustomStateManager) SetStepState(stepIndex int, success bool) error {
// Implement logic to store the state of each step.
return nil
}
func (c *CustomStateManager) StepState(stepIndex int) (bool, error) {
// Implement logic to retrieve the state of each step.
return false, nil
}
func main() {
// Create a custom state manager.
stateManager := &CustomStateManager{}
// Create a new Saga instance with the custom state manager.
s := saga.New(saga.WithStateManager(stateManager))
// Add steps to the Saga.
s.AddStep(saga.NewStep("step1",
func(ctx context.Context) error {
fmt.Println("Executing Step 1")
return nil
},
func(ctx context.Context) error {
fmt.Println("Compensating Step 1")
return nil
},
))
s.AddStep(saga.NewStep("step2",
func(ctx context.Context) error {
fmt.Println("Executing Step 2")
return fmt.Errorf("Step 2 failed")
},
func(ctx context.Context) error {
fmt.Println("Compensating Step 2")
return nil
},
))
// Execute the Saga.
if err := s.Execute(context.Background()); err != nil {
fmt.Printf("Saga failed: %v\n", err)
} else {
fmt.Println("Saga completed successfully")
}
}
make test
make coverage
Contributions are welcome! If you find any issues or have suggestions for improvements, please open an issue or submit a pull request.
This project is licensed under the MIT License. See the LICENSE file for more details.
FAQs
Unknown package
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
Security News
A Stanford study reveals 9.5% of engineers contribute almost nothing, costing tech $90B annually, with remote work fueling the rise of "ghost engineers."
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.