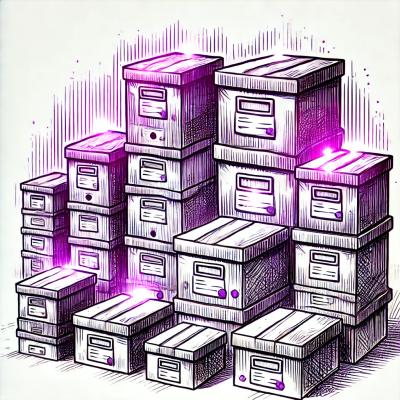
Security News
PyPI’s New Archival Feature Closes a Major Security Gap
PyPI now allows maintainers to archive projects, improving security and helping users make informed decisions about their dependencies.
github.com/yaotian/go-sitemap
package main
import (
"github.com/yaotian/go-sitemap/stm"
)
func main() {
sm := stm.NewSitemap()
// Create method must be that calls first this method in that before
// call to Add method on this struct.
sm.Create()
//this is to support baidu
sm.SetSearchEngine(stm.SearcnEngine_Baidu)
mobileType := stm.NewMobleType("pc,mobile")
sm.Add(stm.URL{"loc": "home", "changefreq": "always", "mobile": mobileType})
sm.Add(stm.URL{"loc": "readme"})
sm.Add(stm.URL{"loc": "aboutme", "priority": 0.1})
sm.Finalize().PingSearchEngines()
}
Sitemap provides interface for create sitemap xml file and that has convenient interface. And also needs to use first Sitemap struct if it wants to use this package.
$ go get github.com/yaotian/go-sitemap/stm
Current Features or To-Do
To disable all non-essential output you can give false
to sm.SetVerbose
.
To disable output in-code use the following:
sm := stm.NewSitemap()
sm.SetVerbose(false)
PingSearchEngines requests some ping server.
sm.Finalize().PingSearchEngines()
If you want to add new search engine
, you can set that to method's arguments. like this.
sm.Finalize().PingSearchEngines("http://newengine.com/ping?url=%s")
// Your website's host name
sm.SetDefaultHost("http://www.example.com")
// The remote host where your sitemaps will be hosted
sm.SetSitemapsHost("http://s3.amazonaws.com/sitemap-generator/")
// The directory to write sitemaps to locally
sm.SetPublicPath("tmp/")
// Set this to a directory/path if you don't want to upload to the root of your `SitemapsHost`
sm.SetSitemapsPath("sitemaps/")
// Struct of `S3Adapter`
sm.SetAdapter(&stm.S3Adapter{Region: "ap-northeast-1", Bucket: "your-bucket", ACL: "public-read"})
// It changes to output filename
sm.SetFilename("new_filename")
package main
import (
"github.com/aws/aws-sdk-go/aws/credentials"
"github.com/yaotian/go-sitemap/stm"
)
func main() {
sm := stm.NewSitemap()
sm.SetDefaultHost("http://example.com")
sm.SetSitemapsPath("sitemap-generator") // default: public
sm.SetSitemapsHost("http://s3.amazonaws.com/sitemap-generator/")
sm.SetAdapter(&stm.S3Adapter{
Region: "ap-northeast-1",
Bucket: "your-bucket",
ACL: "public-read",
Creds: credentials.NewEnvCredentials(),
})
sm.Create()
sm.Add(stm.URL{"loc": "home", "changefreq": "always", "mobile": true})
sm.Add(stm.URL{"loc": "readme"})
sm.Add(stm.URL{"loc": "aboutme", "priority": 0.1})
sm.Finalize().PingSearchEngines()
}
sm.Add(stm.URL{"loc": "/news", "news": stm.URL{
"publication": stm.URL{
"name": "Example",
"language": "en",
},
"title": "My Article",
"keywords": "my article, articles about myself",
"stock_tickers": "SAO:PETR3",
"publication_date": "2011-08-22",
"access": "Subscription",
"genres": "PressRelease",
}})
Look at Creating a Google News Sitemap as required.
sm.Add(stm.URL{"loc": "/videos", "video": stm.URL{
"thumbnail_loc": "http://www.example.com/video1_thumbnail.png",
"title": "Title",
"description": "Description",
"content_loc": "http://www.example.com/cool_video.mpg",
"category": "Category",
"tag": []string{"one", "two", "three"},
}})
Look at Video sitemaps as required.
sm.Add(stm.URL{"loc": "/images", "image": []stm.URL{
{"loc": "http://www.example.com/image.png", "title": "Image"},
{"loc": "http://www.example.com/image1.png", "title": "Image1"},
}})
Look at Image sitemaps as required.
sm.Add(stm.URL{"loc": "/geos", "geo": stm.URL{
"format": "kml",
}})
Couldn't find Geo sitemaps example. Although its like a below.
<url>
<loc>/geos</loc>
<geo:geo>
<geo:format>kml</geo:format>
</geo:geo>
</url>
sm.Add(stm.URL{"loc": "mobiles", "mobile": true})
Look at Feature phone sitemaps as required.
package main
import (
"github.com/yaotian/go-sitemap/stm"
)
func main() {
sm := stm.NewSitemap()
sm.SetDefaultHost("http://yourhost.com")
sm.SetSitemapsHost("http://s3.amazonaws.com/sitemaps/")
sm.SetSitemapsPath("sitemaps/")
sm.SetFilename("anothername")
sm.SetCompress(true)
sm.SetVerbose(true)
sm.SetAdapter(&stm.S3Adapter{Region: "ap-northeast-1", Bucket: "your-bucket"})
sm.Create()
sm.Add(stm.URL{"loc": "/home", "changefreq": "dayly"})
sm.Add(stm.URL{"loc": "/abouts", "mobile": true})
sm.Add(stm.URL{"loc": "/news", "news": stm.URL{
"publication": stm.URL{
"name": "Example",
"language": "en",
},
"title": "My Article",
"keywords": "my article, articles about myself",
"stock_tickers": "SAO:PETR3",
"publication_date": "2011-08-22",
"access": "Subscription",
"genres": "PressRelease",
}})
sm.Add(stm.URL{"loc": "/images", "image": []stm.URL{
{"loc": "http://www.example.com/image.png", "title": "Image"},
{"loc": "http://www.example.com/image1.png", "title": "Image1"},
}})
sm.Add(stm.URL{"loc": "/videos", "video": stm.URL{
"thumbnail_loc": "http://www.example.com/video1_thumbnail.png",
"title": "Title",
"description": "Description",
"content_loc": "http://www.example.com/cool_video.mpg",
"category": "Category",
"tag": []string{"one", "two", "three"},
}})
sm.Add(stm.URL{"loc": "/geos", "geo": stm.URL{
"format": "kml",
}})
sm.Finalize().PingSearchEngines("http://newengine.com/ping?url=%s")
}
//this is your urls
urls := makeUrl()
//baidu
baidu_sm := stm.NewSitemap()
baidu_sm.SetVerbose(true)
baidu_sm.SetCompress(false)
baidu_sm.SetDefaultHost(setting.AppUrl2)
baidu_sm.SetPublicPath("static/baidu/")
baidu_sm.SetFilename("sitemap")
baidu_sm.Create()
//有一些特别的searchengine
baidu_sm.SetSearchEngine(stm.SearcnEngine_Baidu)
//其它
sm2 := stm.NewSitemap()
sm2.SetVerbose(true)
sm2.SetCompress(false)
sm2.SetDefaultHost(setting.AppUrl2)
sm2.SetPublicPath("static/google/")
sm2.SetFilename("sitemap")
sm2.Create()
for _, url := range urls {
nv := stm.NewMobleType("pc,mobile")
nv2 := stm.NewMobleType("")
url["mobile"] = nv
baidu_sm.Add(url)
url["mobile"] = nv2
sm2.Add(url)
}
baidu_sm.Finalize()
sm2.Finalize()
Prepare testing
$ go get github.com/clbanning/mxj
Do testing
$ go test -v -cover ./...
FAQs
Unknown package
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now allows maintainers to archive projects, improving security and helping users make informed decisions about their dependencies.
Research
Security News
Malicious npm package postcss-optimizer delivers BeaverTail malware, targeting developer systems; similarities to past campaigns suggest a North Korean connection.
Security News
CISA's KEV data is now on GitHub, offering easier access, API integration, commit history tracking, and automated updates for security teams and researchers.